Calculator
A component that evaluates mathematical expressions and performs calculations. The Calculator component provides a reliable way to integrate computation capabilities into your workflows.
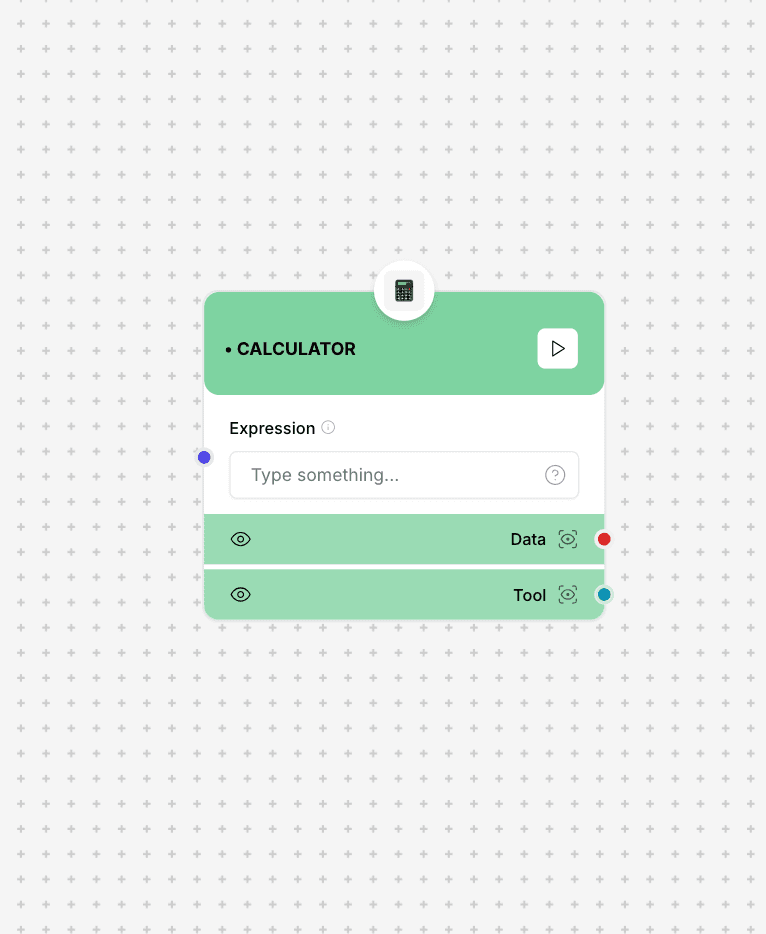
Calculator component interface and configuration
Expression Safety: The Calculator component runs in a sandboxed environment for security. Complex expressions are supported, but you should avoid expressions that could cause infinite loops or excessive memory usage.
Component Inputs
- Expression: Mathematical expression to evaluate
Example: "2 + 2", "sqrt(16) * 5", "sin(45 * PI/180)"
- Toolset Configuration: Optional settings for the calculator engine
Example: precision: 10, notation: 'scientific'
Component Outputs
- Toolset: Calculation result
Example: 4 (for "2 + 2"), 20 (for "sqrt(16) * 5"), 0.7071 (for "sin(45 * PI/180)")
Supported Operations
Basic Arithmetic
Standard mathematical operations
Addition (+): 3 + 4
Subtraction (-): 7 - 2
Multiplication (*): 5 * 6
Division (/): 10 / 2
Exponentiation (^): 2^3 or 2**3
Trigonometric Functions
Angle-related calculations (input in radians by default)
sin(), cos(), tan(), asin(), acos(), atan()
Example: sin(PI/2), cos(0), tan(PI/4)
Logarithmic Functions
Logarithm calculations
log() - Natural logarithm (base e)
log10() - Base 10 logarithm
Example: log(2.718), log10(100)
Statistical Functions
Operations on data sets
mean([a, b, c]) - Average of values
std([a, b, c]) - Standard deviation
min(), max() - Minimum/maximum values
Example: mean([1, 2, 3, 4, 5]), max([10, 20, 30])
Constants
Built-in mathematical constants
PI - 3.14159...
E - 2.71828...
PHI - 1.61803... (Golden ratio)
Example: 2 * PI, E^2
Implementation Example
// Example: Using the calculator in financial calculations
async function calculateLoanPayment(principal, annualRate, years) {
// Convert annual rate to monthly rate (decimal)
const monthlyRate = await calculatorComponent.execute({
expression: `${annualRate} / 100 / 12`
});
// Calculate total number of payments
const payments = await calculatorComponent.execute({
expression: `${years} * 12`
});
// Calculate monthly payment using loan formula
const monthlyPayment = await calculatorComponent.execute({
expression: `${principal} * (${monthlyRate.toolset} * (1 + ${monthlyRate.toolset})^${payments.toolset}) / ((1 + ${monthlyRate.toolset})^${payments.toolset} - 1)`,
toolsetConfiguration: {
precision: 2
}
});
// Calculate total payment amount
const totalPayment = await calculatorComponent.execute({
expression: `${monthlyPayment.toolset} * ${payments.toolset}`,
toolsetConfiguration: {
precision: 2
}
});
// Calculate total interest paid
const totalInterest = await calculatorComponent.execute({
expression: `${totalPayment.toolset} - ${principal}`,
toolsetConfiguration: {
precision: 2
}
});
// Return the complete loan analysis
return {
principalAmount: principal,
annualInterestRate: annualRate,
loanTermYears: years,
monthlyPayment: monthlyPayment.toolset,
totalPayments: totalPayment.toolset,
totalInterest: totalInterest.toolset
};
}
Use Cases
- Financial Analysis: Calculate investment returns, loan payments, and financial metrics
- Data Processing: Perform statistical operations on data sets
- Engineering Calculations: Solve complex mathematical formulas for engineering applications
- Unit Conversion: Convert between different units of measurement
- Scientific Computing: Perform scientific calculations involving advanced mathematical operations
Best Practices
- Break complex calculations into smaller, manageable steps
- Use parentheses to ensure proper operation precedence
- Set appropriate precision levels for financial and scientific calculations
- Validate inputs before passing them to the calculator
- Use constants (PI, E) rather than decimal approximations
- Consider angle units (radians vs. degrees) for trigonometric functions
- Test expressions with edge cases to ensure reliable results