XML Agent
The XML Agent is a specialized component that processes and manipulates XML data with natural language understanding and advanced validation capabilities.
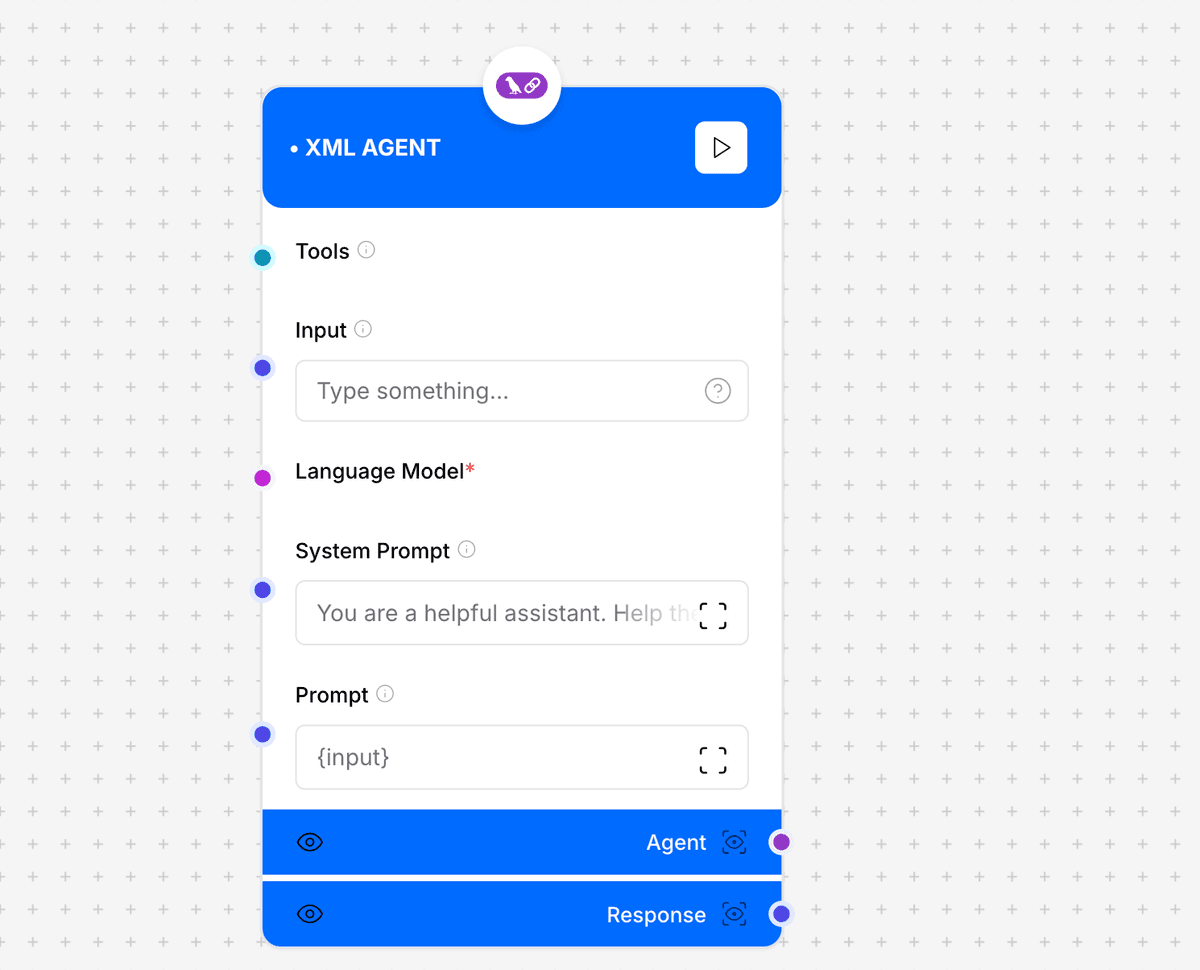
XML Agent interface and configuration options
Configuration Parameters
Required Parameters
- Tool: XML processing capabilities
- Handle Parse Errors: Error handling
- Web Search: Web search capability
- Agent Description: Agent behavior
- Language Model: The AI model
- Chat History: Conversation context
- System Prompt: Base prompt
- Prompt: Processing instructions
Output Format
{ "response": { "result": string, "metadata": { "processingTime": string, "validationStatus": string, "schemaCompliance": boolean } }, "agentId": string }
Example Usage
const xmlAgent = new XMLAgent({ tool: "xml-processor", handleParseErrors: true, webSearch: { enabled: true, maxResults: 5 }, agentDescription: "XML processing and validation agent", languageModel: "gpt-4", chatHistory: [], systemPrompt: "Process and validate XML data...", prompt: "Transform the following data into valid XML..." }); const result = await xmlAgent.process({ input: "Convert this data structure to XML format" });
Additional Resources
Best Practices
- Validate XML schema
- Handle namespaces properly
- Implement error recovery
- Monitor processing time
- Use appropriate encoding