JSON Agent
The JSON Agent provides an intelligent interface for querying and manipulating JSON data. It translates natural language queries into JSON operations, handles complex data structures, and provides detailed analysis of JSON content.
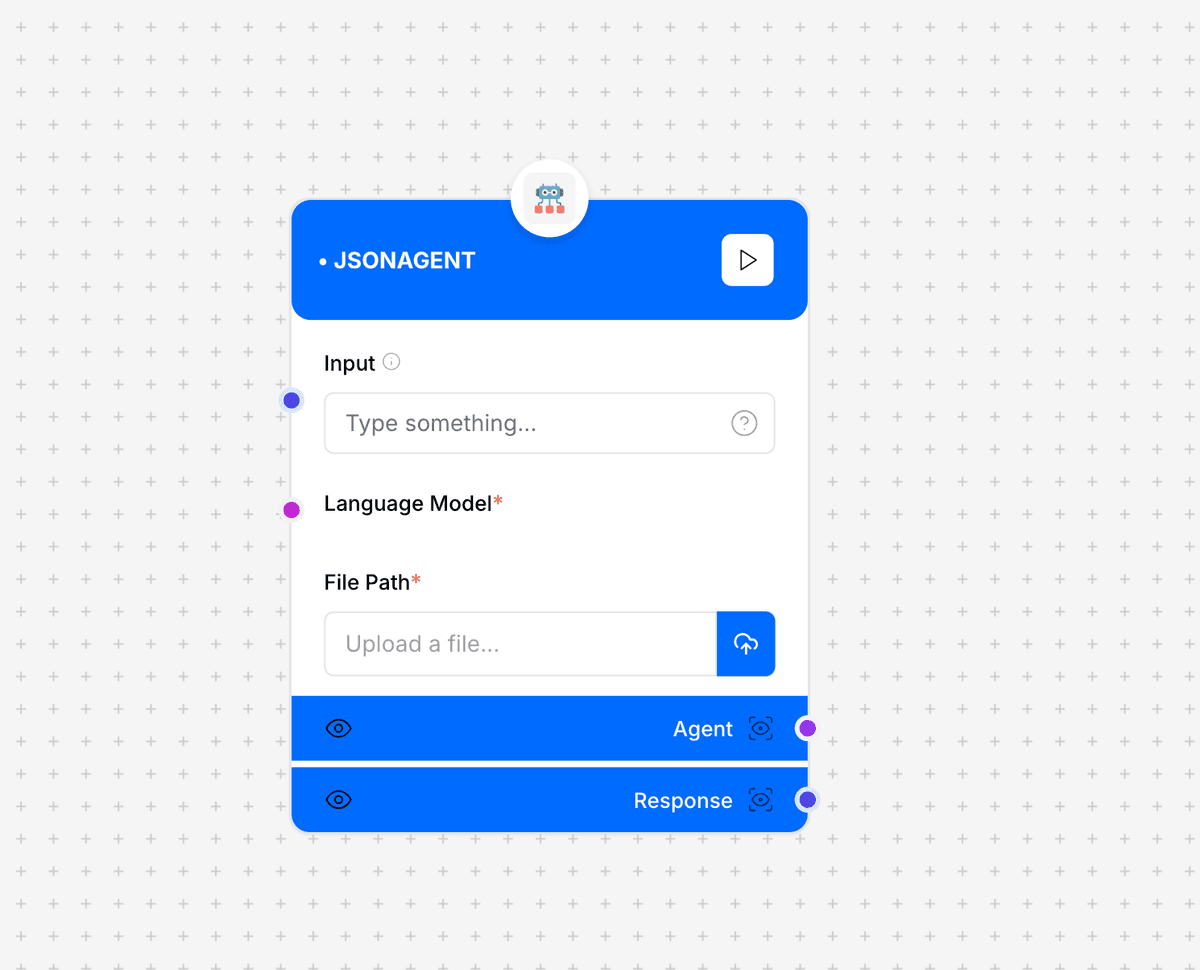
JSON Agent workflow and architecture
Configuration Parameters
Required Input Parameters
- input: Natural language query or instruction
- file_path: Path to JSON file
Optional Configuration
- llm: Language model configuration
- model_name: Model identifier
- temperature: Response creativity
- max_tokens: Response length limit
- json_config: JSON handling options
- max_depth: Maximum nesting level
- array_limit: Maximum array items to process
- preserve_order: Maintain key order
- pretty_print: Format output
Output Format
{ "agent": { "status": "success" | "error", "execution_info": { "operation_type": string, "paths_accessed": array, "modifications_made": array }, "json_analysis": { "structure": { "depth": number, "keys_found": array, "data_types": object }, "validation": { "is_valid": boolean, "issues": array } } }, "response": { "query_result": { "data": any, "type": string, "path": string }, "metadata": { "total_matches": number, "processing_time": number }, "analysis": { "summary": string, "insights": array, "suggestions": array }, "transformations": { "applied": array, "reverted": array, "failed": array } } }
Features
- Natural language JSON querying
- Complex data traversal
- Schema validation
- Data transformation
- Structure analysis
- Error handling
- Performance optimization
- Format preservation
Note: Consider memory usage when processing large JSON files. Use array_limit and max_depth for better performance.
Tip: Use specific queries for better accuracy. Enable pretty_print for more readable output during development.
Example Usage
const jsonAgent = new JSONAgent({ file_path: "./data/complex.json", llm: { model_name: "gpt-4", temperature: 0.3 }, json_config: { max_depth: 5, array_limit: 1000, preserve_order: true, pretty_print: true } }); const response = await jsonAgent.process({ input: "Find all users with active subscriptions and summarize their usage patterns" });