LM Studio Component
Drag & Drop Local LLM Component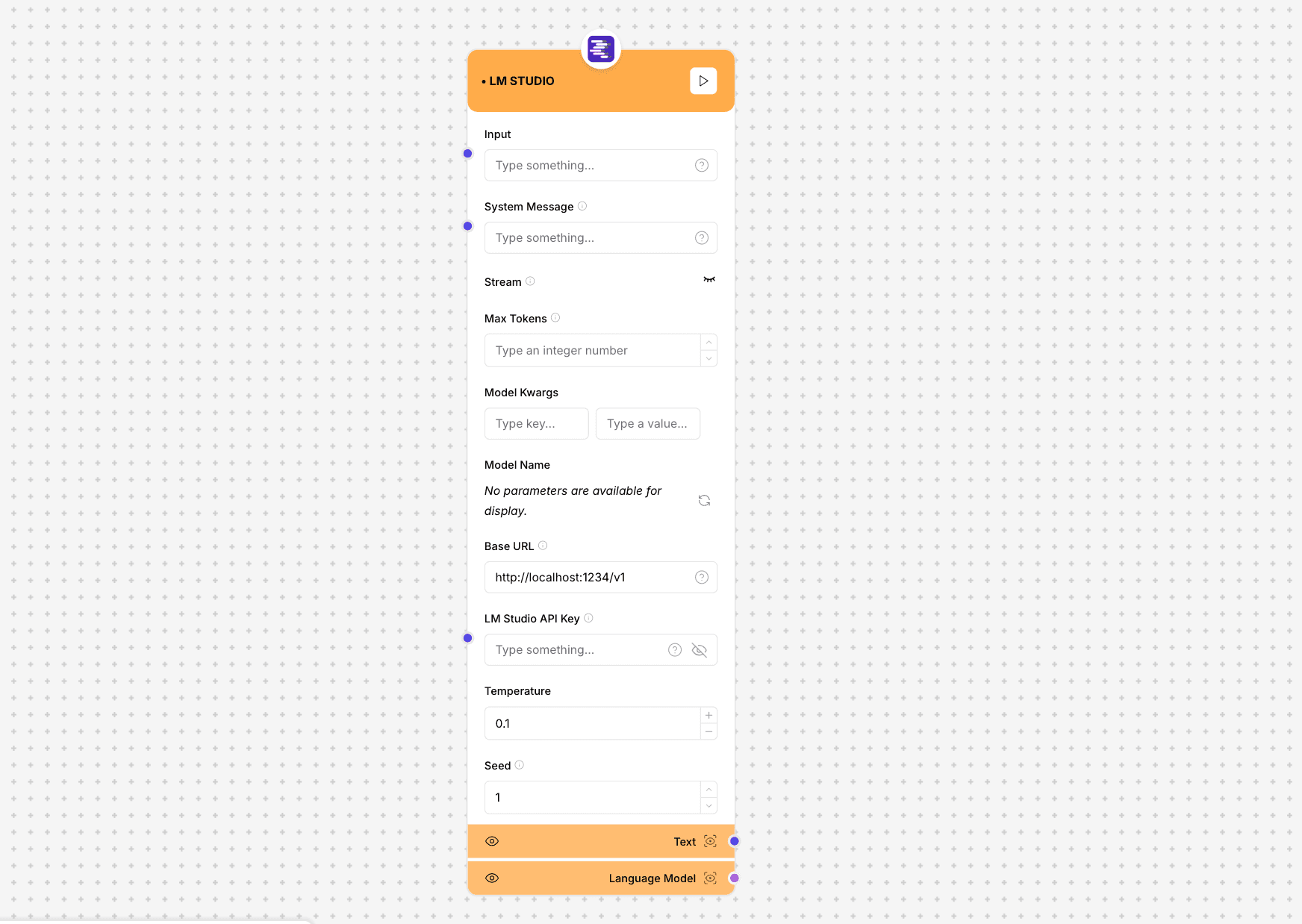
Overview
A drag-and-drop component for integrating with LM Studio's local LLM server. Configure model parameters and connect inputs/outputs while keeping all processing on your local machine.
Component Configuration
Basic Parameters
Input
Text input for the modelSystem Message
System prompt to guide model behaviorStream
Toggle for streaming responsesBase URL
Default: http://localhost:1234/v1
API Configuration
LM Studio API Key
Your API authentication keyModel Kwargs
Additional model parametersModel Name
Selected model identifier
Generation Parameters
Max Tokens
Maximum number of tokens to generateTemperature
Creativity control (0.1 default)Seed
Random seed for reproducibility (1 default)
Output Connections
Text
Generated text outputLanguage Model
Model information and metadata
Usage Tips
- Ensure LM Studio server is running
- Verify correct base URL
- Use consistent seeds for reproducibility
- Start with low temperature values
Best Practices
- Monitor system resources
- Set appropriate token limits
- Test with streaming enabled
- Implement proper error handling