ML Task Runner
A component that executes machine learning tasks using pre-defined models and configurations. The ML Task Runner simplifies the integration of machine learning capabilities into your workflows without requiring deep ML expertise.
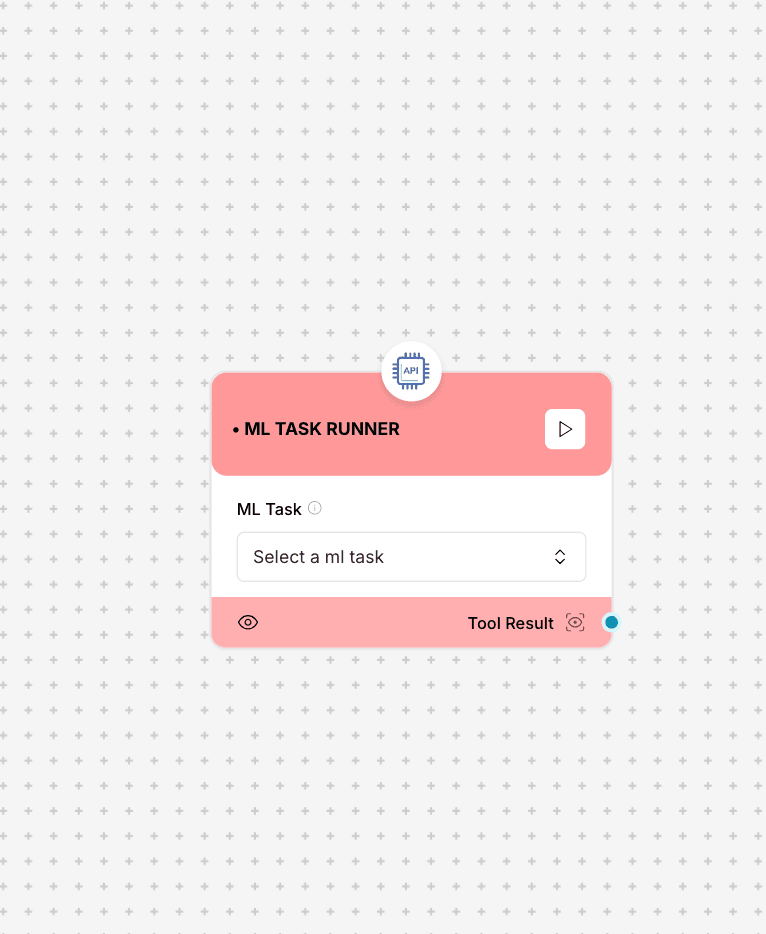
ML Task Runner component interface and configuration
Resource Requirements: ML tasks can be compute-intensive. Ensure that your environment has sufficient resources for the selected ML task. Some complex models may require GPU acceleration for optimal performance.
Component Inputs
- ML Task: Type of machine learning task to perform
Example: "Select a ML task" dropdown with options like "Image Classification", "Text Summarization", "Sentiment Analysis"
- Select a task: Specific pre-configured model to use
Example: Selection from available models for the chosen task category
- Tool Input: Data to process with the selected model
Example: Text for NLP tasks, image URL for vision tasks, data points for prediction tasks
Component Outputs
- Tool Result: Results from the ML model execution
Example: Classification labels and confidence scores, generated text, detected entities, or predictions
Supported ML Task Categories
Computer Vision
Image and video processing tasks
Tasks include:
- Image Classification
- Object Detection
- Image Segmentation
- Face Recognition
- Pose Estimation
Natural Language Processing
Text processing and generation tasks
Tasks include:
- Text Classification
- Named Entity Recognition
- Sentiment Analysis
- Text Summarization
- Question Answering
- Language Translation
Tabular Data
Structured data analysis tasks
Tasks include:
- Regression
- Classification
- Anomaly Detection
- Time Series Forecasting
- Clustering
Multimodal
Tasks that combine multiple data types
Tasks include:
- Image Captioning
- Visual Question Answering
- Document Analysis
- Audio-Text Transcription
Implementation Example
// Example: Using ML Task Runner for sentiment analysis
async function analyzeFeedbackSentiment(customerFeedback) {
// Run sentiment analysis on customer feedback
const sentimentResult = await mlTaskRunnerComponent.execute({
mlTask: "Natural Language Processing",
selectTask: "Sentiment Analysis",
toolInput: customerFeedback
});
// Process the results
const sentiment = sentimentResult.toolResult;
// Categorize feedback based on sentiment score
let feedbackCategory;
if (sentiment.score > 0.6) {
feedbackCategory = "Positive";
} else if (sentiment.score < 0.4) {
feedbackCategory = "Negative";
} else {
feedbackCategory = "Neutral";
}
// Return the analysis result
return {
originalFeedback: customerFeedback,
sentimentCategory: feedbackCategory,
sentimentScore: sentiment.score,
sentimentConfidence: sentiment.confidence,
analysisTimestamp: new Date().toISOString()
};
}
// Example: Using ML Task Runner for image classification
async function categorizeProductImage(imageUrl) {
// Run image classification on product image
const classificationResult = await mlTaskRunnerComponent.execute({
mlTask: "Computer Vision",
selectTask: "Image Classification",
toolInput: imageUrl
});
// Extract classification labels and scores
const classifications = classificationResult.toolResult;
// Return the top 3 category predictions
return {
imageUrl: imageUrl,
topCategories: classifications.slice(0, 3).map(c => ({
category: c.label,
confidence: c.score
})),
processedAt: new Date().toISOString()
};
}
Use Cases
- Content Moderation: Automatically detect and filter inappropriate content
- Customer Feedback Analysis: Process and categorize customer reviews and feedback
- Data Enrichment: Extract insights and metadata from unstructured content
- Document Processing: Extract information from documents and forms
- Content Recommendation: Generate personalized content recommendations
- Predictive Analytics: Forecast trends and patterns in business data
Best Practices
- Ensure input data matches the expected format for the selected ML task
- Consider pre-processing data before sending it to ML models
- Use appropriate task-specific models for better accuracy
- Implement error handling for cases where ML models might fail
- Consider resource requirements when choosing complex ML tasks
- Validate ML outputs before using them in critical applications
- Monitor model performance and results for potential drift or degradation
- Use confidence scores to filter low-confidence predictions when appropriate