Chat Components
The Chat Components provide a comprehensive solution for handling both message input and output in chat interfaces. These components work together to create a seamless chat experience with customizable styling and behavior.
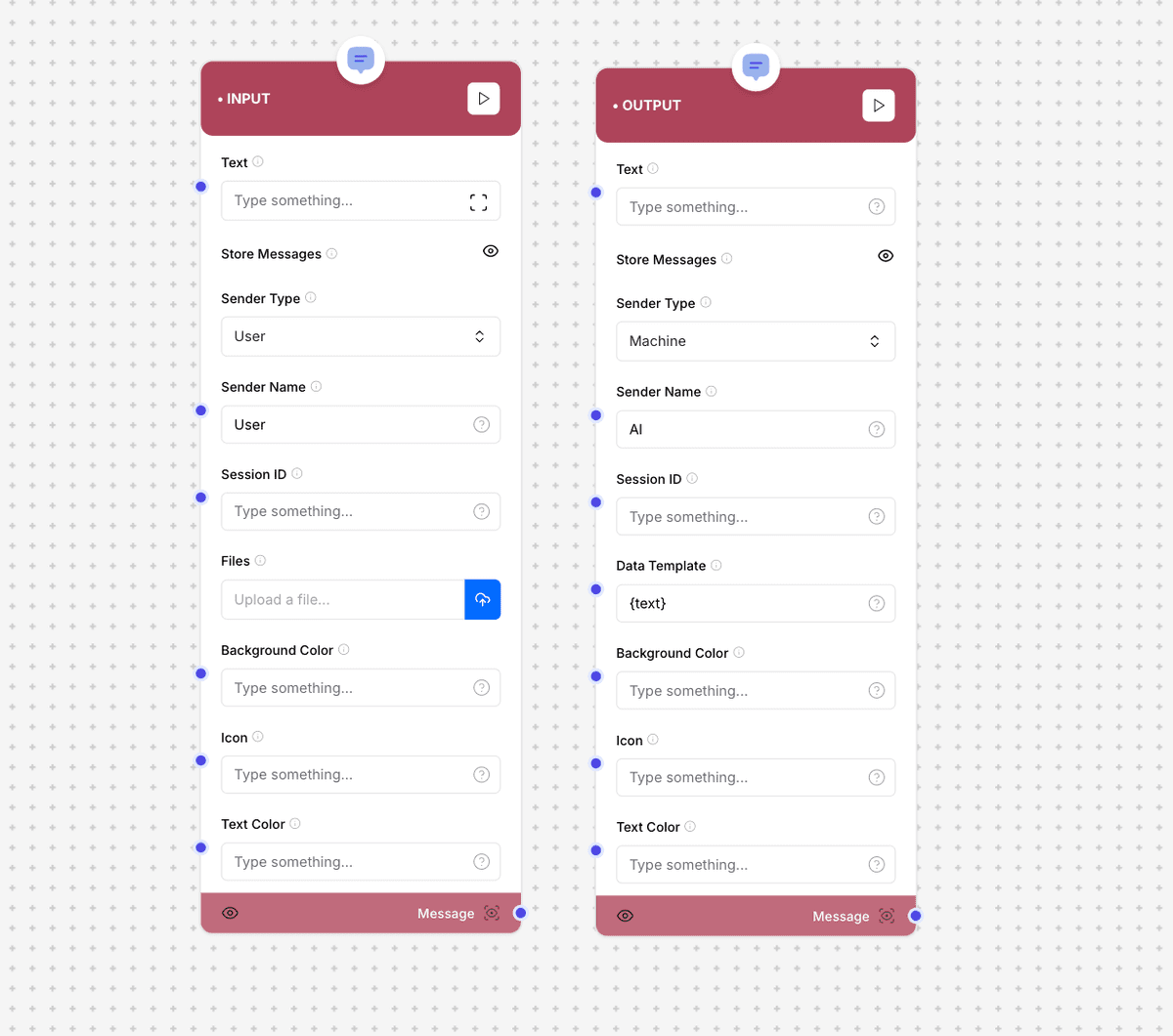
Chat Components interaction flow
Input Component
Input Props
- inputText: Current input text value
- storeMessages: Message storage function
- senderName: Name of message sender
- sessionId: Unique session identifier
- files: Array of attached files (optional)
- backgroundColor: Component background color (optional)
- icon: Custom icon component (optional)
- textColor: Text color (optional)
Input Component Output
{ "message": { "text": string, "sender": string, "sessionId": string, "timestamp": number, "files": Array<{ "name": string, "type": string, "size": number, "url": string }>, "metadata": { "device": string, "location": string, "userAgent": string } } }
Output Component
Output Props
- inputText: Message text content
- storeMessage: Message storage function
- senderType: Type of message sender
- senderName: Name of message sender
- sessionId: Unique session identifier
- dataTemplate: Custom message template (optional)
- backgroundColor: Message background color (optional)
- icon: Custom sender icon (optional)
- textColor: Message text color (optional)
Output Component Output
{ "message": { "text": string, "sender": { "type": string, "name": string }, "sessionId": string, "timestamp": number, "template": { "type": string, "data": object }, "styling": { "backgroundColor": string, "textColor": string, "icon": ReactNode }, "metadata": { "renderTime": number, "templateVersion": string } } }
Features
- Text input and output handling
- File attachments
- Message storage and retrieval
- Session management
- Custom styling options
- Template support
- Icon customization
- Error handling
Note: Ensure consistent styling between input and output components. Implement proper error handling for both components.
Tip: Use shared styling constants for consistent appearance. Implement message sanitization for security.
Example Usage
// Input Component <ChatInput inputText={currentText} storeMessages={handleMessageStore} senderName="John Doe" sessionId="session-123" files={attachedFiles} backgroundColor="#f0f0f0" icon={<CustomIcon />} textColor="#333333" /> // Output Component <ChatOutput inputText="Hello, world!" storeMessage={handleMessageStore} senderType="user" senderName="John Doe" sessionId="session-123" dataTemplate={customTemplate} backgroundColor="#ffffff" icon={<UserIcon />} textColor="#000000" />