Hierarchical Crew
A component that allows you to organize and orchestrate multiple AI agents into a collaborative team. The Hierarchical Crew component enables complex, multi-step workflows with specialized agents working together toward a common goal.
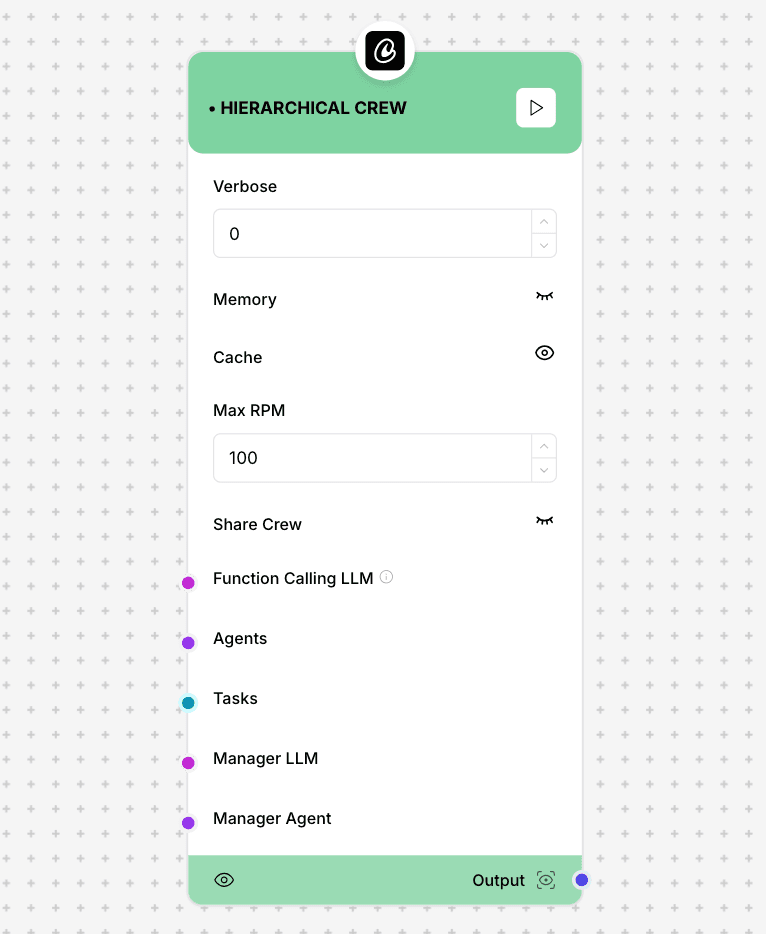
Hierarchical Crew component interface and configuration
Advanced Configuration: This component requires careful planning of agent roles and responsibilities. Proper configuration of memory sharing and agent interactions is crucial for effective collaboration.
Component Inputs
- Verbose: Level of detail in crew operation logs
Example: 0 (minimal logging) to 2 (detailed logging of all agent interactions)
- Memory: Shared memory configuration for the crew
Example: "Shared" (all agents access the same memory) or "Individual" (each agent has private memory)
- Cache: Whether to cache agent responses
Example: Enabled (for faster repeated operations) or Disabled (for always fresh responses)
- Max RPM: Maximum requests per minute for the crew
Example: 100 (limits the rate of API calls to prevent throttling)
- Share Crew: Whether agents can reference other agents in the crew
Example: Enabled (agents can collaborate directly) or Disabled (agents work independently)
Component Outputs
- Toolset: The result of the crew's collaborative work
Example: The final output from the orchestrated workflow, which could be a document, analysis, or other collaborative result
Agent Types and Roles
Function Calling LLM
An agent that can execute specific functions and interact with external tools
Role: Primary interface for tool interactions and function execution
Example: API caller, data processor, or query executor
Agents
Specialized agents with specific skills or knowledge domains
Role: Domain-specific experts that handle specialized tasks
Example: Research agent, creative writer, code reviewer
Tasks
Discrete work items that agents can perform
Role: Structured units of work assigned to agents
Example: Data analysis task, content generation task, evaluation task
Manager LLM
Oversees the workflow and coordinates agent activities
Role: Orchestrator that assigns tasks and monitors progress
Example: Project manager, workflow coordinator
Manager Agent
Assistant to the manager that handles specific coordination aspects
Role: Task dispatcher and progress tracker
Example: Quality assurance, timeline manager
Implementation Example
// Example configuration for a content creation crew
const contentCreationCrew = {
verbose: 1,
memory: "Shared",
cache: true,
maxRpm: 60,
shareCrew: true,
agents: [
{
role: "Manager",
type: "ManagerLLM",
model: "gpt-4",
description: "Coordinates the content creation workflow and ensures quality"
},
{
role: "Researcher",
type: "Agent",
model: "gpt-4",
description: "Gathers relevant information on the topic"
},
{
role: "Writer",
type: "Agent",
model: "gpt-4",
description: "Creates engaging content based on research"
},
{
role: "Editor",
type: "Agent",
model: "gpt-4",
description: "Reviews and refines the content for clarity and accuracy"
}
],
workflow: [
{
name: "Research",
type: "Task",
assignedTo: "Researcher",
description: "Collect key information and data points on the topic"
},
{
name: "Draft",
type: "Task",
assignedTo: "Writer",
description: "Create a compelling first draft based on research findings"
},
{
name: "Edit",
type: "Task",
assignedTo: "Editor",
description: "Review and refine the draft for quality and accuracy"
},
{
name: "Finalize",
type: "Task",
assignedTo: "Manager",
description: "Approve the final content and prepare for delivery"
}
]
};
// Example integration in an application
async function createContent(topic, audience, format) {
// Initialize the crew component
const crewResult = await hierarchicalCrewComponent.execute({
verbose: contentCreationCrew.verbose,
memory: contentCreationCrew.memory,
cache: contentCreationCrew.cache,
maxRpm: contentCreationCrew.maxRpm,
shareCrew: contentCreationCrew.shareCrew,
context: {
topic: topic,
audience: audience,
format: format,
deadline: new Date().addDays(1).toISOString()
}
});
// Extract and use the crew's output
return {
content: crewResult.toolset,
metadata: {
created: new Date().toISOString(),
topic: topic,
audience: audience
}
};
}
Use Cases
- Content Creation: Orchestrate research, writing, and editing agents
- Product Development: Coordinate design, development, and testing workflows
- Customer Support: Manage ticket classification, research, and response generation
- Market Analysis: Combine data collection, processing, and insight generation
- Research Projects: Coordinate literature review, analysis, and reporting
Best Practices
- Clearly define each agent's role and responsibilities
- Start with a simpler crew structure and expand gradually
- Use shared memory for tasks requiring context preservation
- Set appropriate verbosity levels for debugging and monitoring
- Design workflows with clear dependencies and handoffs
- Implement error handling and recovery mechanisms
- Monitor resource usage to prevent excessive API consumption
- Test crews with various inputs to ensure robust performance