JSON Cleaner
The JSON Cleaner is a component designed to clean and validate JSON strings, ensuring they are properly formatted and normalized for further processing.
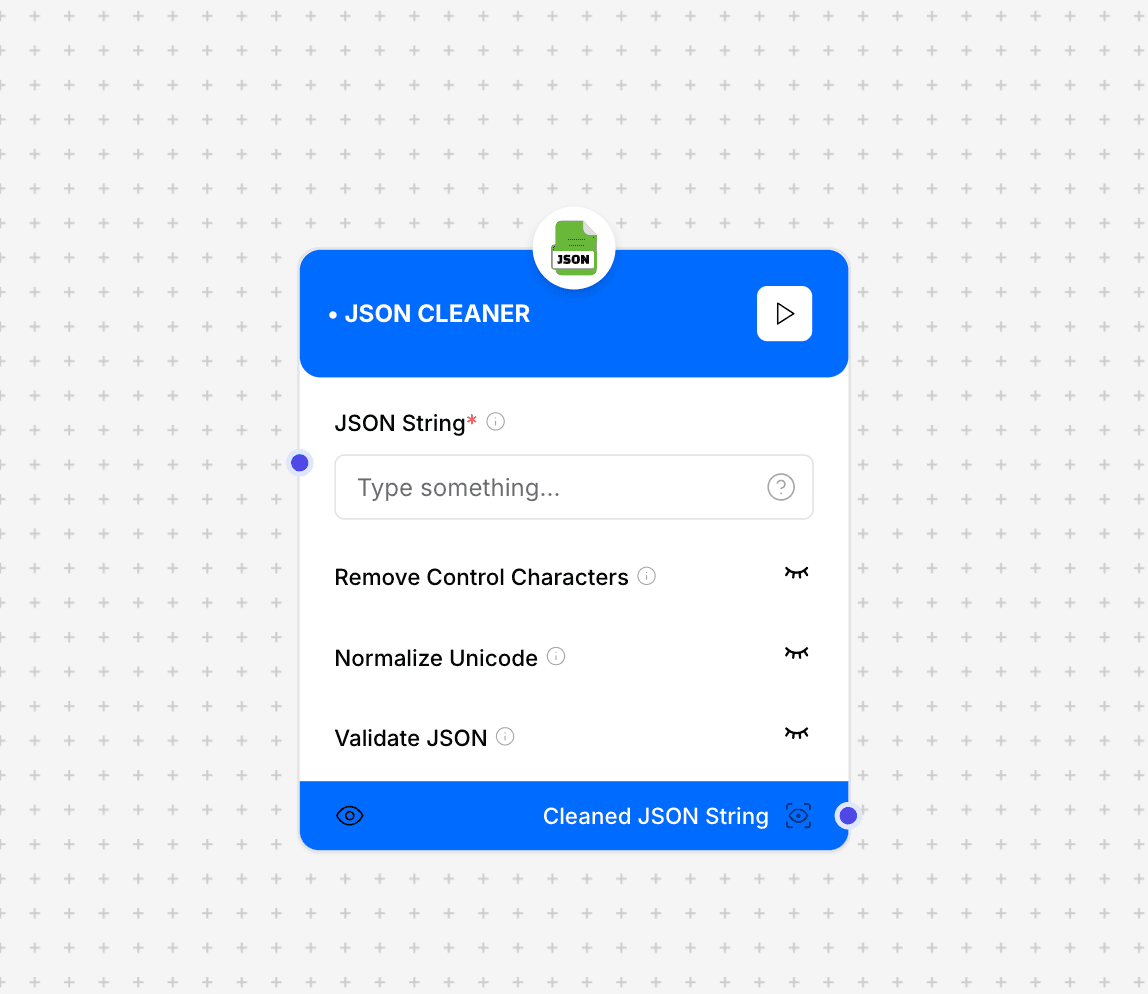
JSON Cleaner interface and configuration
Component Inputs
- JSON String: The input JSON string to be cleaned
Required input field for the JSON content
- Remove Control Characters: Toggle to remove control characters
Optional boolean parameter
- Normalize Unicode: Toggle to normalize Unicode characters
Optional boolean parameter
- Validate JSON: Toggle to validate JSON structure
Optional boolean parameter
Component Outputs
- Cleaned JSON String: The processed and cleaned JSON output
Returns the cleaned and validated JSON string
How It Works
The JSON Cleaner processes input JSON strings through several cleaning and validation steps based on the selected options.
Processing Steps
- Input JSON string validation
- Control character removal (if enabled)
- Unicode normalization (if enabled)
- JSON structure validation (if enabled)
- Output generation
Implementation Example
const jsonCleaner = {
input: '{"text": "Sample\u0020text\nwith\tcontrol\rchars"}',
removeControlChars: true,
normalizeUnicode: true,
validateJSON: true
};
// Output:
// {
// "text": "Sample text with control chars"
// }
Additional Resources
Best Practices
- Always validate input JSON before processing
- Enable Unicode normalization for international text
- Remove control characters when dealing with raw text
- Validate the output JSON structure