Universal Agent
The Universal Agent provides a flexible interface for interacting with various AI models across different providers. It supports multiple model providers, custom instructions, and tool integration while maintaining a consistent interface for all interactions.
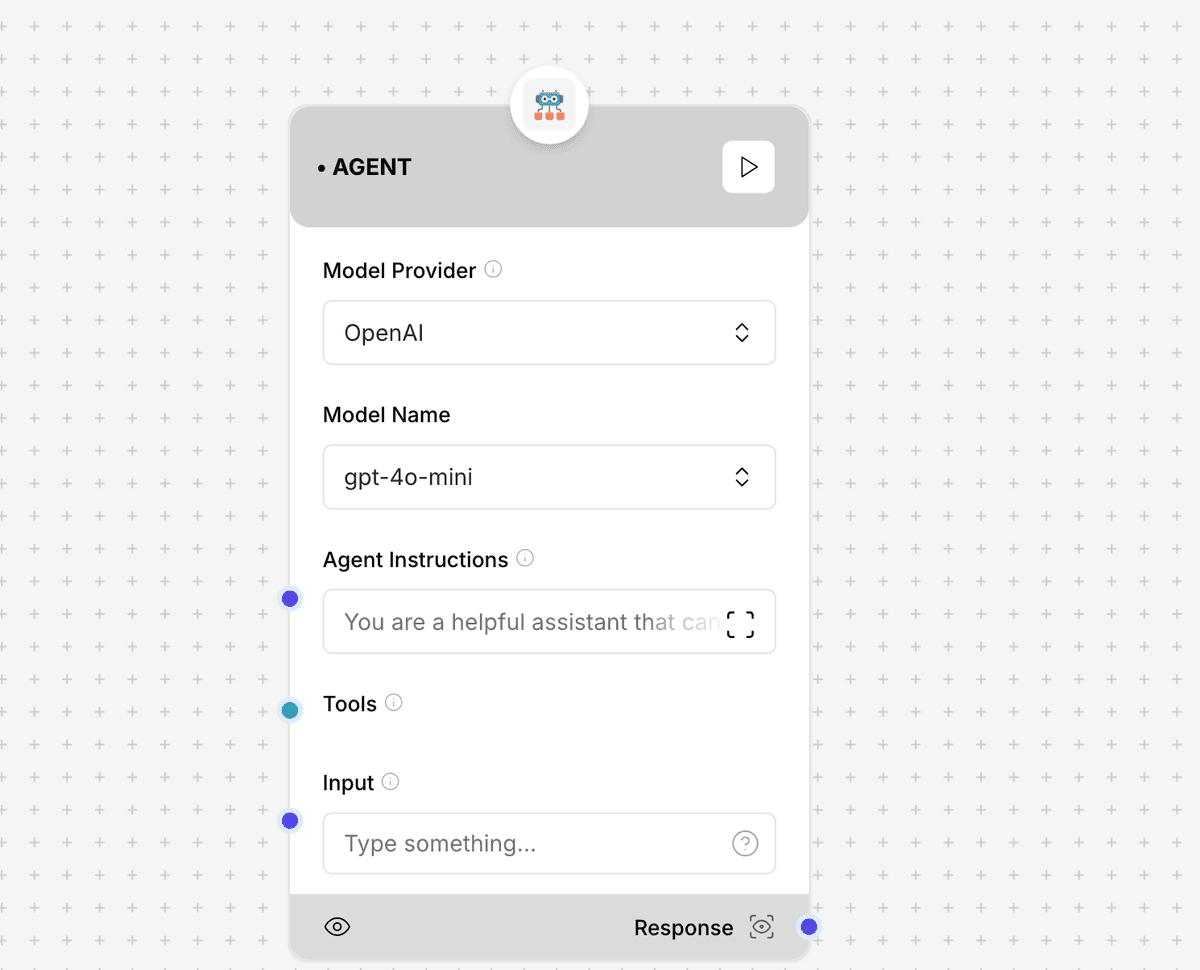
Universal Agent workflow and architecture
Configuration Parameters
Model Provider Options
- Amazon Bedrock
- Claude
- Titan
- Jurassic-2
- Anthropic
- Claude 3 Opus
- Claude 3 Sonnet
- Claude 3 Haiku
- Azure OpenAI
- GPT-4
- GPT-3.5-Turbo
- Groq
- LLaMA2 70B
- Mixtral 8x7B
- OpenAI
- GPT-4
- GPT-3.5-Turbo
- Custom
- User-defined model endpoints
- Custom model implementations
Required Input Parameters
- model_provider: Selected AI model provider
- model_name: Specific model to use
- input: User query or instruction
Optional Configuration
- agent_instructions: Custom behavior instructions
- tools: Array of available tools
- name: Tool identifier
- description: Tool functionality
- parameters: Required inputs
- handler: Function implementation
- model_params: Model-specific parameters
- temperature: Response creativity
- max_tokens: Response length
- top_p: Nucleus sampling
Output Format
{ "response": { "content": string, "role": string, "tool_calls": [ { "tool": string, "input": object, "output": any } ] }, "metadata": { "model_info": { "provider": string, "model": string, "version": string }, "usage": { "prompt_tokens": number, "completion_tokens": number, "total_tokens": number }, "latency": number } }
Features
- Multi-provider support
- Custom tool integration
- Flexible instruction setting
- Error handling and retries
- Usage tracking
- Performance monitoring
- Model fallback options
Note: Each model provider may have specific authentication requirements and rate limits. Ensure proper API keys and credentials are configured for your selected provider.
Tip: Use clear and specific agent instructions to optimize model behavior. Consider implementing model fallback strategies for improved reliability.
Example Usage
const agent = new Agent({ model_provider: "anthropic", model_name: "claude-3-opus", agent_instructions: "You are a helpful assistant...", tools: [ { name: "calculator", description: "Perform mathematical calculations", parameters: { expression: "string" } } ] }); const response = await agent.run({ input: "Calculate the compound interest on $1000 at 5% for 3 years" });