Yahoo Finance
A component that retrieves financial data from Yahoo Finance. Connect stock symbols and retrieval parameters to access up-to-date market information, historical prices, and financial metrics.
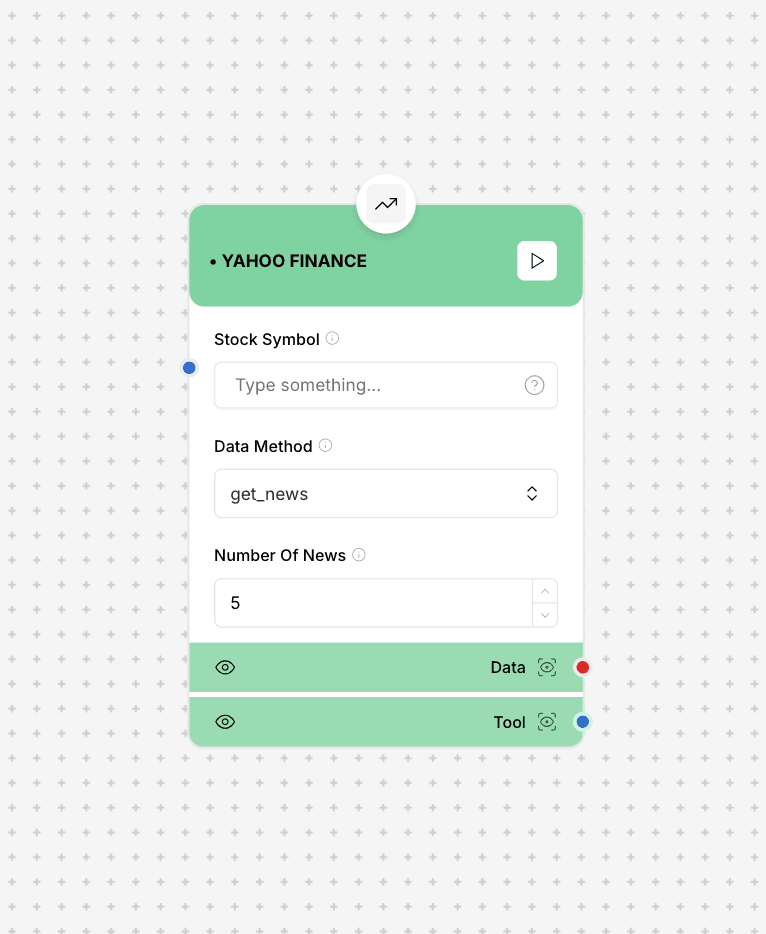
Yahoo Finance component interface and configuration
Data Limitations: The component relies on Yahoo Finance's API availability. Be aware of rate limits and data refresh frequencies. Some data may be delayed by 15-20 minutes depending on the exchange.
Component Inputs
- Stock Symbol: Ticker symbol for the financial instrument
Example: "AAPL" (Apple Inc.), "MSFT" (Microsoft), "^GSPC" (S&P 500 Index)
- Data Method: Type of data to retrieve
Example: "get_news", "get_history", "get_financials", "get_info"
- Number of News: For news retrieval, maximum number of articles
Example: 5 (retrieve the 5 most recent news articles)
Component Outputs
- Toolset: Retrieved financial data
Example: Stock information, financial metrics, news articles, or historical data depending on the selected method
Data Methods
get_news
Retrieves recent news articles related to the stock
Returns:
- Array of news articles with title, publisher, link, and publish date
Parameters:
- Number of News: Maximum articles to return
get_history
Retrieves historical price data for the specified symbol
Returns:
- Historical data including open, high, low, close prices, and volume
Parameters:
- Period: e.g., '1d', '5d', '1mo', '3mo', '6mo', '1y', '2y', '5y', 'max'
get_financials
Retrieves financial statements data
Returns:
- Income statements, balance sheets, and cash flow data
Parameters:
- Quarterly: Boolean to toggle between quarterly or annual data
get_info
Retrieves summary information about the stock
Returns:
- Summary data including current price, market cap, 52-week range, volume, and more
Implementation Example
// Example: Retrieving stock information and recent news
async function getStockAnalysis(symbol) {
// Get stock information
const stockInfo = await yahooFinanceComponent.execute({
stockSymbol: symbol,
dataMethod: "get_info"
});
// Get recent news (5 articles)
const stockNews = await yahooFinanceComponent.execute({
stockSymbol: symbol,
dataMethod: "get_news",
numberOfNews: 5
});
// Get historical data for the past month
const historicalData = await yahooFinanceComponent.execute({
stockSymbol: symbol,
dataMethod: "get_history",
period: "1mo"
});
// Combine the results for a comprehensive analysis
return {
symbol: symbol,
currentPrice: stockInfo.toolset.currentPrice,
marketCap: stockInfo.toolset.marketCap,
peRatio: stockInfo.toolset.trailingPE,
recentNews: stockNews.toolset,
priceHistory: historicalData.toolset,
analysisDate: new Date().toISOString()
};
}
Use Cases
- Market Dashboards: Create custom financial dashboards with real-time data
- Investment Research: Gather data for investment analysis and decision making
- Financial Reporting: Automate the collection of market data for reports
- Technical Analysis: Obtain historical data for technical indicator calculations
- Sentiment Analysis: Combine news data with NLP components for market sentiment
Best Practices
- Use proper stock symbols including exchange information when necessary (e.g., "AAPL.NE" for Apple on NEO Exchange)
- Implement caching for frequently accessed data to reduce API calls
- Be aware of market hours when interpreting real-time data
- Consider time zones when working with historical data
- Respect rate limits to prevent access restrictions
- Handle potential data gaps or API failures gracefully
- Validate symbols before querying to prevent errors