Amazon Connectors
The platform integrates a variety of connectors to interact with Amazon services, including OpenSearch, DynamoDB, Glue Data Catalog, RDS, and S3. These connectors help simplify the integration, processing, and management of data in cloud and hybrid workflows.
1. AWS OpenSearch
Description
The OpenSearch connector enables querying and manipulation of data stored in Amazon OpenSearch Service (formerly Amazon Elasticsearch).
Use Cases
- Text search across large data collections
- Analysis of logs or real-time metrics
Inputs
- OpenSearch URL: URL of the OpenSearch cluster
- Index Name: Name of the index
- Search Input: Search query
- Ingest Data: Data to ingest (optional)
- Embedding: Embedding data for vector search (optional)
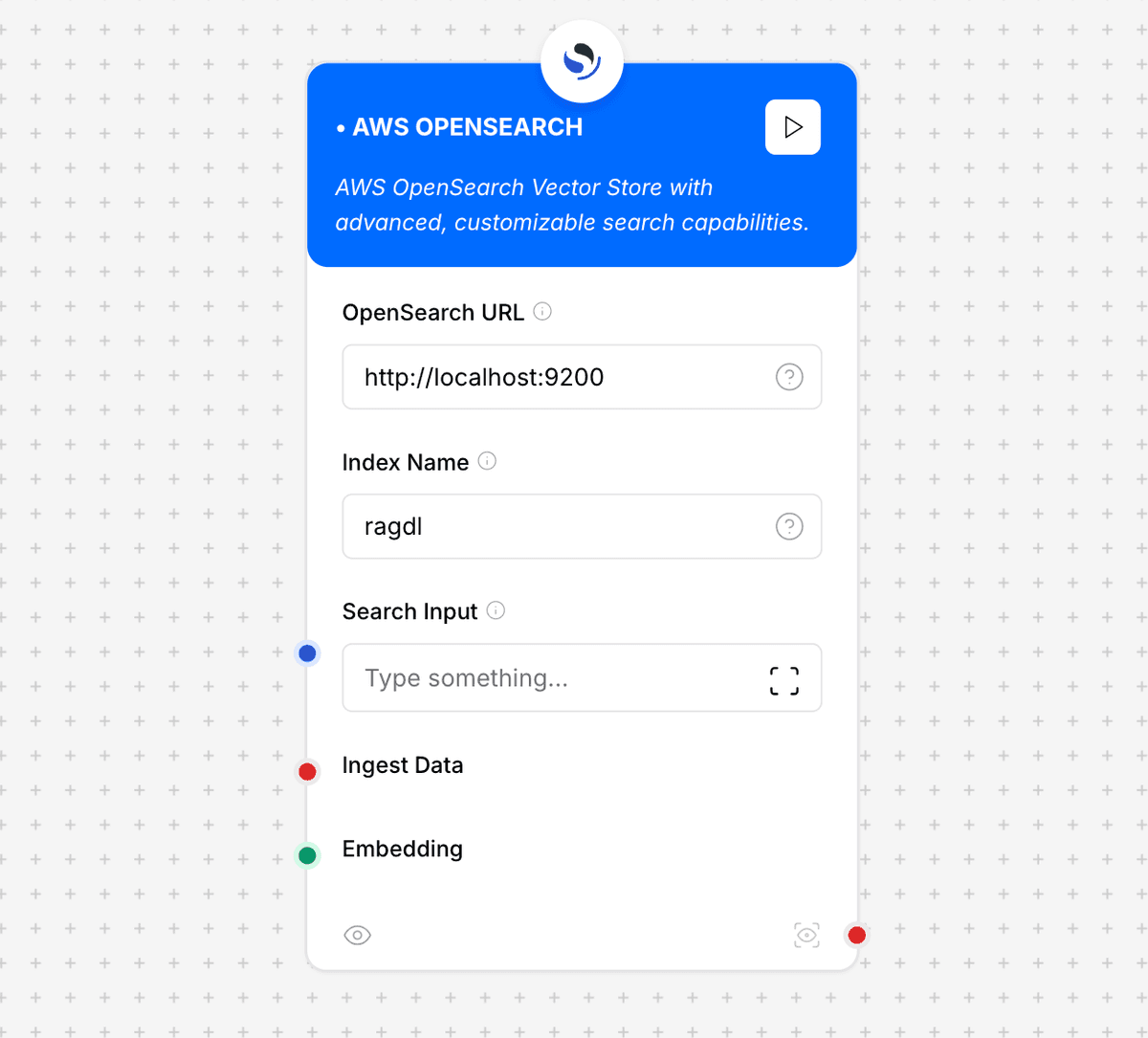
Azure Cosmos DB Loader Architecture
2. AWS SQL Schema Reader
Description
This connector allows you to read and analyze the schema of SQL databases hosted on RDS or other compatible services.
Use Cases
- Database inspection and documentation
- Automatic generation of data models
Inputs
- Database Endpoint: Database address
- Port: Connection port
- Database User: User name
- Database Password: Password
- Database Name: Database name
- Database Type: Database type (PostgreSQL, MySQL, etc.)
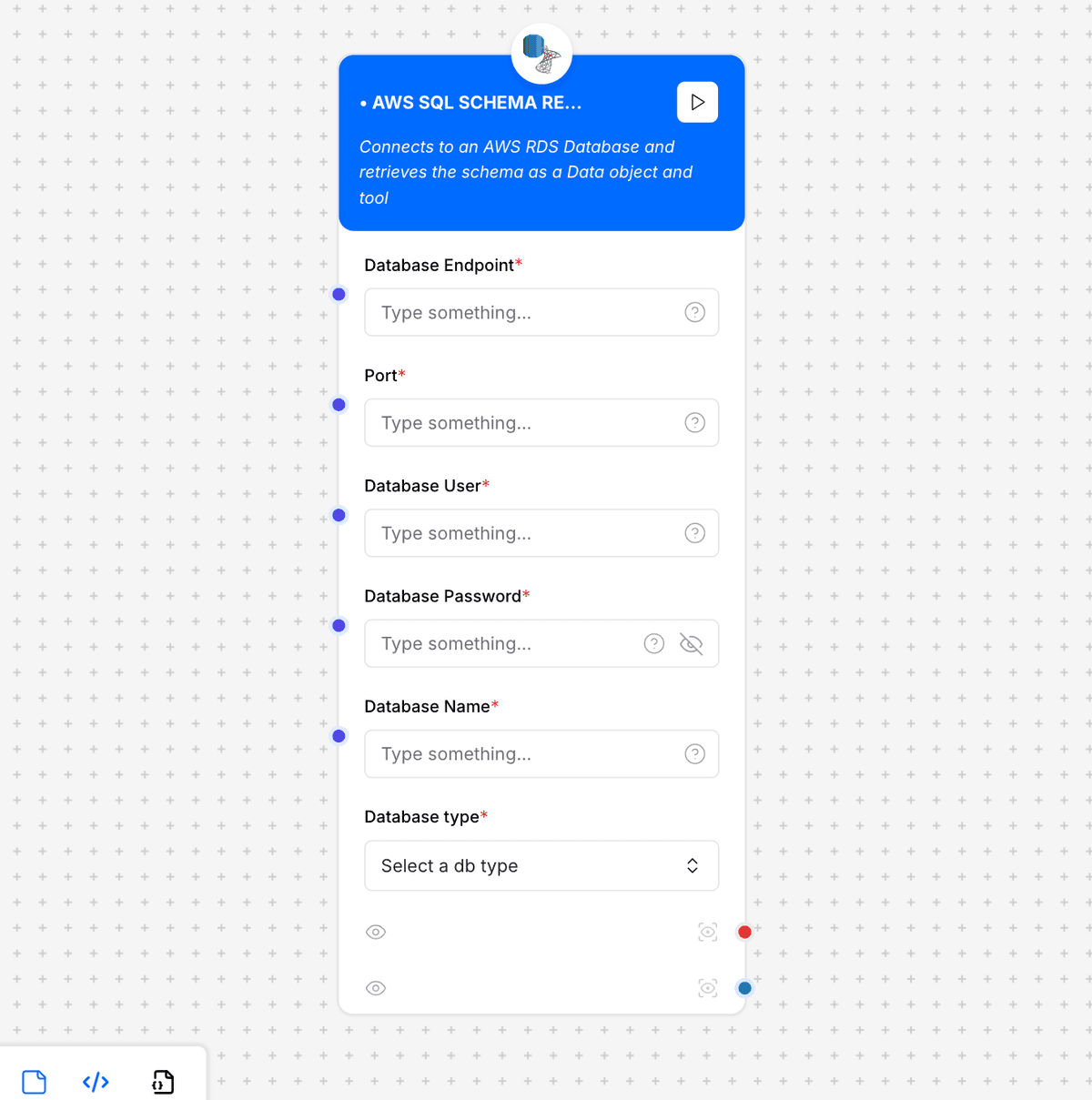
AWS SQL Schema Reader Architecture
3. DynamoDB
Description
The DynamoDB connector provides direct access to DynamoDB tables for read and write operations.
Use Cases
- NoSQL data management
- Storing user sessions or real-time events
Inputs
- AWS Access Key ID: AWS access key
- AWS Secret Access Key: AWS secret key
- AWS Region Name: AWS region
- DynamoDB Table Name: DynamoDB table name
- Session ID: Session ID (optional)
- User Message: User message (optional)
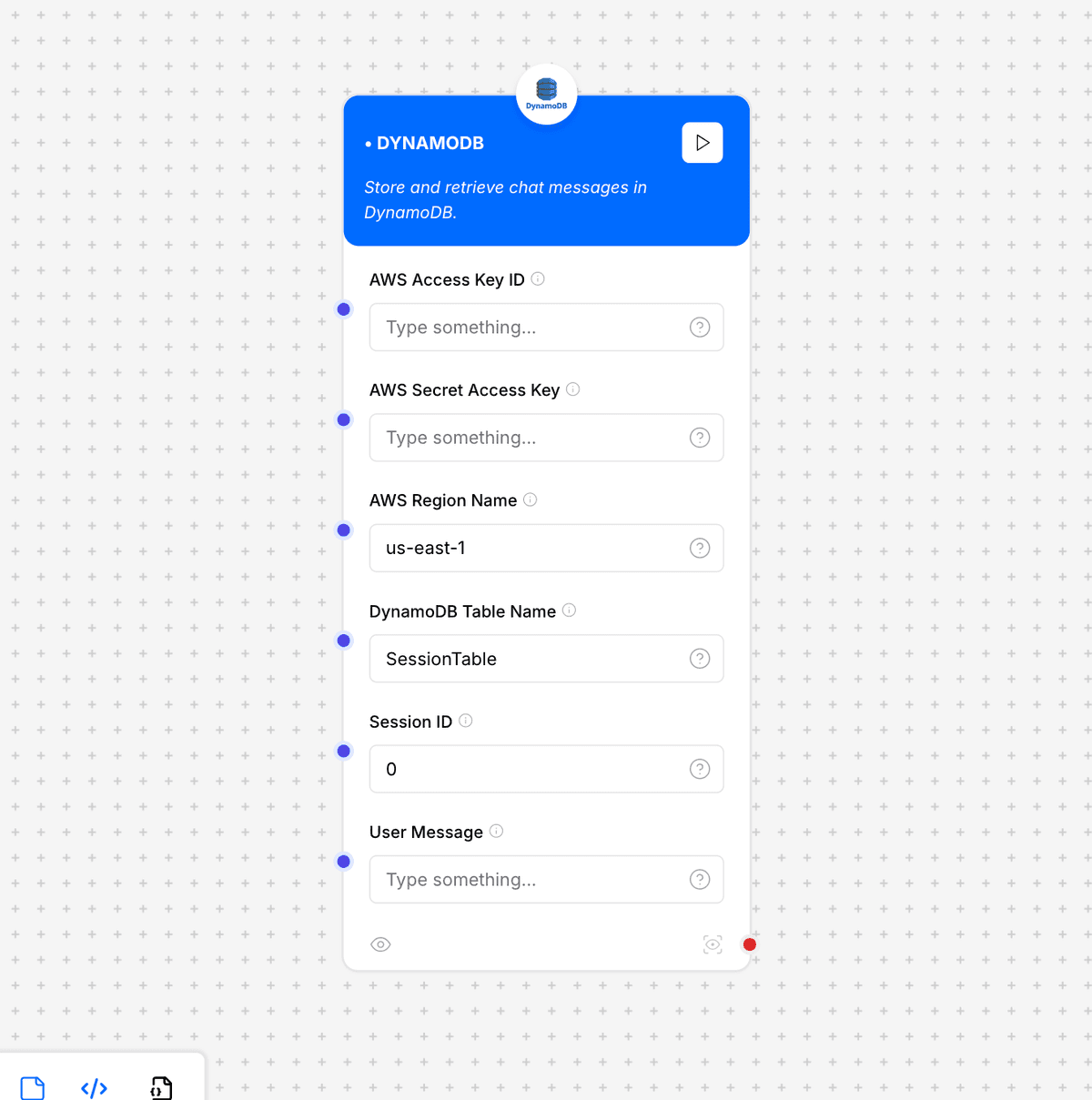
DynamoDB Architecture
4. Glue Data Catalog
Description
This connector allows you to access and manipulate metadata in Amazon Glue Data Catalog.
Use Cases
- Discovering datasets
- Managing ETL pipelines
Inputs
- AWS Access Key ID: AWS Access Key
- AWS Secret Access Key: AWS Secret Key
- AWS Region Name: AWS Region
- Glue Database Name: Glue database name
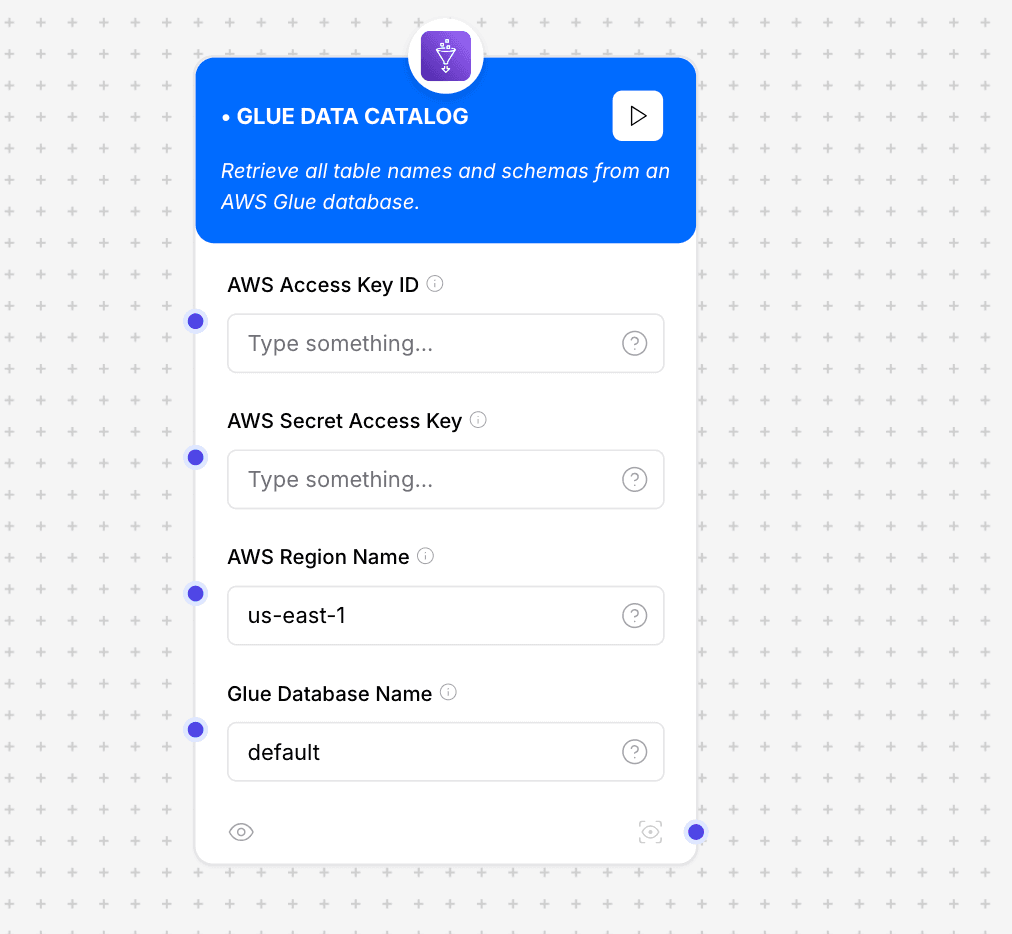
Glue Data Catalog Architecture
5. RDS Agent
Description
The RDS Agent connector makes it easy to manage and monitor RDS instances.
Use Cases
- Monitoring performance
- Automating backups
Inputs
- Input: User input (optional)
- Language Model: Language Model (LLM) to use
- Database URI: Database connection URI
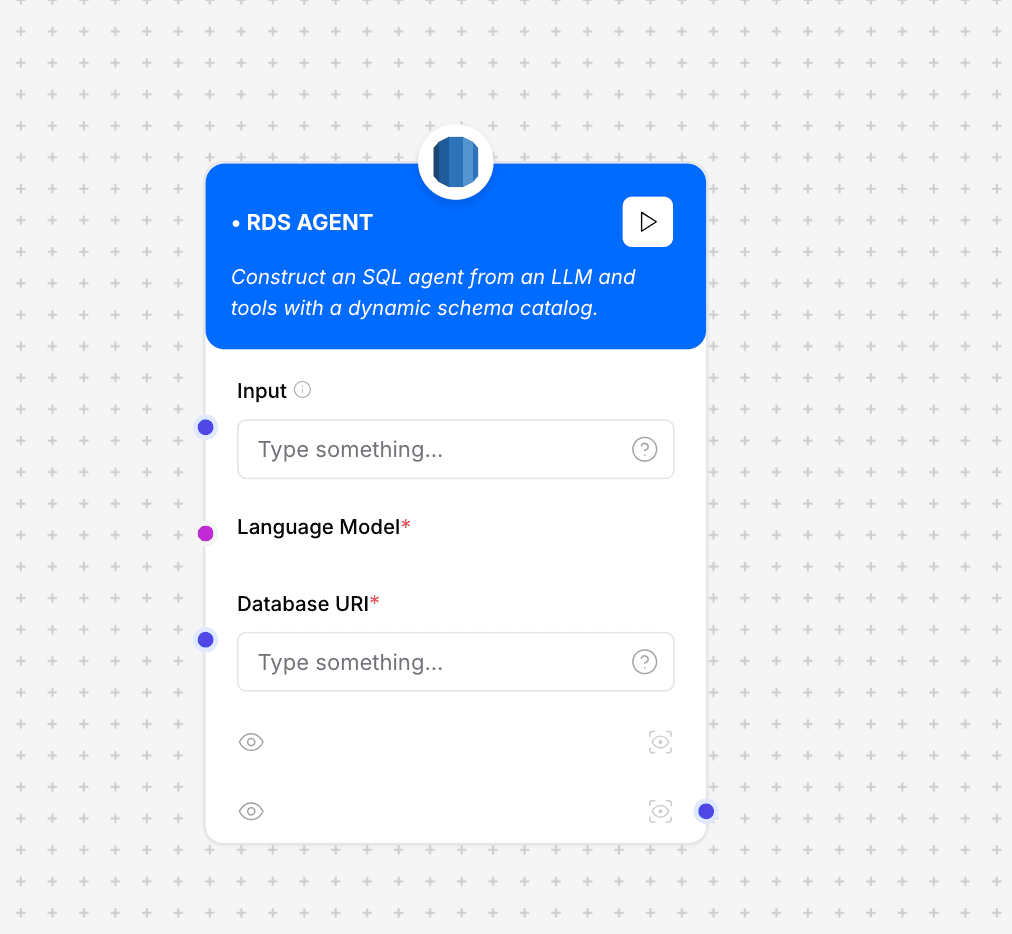
RDS Agent Architecture
6. RDS Connector
Description
This connector allows you to connect to RDS relational databases and perform SQL queries.
Use Cases
- Data extraction and analysis
- Integration with other systems
Inputs
- Database Type: Database type (PostgreSQL, MySQL, etc.)
- Database Username: Username
- Database Password: Password
- RDS Endpoint: RDS server address
- Database Port: Connection port
- Database Name: Database name
- SSL Certificate Path: Path to the SSL certificate (optional)
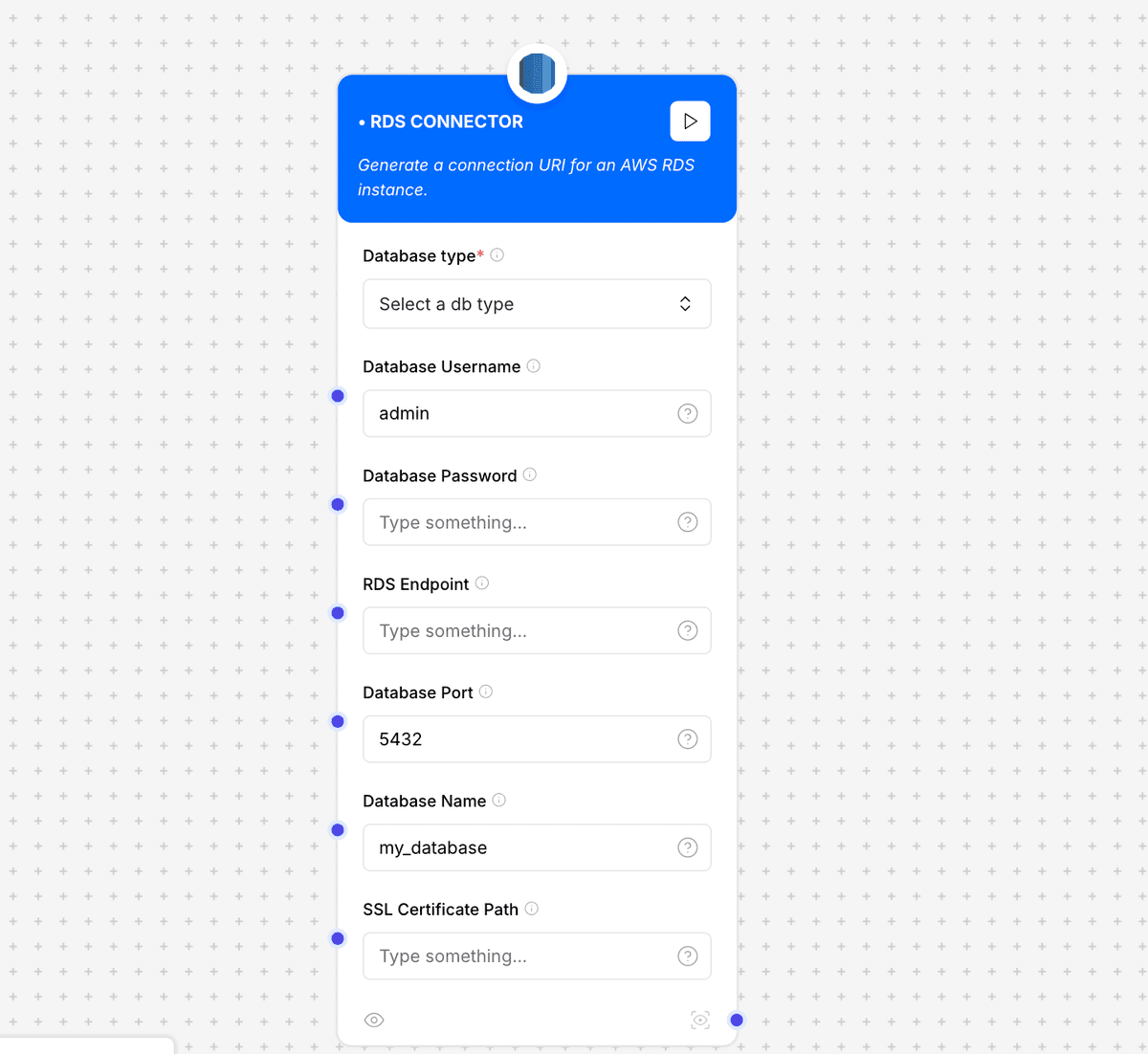
RDS Connector Architecture
7. S3 Directory Loader
Description
This loader allows you to load multiple files from an S3 directory.
Use Cases
- Batch processing of files
- Data migration
Inputs
- Access Key ID: AWS access key
- Secret Access Key: AWS secret key
- Region: AWS region
- Bucket Name: Bucket name
- Directory Path: Directory path
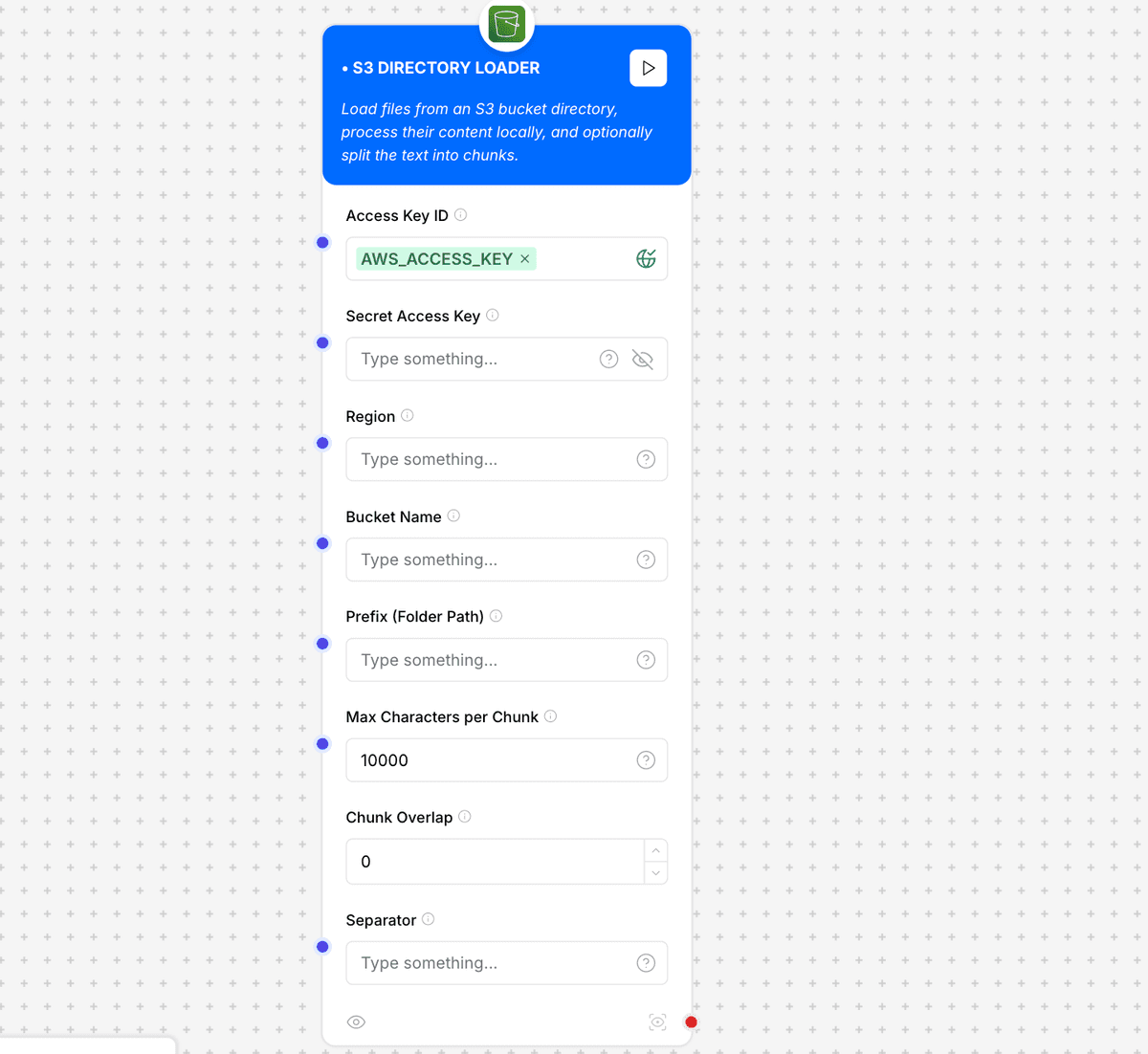
S3 Directory Loader Architecture
8. S3 File Loader
Description
This loader is designed to load a single file from an S3 bucket.
Use Cases
- Loading files for analysis
- Integrating data into workflows
Inputs
- Access Key ID: AWS Access Key
- Secret Access Key: AWS Secret Key
- Region: AWS Region
- Bucket Name: Bucket Name
- File Path: File Path
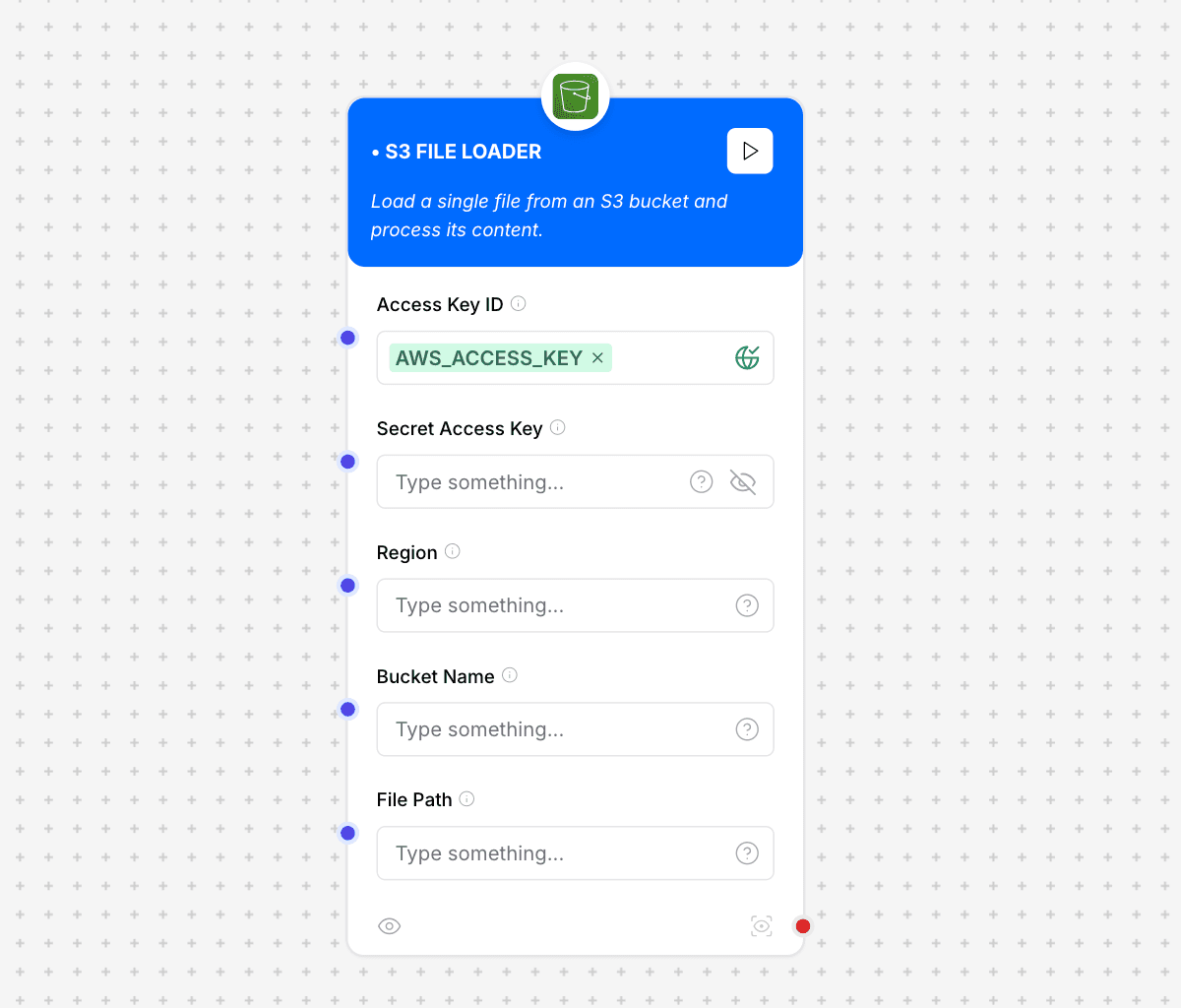
S3 File Loader Architecture
Note: Ensure proper IAM roles and permissions are configured in your AWS account before using these connectors.
Tip: Use AWS Secrets Manager or environment variables to securely store your credentials and access keys.