Structured Output
The Structured Output component transforms unstructured data into organized, structured formats, with support for various output schemas and data validation.
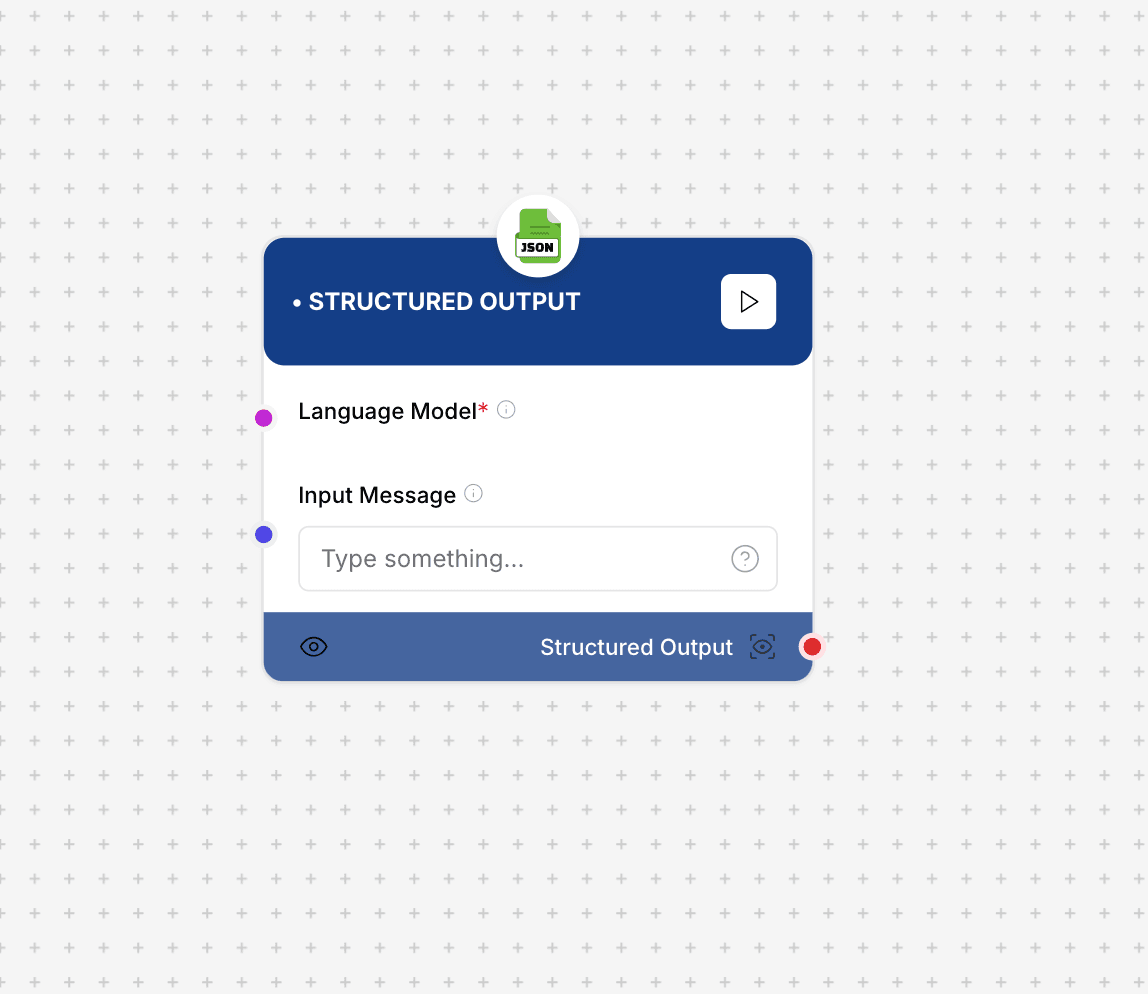
Structured Output interface and configuration
Component Inputs
- Input Data: Raw data input
The unstructured data to process
- Output Schema: Structure definition
The desired output format
- Validation Rules: Data validation config
Rules for validating the output
- Transform Options: Processing settings
Options for data transformation
Component Outputs
- Structured Data: Formatted output
The structured data result
- Validation Report: Validation results
Report of validation checks
Implementation Example
const structuredOutput = {
inputData: "John Doe, 30, john@example.com",
outputSchema: {
type: "object",
properties: {
name: { type: "string" },
age: { type: "number" },
email: { type: "string", format: "email" }
}
},
validationRules: {
age: { min: 0, max: 120 },
email: { pattern: "^[^@]+@[^@]+\.[^@]+$" }
}
};
// Output:
// {
// structuredData: {
// name: "John Doe",
// age: 30,
// email: "john@example.com"
// },
// validationReport: {
// valid: true,
// errors: []
// }
// }
Additional Resources
Best Practices
- Define clear and comprehensive output schemas
- Implement thorough validation rules
- Handle edge cases and invalid data gracefully
- Document schema changes and versioning