CSV Agent
The CSV Agent is a specialized component that processes, analyzes, and manipulates CSV data files with intelligent parsing, data cleaning, and transformation capabilities.
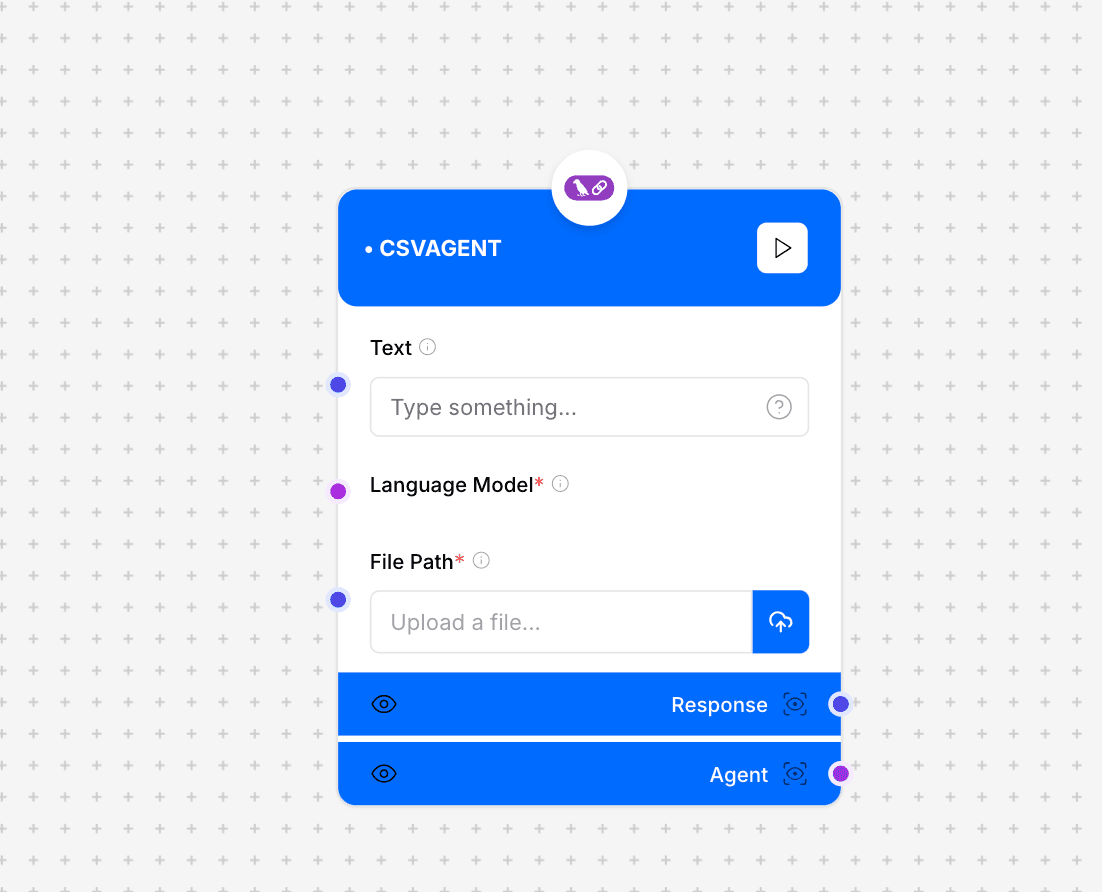
CSV Agent interface and configuration options
Configuration Parameters
Required Parameters
- File Path: Path to CSV file
- Language Model: The AI model for processing
- Agent Type: Type of analysis
- Delimiter: CSV field separator
- Encoding: File encoding format
Optional Configuration
- Parse Options: CSV parsing settings
- skipRows: Number of rows to skip
- headerRow: Header row index
- skipEmptyLines: Skip empty lines
- Data Cleaning: Cleaning options
- removeNulls: Remove null values
- trimWhitespace: Trim whitespace
- convertTypes: Auto-convert types
- Memory Management: Memory options
- chunkSize: Processing chunk size
- maxMemory: Maximum memory usage
Output Format
{ "result": { "data": array, "analysis": { "rowCount": number, "columnCount": number, "summary": object }, "metadata": { "processingTime": string, "memoryUsed": string, "transformations": array } } }
Example Usage
const csvAgent = new CSVAgent({ filePath: "data/sales_records.csv", languageModel: "gpt-4", agentType: "data-analysis", delimiter: ",", encoding: "utf-8", parseOptions: { skipRows: 0, headerRow: 0, skipEmptyLines: true }, dataCleaning: { removeNulls: true, trimWhitespace: true, convertTypes: true }, memoryManagement: { chunkSize: 1000, maxMemory: "1GB" } }); const result = await csvAgent.process({ input: "Analyze sales trends by region" });
Additional Resources
Best Practices
- Validate CSV format before processing
- Use appropriate encoding settings
- Implement memory-efficient processing
- Handle missing or invalid data
- Monitor processing performance