OpenAI Tools Agent
The OpenAI Tools Agent is a specialized component that leverages OpenAI's function calling capabilities to execute various tools and functions through natural language.
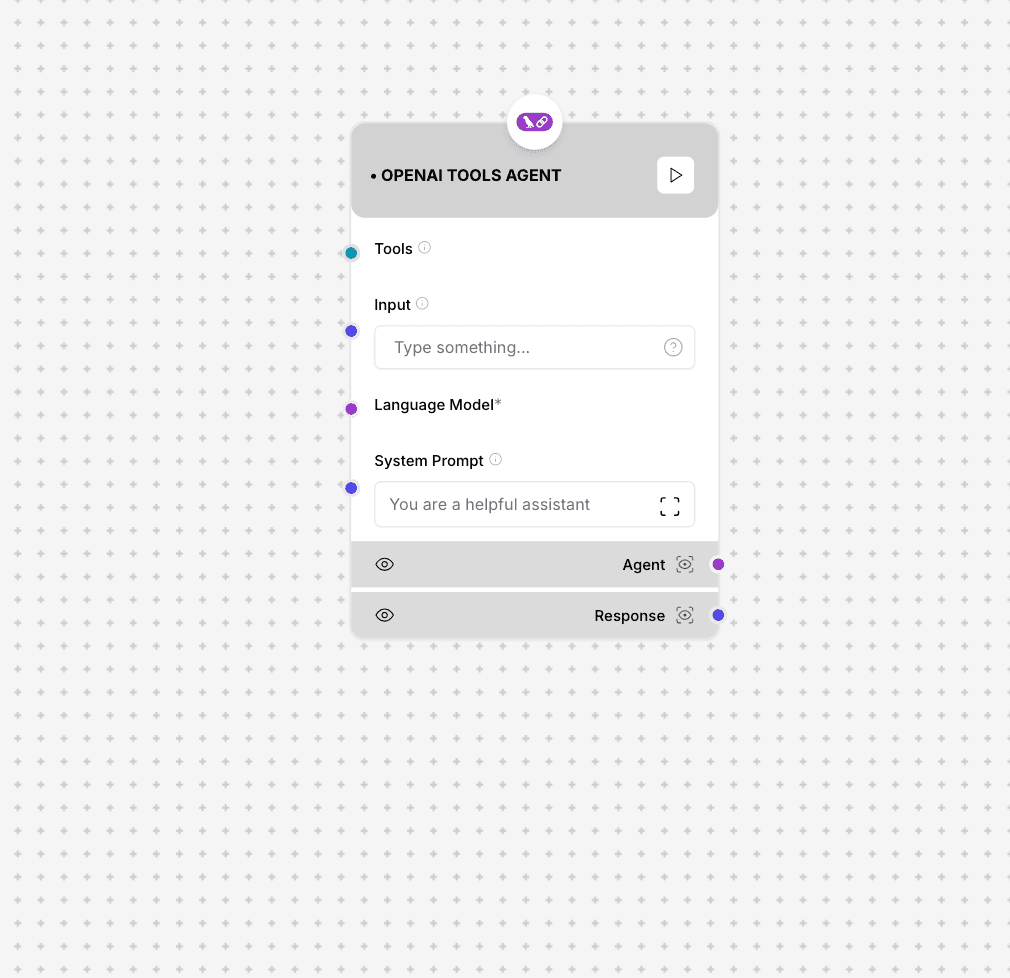
OpenAI Tools Agent interface and configuration options
Configuration Parameters
Required Parameters
- Tool: Available tools configuration
- Web Search: Web search capability
- Agent Description: Agent behavior description
- Language Model: OpenAI model configuration
- System Prompt: Base prompt for the agent
Output Format
{ "agentId": string, "toolsUsed": array, "response": { "result": any, "metadata": { "processingTime": string, "toolCalls": number } } }
Example Usage
const openaiToolsAgent = new OpenAIToolsAgent({ tool: [ { name: "calculator", description: "Perform mathematical calculations", parameters: { expression: "string" } }, { name: "weather", description: "Get weather information", parameters: { location: "string", unit: "celsius|fahrenheit" } } ], webSearch: { enabled: true, maxResults: 5 }, agentDescription: "An AI assistant that can use various tools", languageModel: "gpt-4", systemPrompt: "You are a helpful assistant that can use tools..." }); const result = await openaiToolsAgent.process({ input: "What's the temperature in London and convert it to Fahrenheit?" });
Additional Resources
Best Practices
- Define clear tool specifications
- Handle tool errors gracefully
- Implement rate limiting
- Monitor tool usage
- Validate tool inputs