AgentSQL Query Data
The AgentSQL Query Data component provides a secure and efficient way to execute SQL queries with API-based authentication and parameter handling.
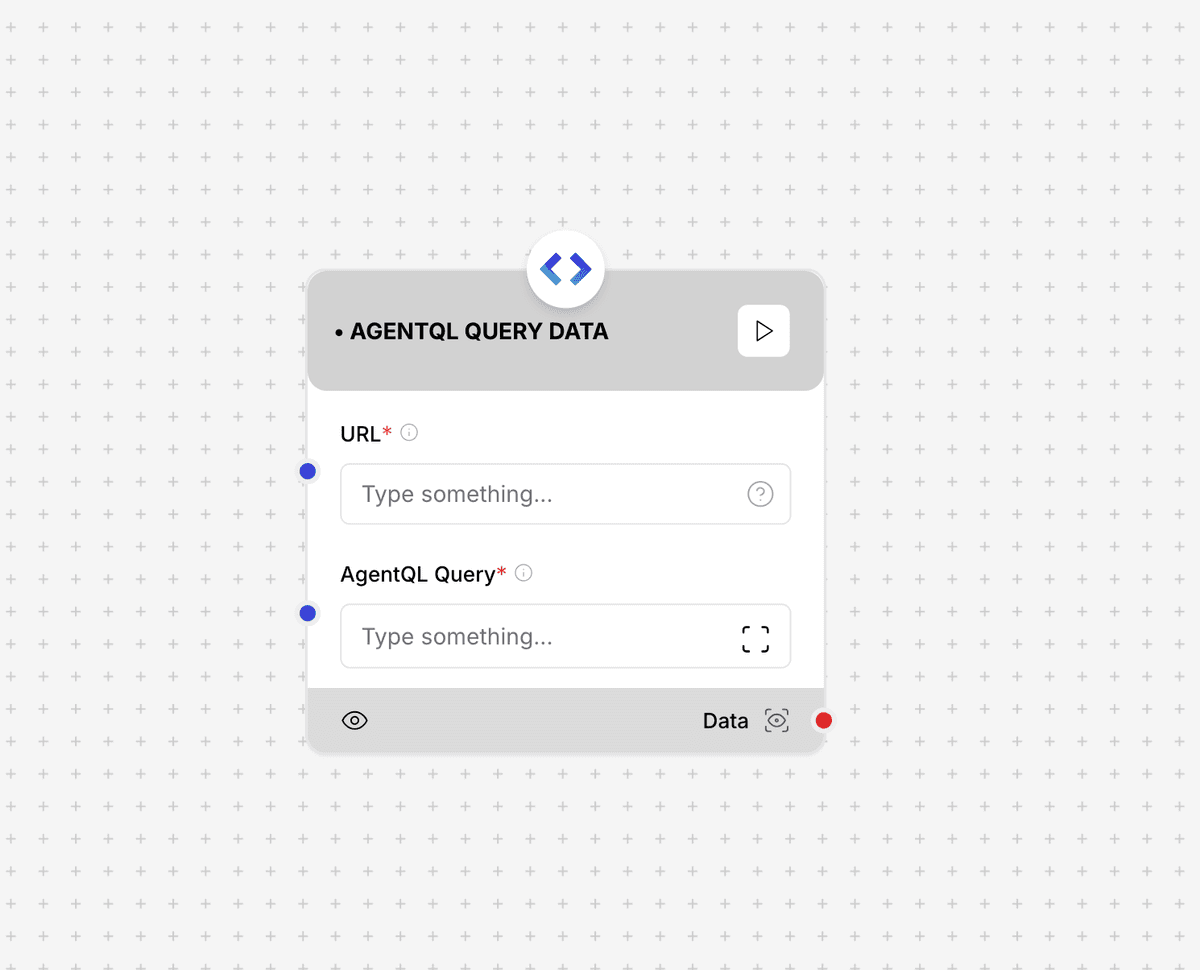
AgentSQL Query Data interface and configuration options
Configuration Parameters
Required Parameters
- Agent API Key: Authentication key
- Endpoint: API endpoint URL
- Additional Params: SQL parameters
Output Format
{ "results": { "data": array, "metadata": { "rowCount": number, "executionTime": string, "queryType": string } } }
Example Usage
const agentSQLQuery = new AgentSQLQueryData({ agentApiKey: "YOUR_API_KEY", endpoint: "https://api.example.com/sql", additionalParams: { query: "SELECT * FROM users WHERE status = ?", params: ["active"] } }); const result = await agentSQLQuery.process({ input: "Fetch all active users" });
Additional Resources
Best Practices
- Use parameterized queries
- Implement rate limiting
- Validate input parameters
- Monitor query performance
- Handle API errors gracefully