Merge Data
The Merge Data component combines multiple data inputs into a single output, with support for various merge operations and data types.
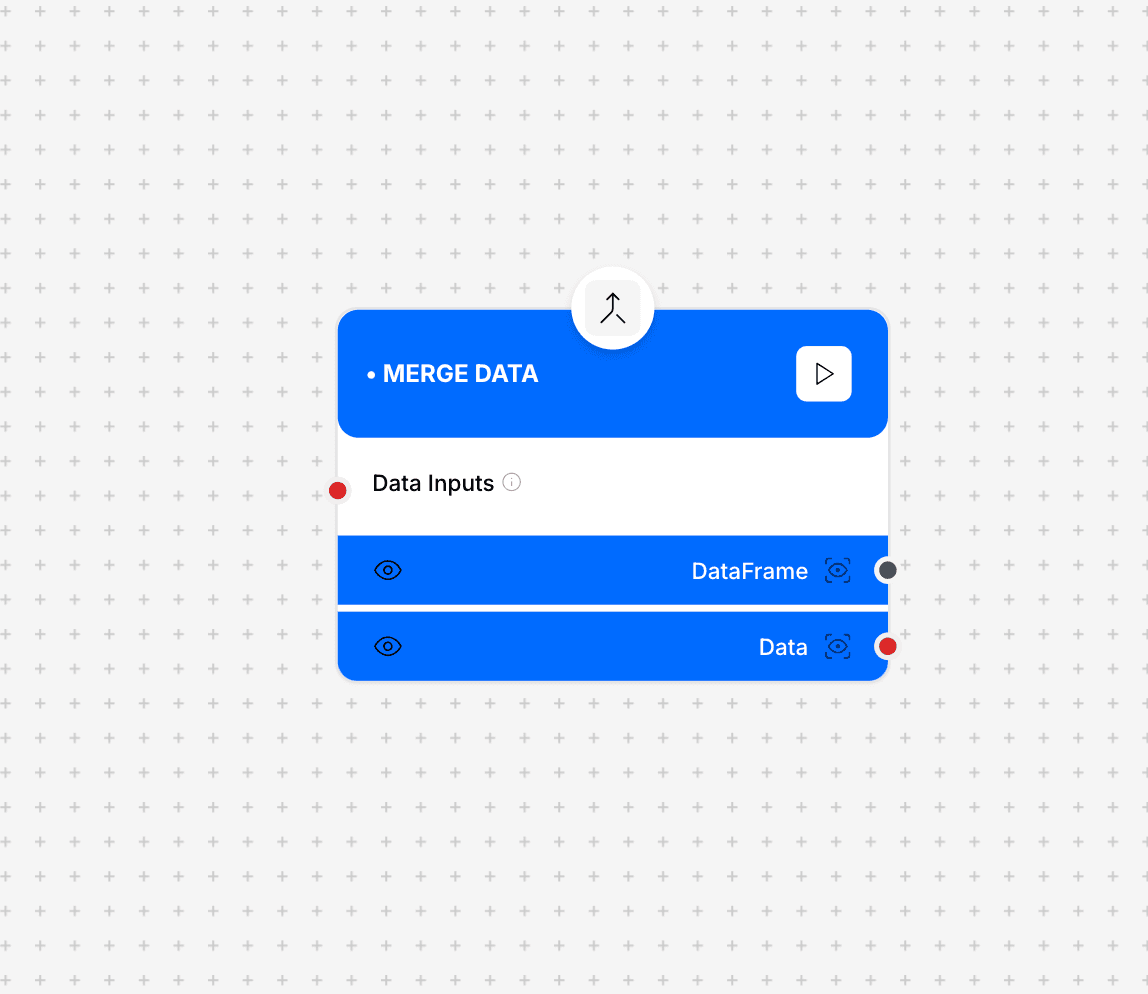
Merge Data interface and configuration
Component Inputs
- Data Inputs: Multiple data sources
The data to be merged
- Merge Operation: concatenate
The type of merge operation to perform
Component Outputs
- DataFrame: Combined data output
The resulting merged DataFrame
- Data: Alternative data output
The merged data in specified format
Implementation Example
const mergeData = {
dataInputs: [
{ id: 1, value: "First dataset" },
{ id: 2, value: "Second dataset" }
],
mergeOperation: "concatenate"
};
// Output:
// {
// dataFrame: [
// { id: 1, value: "First dataset" },
// { id: 2, value: "Second dataset" }
// ]
// }
Additional Resources
Best Practices
- Ensure data types are compatible before merging
- Choose appropriate merge operation for your use case
- Handle duplicate data appropriately
- Validate merged output for consistency