Sequential Task
A component that executes a series of operations in a defined order. The Sequential Task component enables you to create linear workflows where each step builds upon the previous ones.
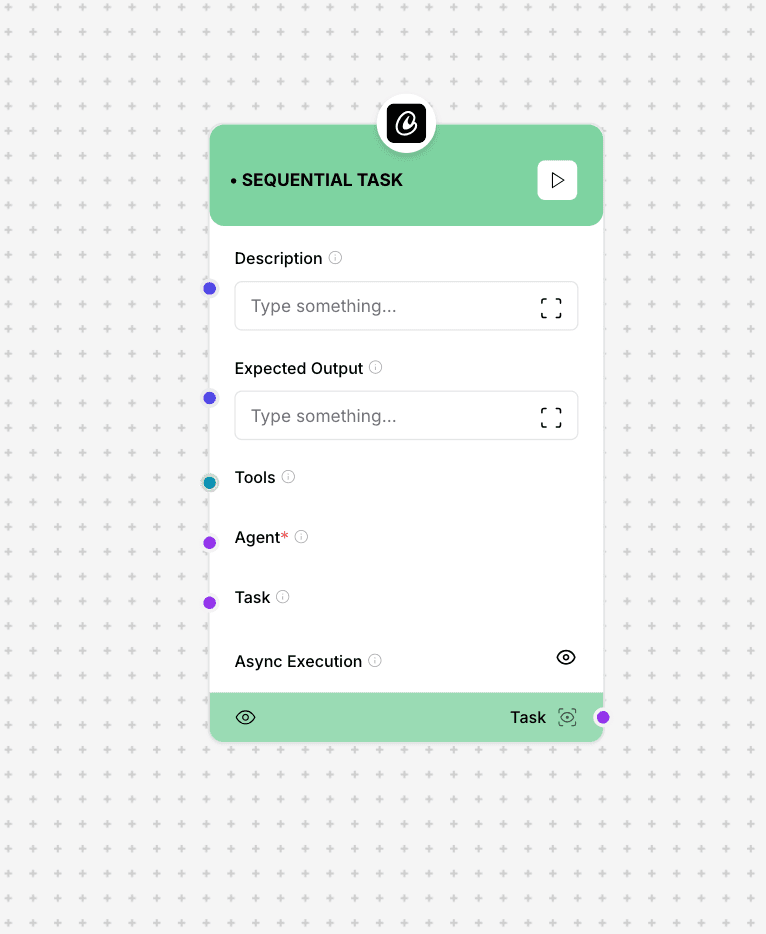
Sequential Task component interface and configuration
Execution Flow: Sequential Tasks execute steps in the exact order defined. If any step fails, the entire task will fail unless error handling is explicitly configured. Ensure proper input validation and error handling for robust workflows.
Component Inputs
- Description: Detailed explanation of what the task should accomplish
Example: "Process customer feedback data, analyze sentiment, and generate response templates."
- Expected Output: Format specification for the task's output
Example: "JSON object containing processed feedback, sentiment scores, and recommended response templates."
- Tools: List of tools the task can use
Example: ["TextProcessor", "SentimentAnalyzer", "TemplateGenerator"]
- Agents: AI agents that can be used in the task
Example: ["DataAnalyst", "ContentCreator"]
Component Outputs
- Task: The result of the executed sequential task
Example: The complete output from all steps in the sequence, formatted according to the expected output specification
Configuration Options
Async Execution
Controls whether the task runs asynchronously
Options: Enabled/Disabled
Default: Disabled (synchronous execution)
Use Case: Enable for long-running tasks that shouldn't block the main workflow
Toolset Configuration
Configure tools available to the task
Settings: Tool selection, access permissions, resource limits
Use Case: Fine-tune available capabilities for the task sequence
Task Steps
The discrete operations in the sequence
Structure: Ordered array of step definitions
Each step includes: name, description, tool/agent to use, input/output mapping
Implementation Example
// Example: Content generation workflow
async function generateBlogContent(topic, targetAudience, length) {
// Configure the sequential task
const contentWorkflow = {
description: `Generate a blog post about ${topic} for ${targetAudience} audience with approximate length of ${length} words.`,
expectedOutput: "JSON object with title, outline, content sections, and SEO metadata",
tools: ["ResearchTool", "ContentGenerator", "SEOAnalyzer"],
agents: ["Researcher", "Writer", "Editor"],
steps: [
{
name: "Research",
description: "Gather relevant information about the topic",
tool: "ResearchTool",
agent: "Researcher",
inputs: { topic, depth: "medium" }
},
{
name: "Outline",
description: "Create a structured outline based on research",
agent: "Writer",
inputs: { researchResults: "{{Research.output}}", audience: targetAudience }
},
{
name: "Content",
description: "Write the full content following the outline",
agent: "Writer",
inputs: {
outline: "{{Outline.output}}",
research: "{{Research.output}}",
wordCount: length
}
},
{
name: "Edit",
description: "Review and refine the content",
agent: "Editor",
inputs: { draft: "{{Content.output}}" }
},
{
name: "SEO",
description: "Generate SEO metadata for the content",
tool: "SEOAnalyzer",
inputs: { content: "{{Edit.output}}", keywords: "{{Research.keywords}}" }
}
]
};
// Execute the sequential task
const result = await sequentialTaskComponent.execute({
description: contentWorkflow.description,
expectedOutput: contentWorkflow.expectedOutput,
tools: contentWorkflow.tools,
agents: contentWorkflow.agents,
asyncExecution: false,
toolsetConfiguration: {
steps: contentWorkflow.steps
}
});
// Return the generated content
return result.task;
}
Use Cases
- Content Creation: Multi-stage content generation with research, writing, and editing phases
- Data Processing: Extract, transform, and load data through sequential operations
- Customer Engagement: Process customer input, analyze intent, and generate appropriate responses
- Report Generation: Collect data, analyze metrics, and compile formatted reports
- Document Processing: Extract information, validate, transform, and store document data
Best Practices
- Define clear input and output requirements for each step
- Use variable references to pass data between steps
- Keep each step focused on a single responsibility
- Implement error handling for critical steps
- Consider using async execution for long-running sequences
- Test workflows with various inputs to ensure robustness
- Monitor execution times and resource usage
- Document the purpose and dependencies of each step