JSON Key Extractor Component
The JSON Key Extractor component provides precise value extraction from JSON structures using key paths. It supports nested object traversal, array access, and strict validation while maintaining type safety and error handling.
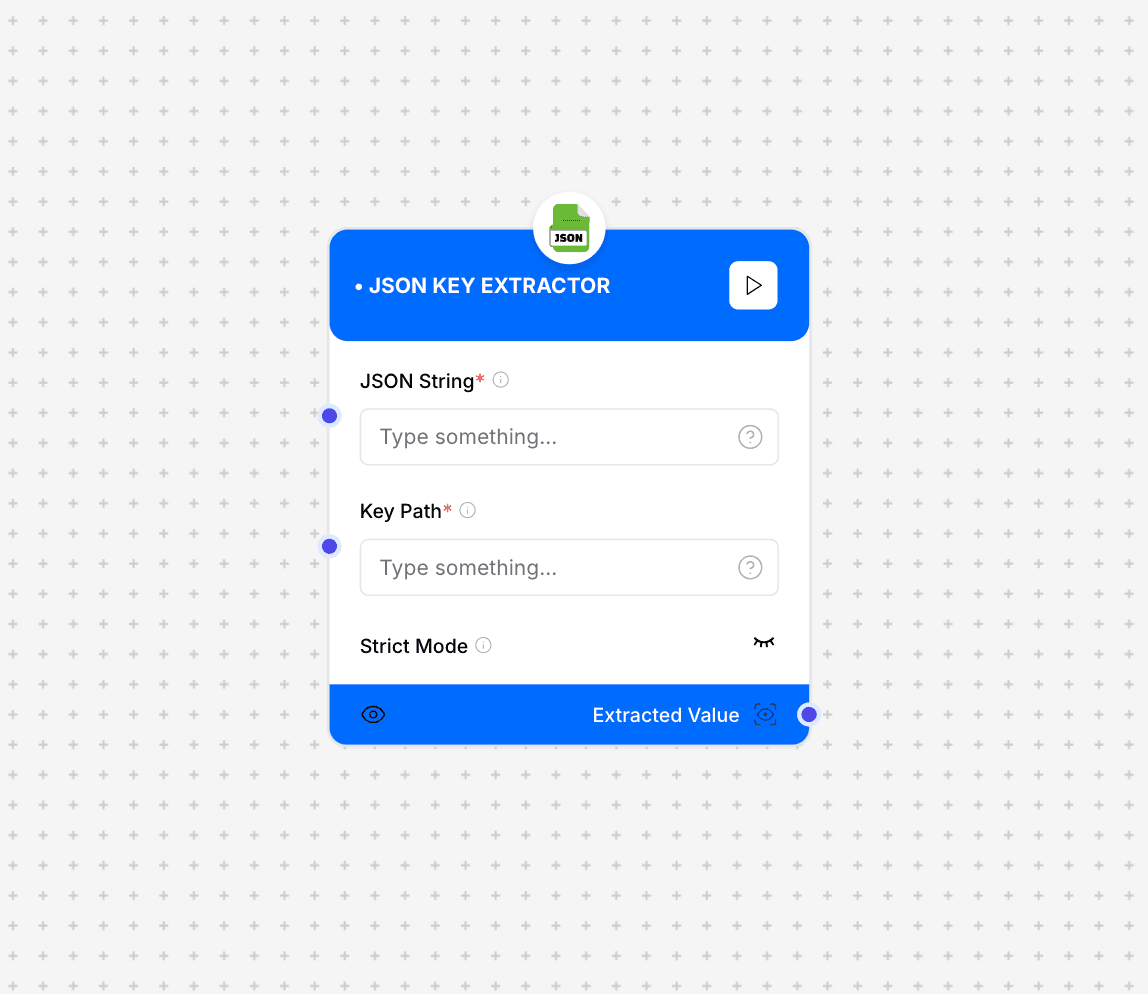
JSON Key Extractor workflow and architecture
Configuration Parameters
Required Parameters
- jsonString: Input JSON string to parse
- keyPath: Path to desired value
- Dot notation: "user.address.city"
- Array access: "users[0].name"
- Mixed path: "data.items[2].details.id"
Optional Parameters
- strictMode: Enable strict validation (default: false)
- Type checking
- Path validation
- Null checking
Output Format
{ "extracted_value": any, // The extracted value of any valid JSON type "metadata": { "path": string, "value_type": string, "depth": number, "extraction_time": number }, "validation": { "strict_mode": boolean, "type_check_passed": boolean, "path_valid": boolean, "value_present": boolean }, "error": { "code": string, "message": string, "details": { "path_segment": string, "expected_type": string, "actual_type": string } } | null }
Features
- Nested object traversal
- Array access support
- Type validation
- Strict mode validation
- Error handling
- Performance metrics
- Path validation
- Null safety
Note: Enable strict mode for sensitive data extraction. Handle potential null values appropriately.
Tip: Use dot notation for better readability. Implement error handling for missing paths.
Example Usage
const extractor = new JsonKeyExtractor(); // Simple key extraction const result1 = await extractor.extract({ jsonString: '{"user": {"name": "John", "age": 30}}', keyPath: "user.name", strictMode: false }); // Array access with strict mode const result2 = await extractor.extract({ jsonString: `{ "products": [ {"id": 1, "details": {"price": 29.99}}, {"id": 2, "details": {"price": 39.99}} ] }`, keyPath: "products[1].details.price", strictMode: true }); // Complex nested path const result3 = await extractor.extract({ jsonString: `{ "data": { "items": [ { "category": { "subcategories": [ {"name": "Electronics", "id": "e001"} ] } } ] } }`, keyPath: "data.items[0].category.subcategories[0].id", strictMode: true });
Key Path Patterns:
// Simple path "user.name" // Array access "users[0].address" // Multiple array access "data.items[0].subitems[2].id" // Mixed notation "organization.departments[0].employees[1].contact.phone"