OpenAI Component
Drag & Drop LLM Component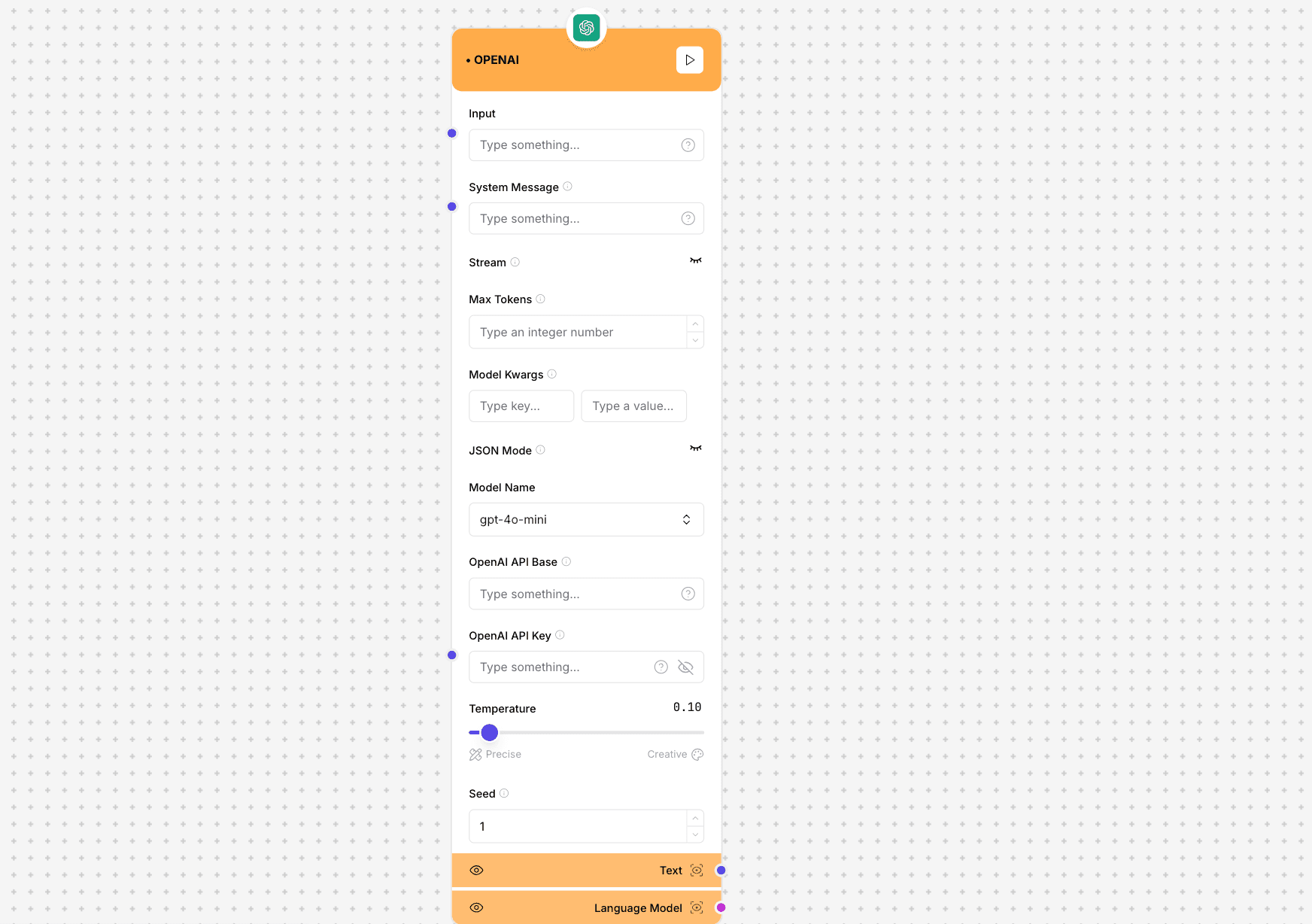
Overview
A drag-and-drop component for integrating OpenAI's language models. Configure model parameters and connect inputs/outputs to leverage OpenAI's powerful AI capabilities.
Component Configuration
Basic Parameters
Input
Text input for the modelSystem Message
System prompt to guide model behaviorStream
Toggle for streaming responsesModel Name
e.g., gpt-4o-mini
API Configuration
OpenAI API Key
Your API authentication keyOpenAI API Base
Custom API endpoint (optional)JSON Mode
Toggle for JSON output format
Generation Parameters
Max Tokens
Maximum tokens to generateTemperature
Creativity control (0.10 default)Seed
Random seed for reproducibility (1 default)Model Kwargs
Additional model parameters
Output Connections
Text
Generated text outputLanguage Model
Model information and metadata
Usage Tips
- Use JSON mode for structured output
- Enable streaming for real-time responses
- Set seed for reproducible results
- Choose appropriate model for task
Best Practices
- Secure API key handling
- Monitor token usage
- Implement proper error handling
- Use system messages effectively