GCS Vision OCR
The GCS Vision OCR component leverages Google Cloud's Vision API to extract text from images and documents. It provides high-accuracy optical character recognition capabilities integrated with Google Cloud Storage for efficient document processing and analysis.
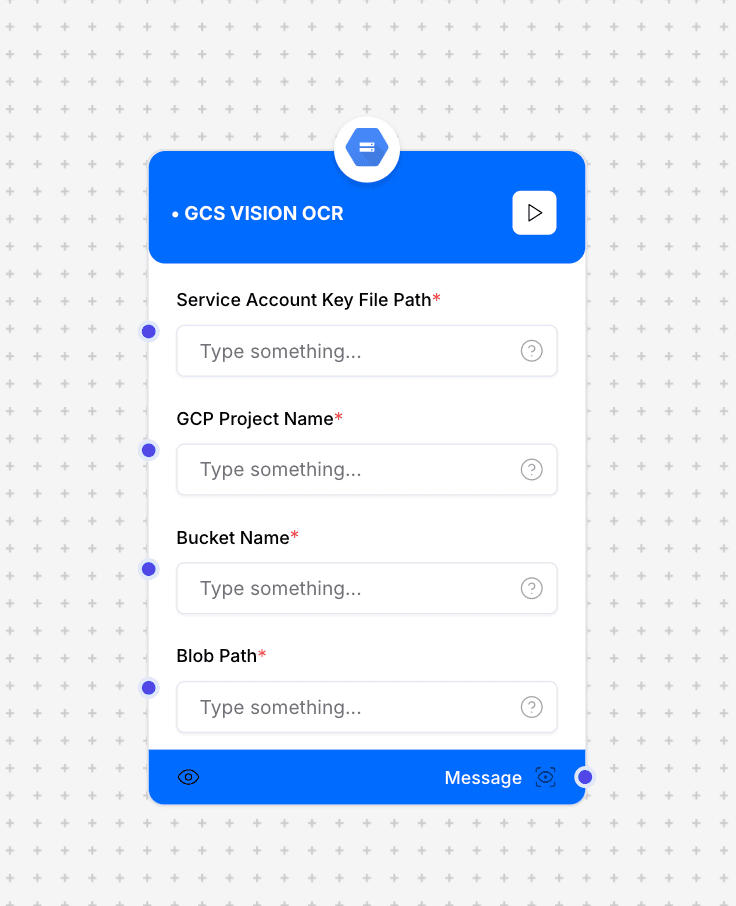
GCS Vision OCR interface and configuration
Configuration Note: Ensure your Google Cloud Service Account has appropriate permissions for Vision API and Cloud Storage access. The service account key file should be securely stored and properly formatted.
Component Inputs
- Service Account Key File Path: Path to the Google Cloud service account key file
Example: "/path/to/service-account-key.json"
- GCP Project Name: Google Cloud Platform project identifier
Example: "my-gcp-project-123456"
- Bucket Name: Google Cloud Storage bucket name for document storage
Example: "document-ocr-bucket"
- Blob Path: Path to the file within the GCS bucket
Example: "documents/invoice.pdf"
Component Outputs
- Extracted Text: Full text content extracted from the document
Example: Complete text extracted from the processed document
- Message: Status message about the OCR operation
Example: "OCR processing successful" or error details
- Text Blocks: Structured representation of text with positioning information
Contains text segments with their location coordinates in the document
How It Works
The GCS Vision OCR component connects to Google Cloud Vision API and processes documents stored in Google Cloud Storage. It authenticates using the provided service account credentials, retrieves the document from the specified bucket location, and applies OCR processing to extract text and structural information.
Processing Flow
- Authentication with Google Cloud using the service account key
- Identification of the target document in Cloud Storage
- Vision API request for OCR processing
- Text extraction and structural analysis
- Results compilation and formatting
- Return of extracted text and metadata
Use Cases
- Document Digitization: Convert scanned documents to searchable text
- Form Processing: Extract data from structured forms and applications
- Invoice Analysis: Automate extraction of invoice details and financial information
- Receipt Processing: Digitize expense receipts for accounting systems
- Legal Document Analysis: Extract text from legal contracts and documents
Implementation Example
const gcsVisionOCR = new GCSVisionOCR({
serviceAccountKeyFile: "/path/to/service-account-key.json",
gcpProjectName: "my-gcp-project-123456",
bucketName: "document-ocr-bucket",
blobPath: "invoices/invoice-2023-05-12.pdf"
});
const result = await gcsVisionOCR.processDocument();
// Output:
// {
// extractedText: "Invoice #12345\nDate: May 12, 2023\nCustomer: Acme Corp\n...",
// message: "OCR processing successful",
// textBlocks: [
// {
// text: "Invoice #12345",
// boundingBox: {x1: 50, y1: 100, x2: 200, y2: 120},
// confidence: 0.98
// },
// // Additional text blocks with positioning data
// ]
// }
Best Practices
- Use high-resolution scans for better OCR accuracy
- Consider preprocessing images to improve contrast and readability
- Organize documents in Cloud Storage using logical folder structures
- Use appropriate IAM permissions to limit access to sensitive documents
- Implement retry logic for large documents or network interruptions