SQL Migration Agent
The SQL Migration Agent automates database migrations between different SQL platforms. It handles schema translation, data type mapping, constraint conversion, and ensures data integrity while migrating from one database system to another.
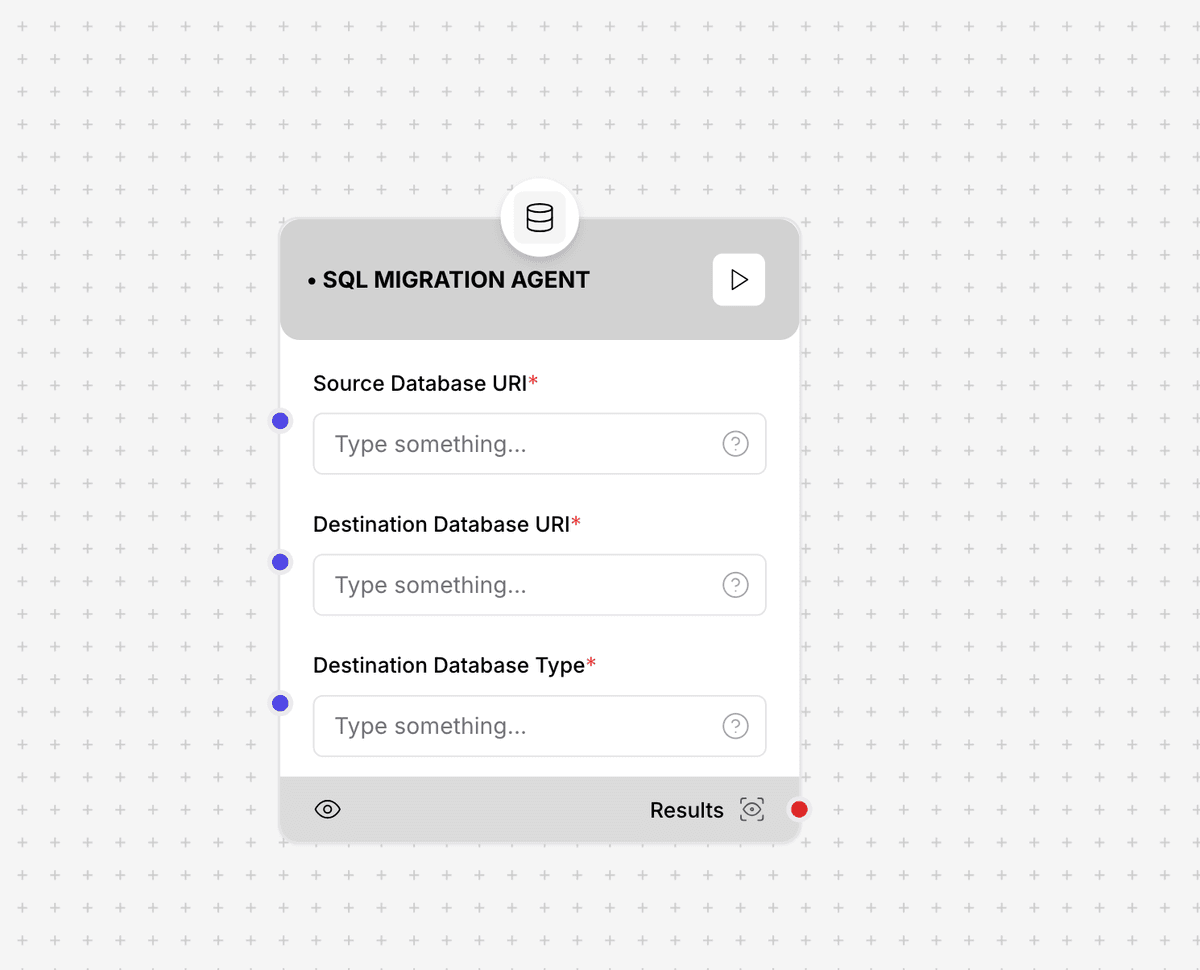
SQL Migration Agent workflow and architecture
Configuration Parameters
Required Input Parameters
- source_database_uri: Connection URI for source database
- destination_database_uri: Connection URI for target database
- destination_database_type: Target database platform
- PostgreSQL
- MySQL
- SQL Server
- Oracle
- SQLite
- openai_api_key: OpenAI API key for schema translation
Output Format
{ "migration_results": { "status": "success" | "partial" | "failed", "completion_time": string, "schema_migration": { "tables_migrated": number, "views_migrated": number, "procedures_migrated": number, "functions_migrated": number, "triggers_migrated": number, "constraints_migrated": number }, "data_migration": { "total_rows": number, "rows_migrated": number, "bytes_transferred": number, "tables_completed": array }, "type_mappings": [ { "source_type": string, "destination_type": string, "conversion_notes": string } ], "errors": [ { "type": string, "object": string, "message": string, "severity": "high" | "medium" | "low" } ], "warnings": [ { "type": string, "object": string, "message": string, "impact": string } ], "performance_metrics": { "total_duration": number, "schema_analysis_time": number, "data_transfer_time": number, "validation_time": number } } }
Features
- Automated schema translation
- Data type mapping
- Constraint preservation
- Index optimization
- Stored procedure conversion
- Trigger migration
- Data validation
- Progress tracking
Note: Always backup your source database before migration. Some complex stored procedures or platform-specific features may require manual review after migration.
Tip: Test the migration with a subset of data first. Consider running migrations during off-peak hours and implement appropriate monitoring for long-running migrations.
Example Usage
const migrationAgent = new SQLMigrationAgent({ source_database_uri: "mysql://user:pass@source-host:3306/db", destination_database_uri: "postgresql://user:pass@dest-host:5432/db", destination_database_type: "PostgreSQL", openai_api_key: "sk-..." }); const results = await migrationAgent.migrate();