Sequential Task Agent
The Sequential Task Agent is designed to break down and execute complex tasks in a structured, step-by-step manner. It can handle multi-stage operations, delegate subtasks, and maintain context throughout the execution process while providing detailed progress tracking.
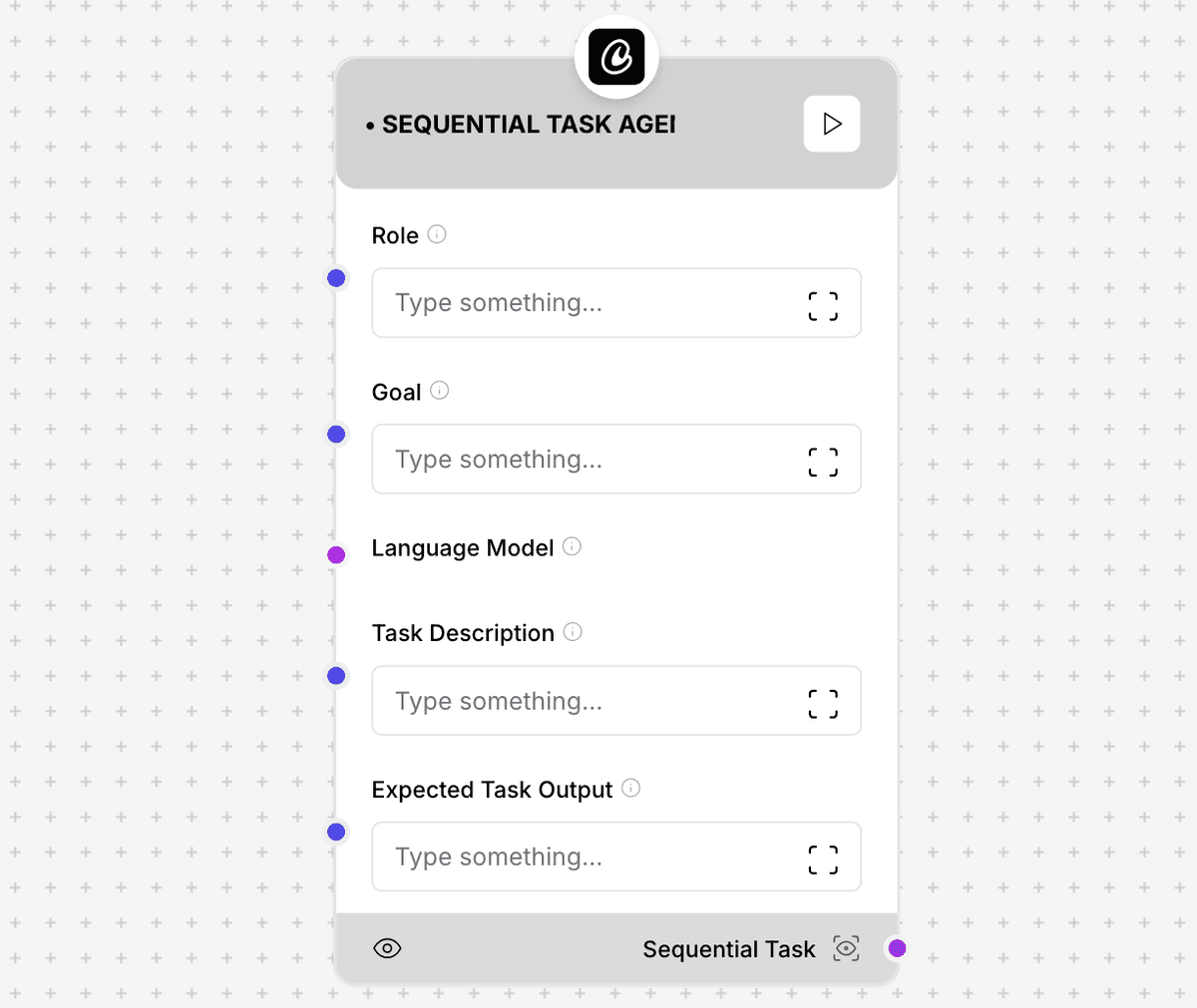
Sequential Task Agent workflow and architecture
Configuration Parameters
Required Input Parameters
- role: The agent's assigned role and responsibilities
- goal: Primary objective to be accomplished
- task_description: Detailed description of the task to be performed
- expected_task_output: Format and requirements for the final output
Optional Configuration
- backstory: Additional context and background for the agent's persona
- tools: Array of available tools and utilities
- llm: Language model configuration (default: GPT-4)
- memory: Memory configuration for maintaining context
- verbose: Enable detailed execution logging (default: false)
- allow_delegation: Enable subtask delegation (default: true)
- allow_code_execution: Enable code execution capabilities (default: false)
- agent_kwargs: Additional agent-specific configuration
Output Format
{ "task_execution": { "status": "completed" | "failed" | "in_progress", "steps": [ { "step_id": string, "description": string, "status": string, "start_time": string, "end_time": string, "output": any, "subtasks": array } ], "dependencies": [ { "from": string, "to": string, "type": string } ] }, "final_output": { "result": any, "format": string, "metadata": object }, "execution_metrics": { "total_duration": number, "steps_completed": number, "resources_used": object }, "agent_state": { "memory_snapshot": object, "context_window": array, "tool_usage": object } }
Features
- Dynamic task decomposition
- Contextual memory management
- Subtask delegation capabilities
- Progress tracking and reporting
- Error handling and recovery
- Tool integration and management
- Code execution sandbox
- Dependency management
Note: When enabling code execution, ensure proper security measures are in place. The agent should run in a sandboxed environment with appropriate permissions and resource limits.
Tip: Use the verbose mode during development to understand the agent's decision-making process. Configure memory settings based on the complexity and duration of your tasks.
Example Usage
const sequentialTaskAgent = new SequentialTaskAgent({ role: "Data Analysis Assistant", goal: "Process and analyze customer feedback data", backstory: "Experienced data analyst with expertise in NLP", tools: ["pandas", "nltk", "matplotlib"], memory: { type: "buffer", max_tokens: 2000 }, allow_delegation: true, verbose: true }); const result = await sequentialTaskAgent.execute({ task_description: "Analyze customer feedback and generate insights", expected_task_output: { format: "report", sections: ["summary", "sentiment", "trends", "recommendations"] } });