Secrets Redactor Agent
The Secrets Redactor Agent detects and masks sensitive information such as API keys, tokens, and credentials in text. It helps prevent accidental exposure of confidential information through pattern matching and contextual analysis.
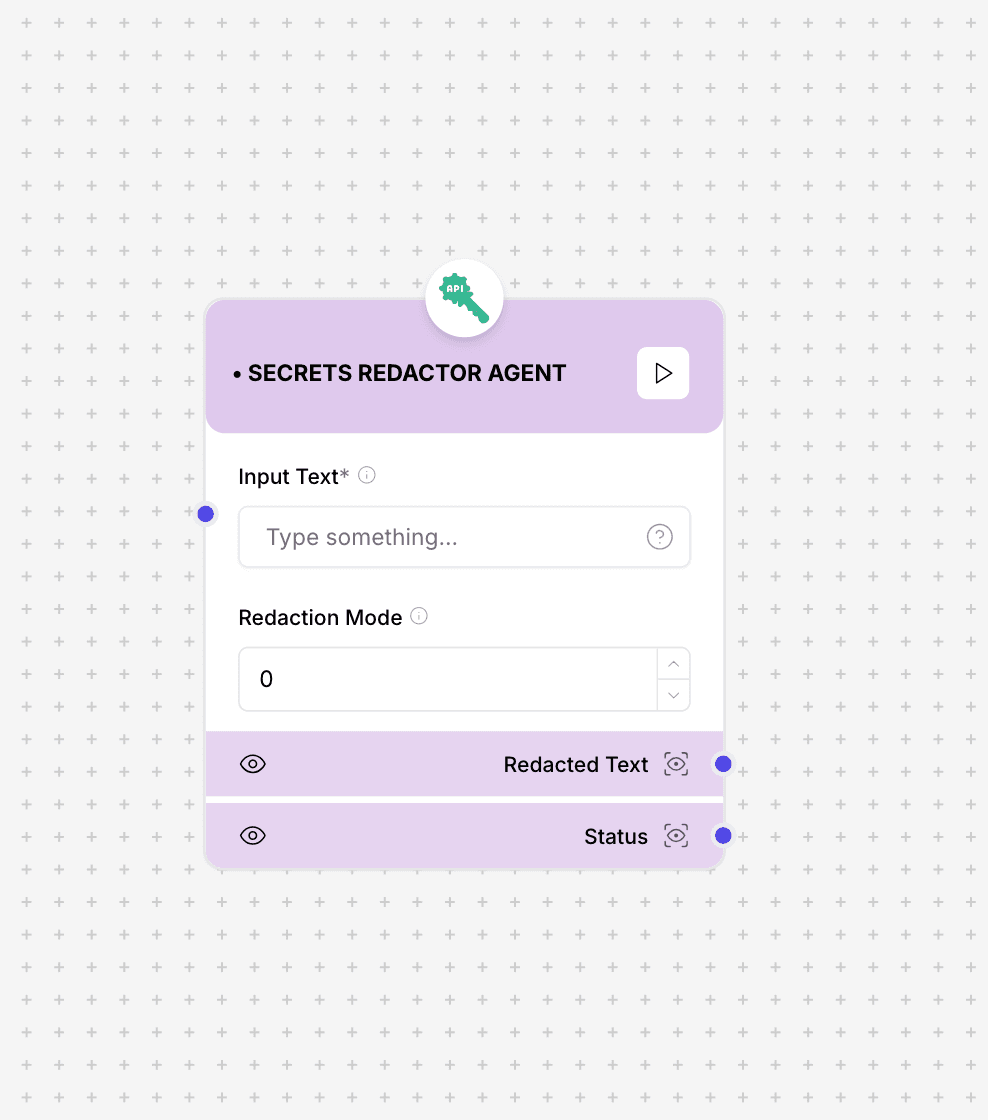
Secrets Redactor Agent interface and configuration
Security Notice: While the Secrets Redactor helps identify and redact common patterns of credentials, it should not be the only security measure in place. Implement comprehensive security practices to protect sensitive information throughout your systems.
Component Inputs
- Input Text: The text content to be analyzed for sensitive information
Example: "My API key is sk_live_51NUoLfGjn6J5YGRtCkJuqJYw3PYyfcGP6W10jRvuZ8Iwi2HlyDFJF"
- Redaction Mode: The level of redaction to apply
Options: 0 (complete), 1 (partial), 2 (tagged)
Example for each mode with an API key:
- 0: "[REDACTED]"
- 1: "sk_live_****************************"
- 2: "[API_KEY: sk_live_51NUoLfGjn6...]"
Component Outputs
- Redacted Text: The processed text with sensitive information redacted
Example: "My API key is [REDACTED]"
- Status: Indication of whether sensitive information was detected
Values: OK (no sensitive info detected), WARNING (sensitive information detected and redacted)
- Risk Score: Numerical evaluation of the sensitivity risk level
Scale: 0.0 (no risk) to 1.0 (high risk)
Detected Secret Types
Common Credentials
- API Keys
- OAuth Tokens
- JWT Tokens
- Access Tokens
- Private Keys
- Session Tokens
Service-Specific
- AWS Keys
- Google API Keys
- GitHub Tokens
- Stripe API Keys
- Database Connection Strings
- SSH Private Keys
How It Works
The Secrets Redactor Agent employs pattern matching through regular expressions and contextual analysis to identify various types of credentials. It analyzes both the structure of potential secrets and surrounding context indicators like variable names and common prefixes.
Detection Techniques
- Pattern-based detection using specialized regex for each credential type
- Entropy analysis to identify random strings likely to be secrets
- Contextual clues like variable naming patterns (e.g., api_key, secret_token)
- Format validation for specific credential types
- Common prefixes detection (sk_, ghp_, AKIA, etc.)
Use Cases
- Chat Applications: Prevent users from accidentally sharing API keys in messages
- Code Review Tools: Scan code submissions for accidentally committed credentials
- Content Management: Sanitize user-generated content to remove exposed secrets
- Documentation Systems: Ensure technical documentation doesn't contain real credentials
- Data Loss Prevention: Monitor outgoing communications for credential leakage
Implementation Example
const secretsRedactor = new SecretsRedactor({
redactionMode: 0, // Complete redaction
secretTypes: [
"API_KEY",
"ACCESS_TOKEN",
"CONNECTION_STRING",
"PRIVATE_KEY"
]
});
const inputText = "check the following AWS key: AKIAIOSFODNN7EXAMPLE ";
const result = secretsRedactor.process(inputText);
// Output:
// {
// redactedText: " check the following AWS key: [REDACTED] ",
// status: "WARNING",
// riskScore: 0.85,
// detectedSecrets: [
// {
// type: "AWS_KEY",
// position: { start: 37, end: 57 },
// confidence: 0.96
// }
// ]
// }
Best Practices
- Use complete redaction (mode 0) for highest security in most scenarios
- Implement pre-submission scanning to catch credentials before they enter your system
- Regularly update detection patterns as new credential formats emerge
- Combine with other security measures like encryption and access controls
- Educate users about the risks of sharing credentials in communications