OpenAPI Agent
The OpenAPI Agent is a specialized component that processes OpenAPI specifications to enable intelligent API interaction, documentation generation, and validation capabilities.
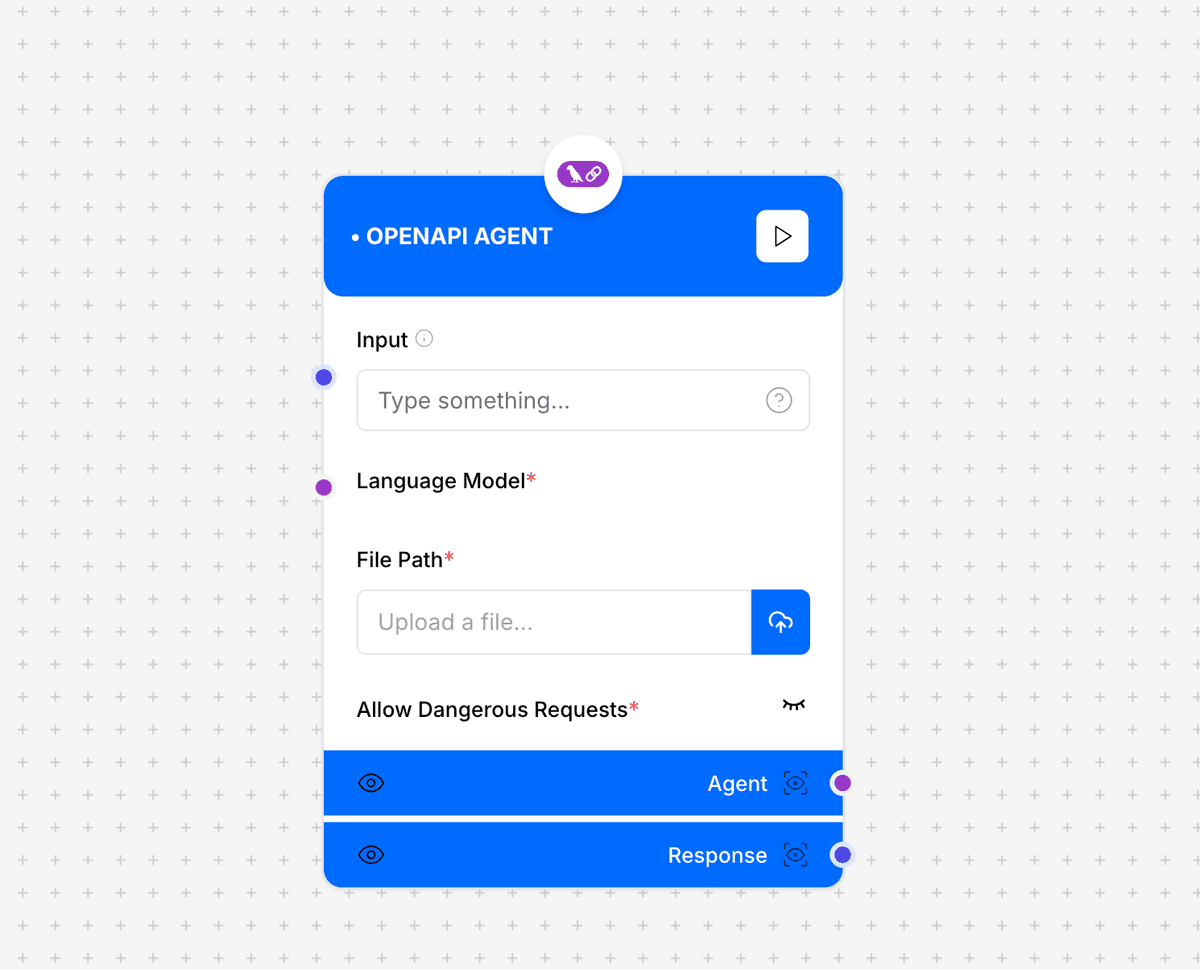
OpenAPI Agent interface and configuration options
Configuration Parameters
Required Parameters
- Language Model: The AI model for processing
- Specification: OpenAPI specification source
- Version: OpenAPI specification version
- Base URL: API base URL
- Authentication: API authentication details
Optional Configuration
- Validation: Validation options
- strict: Strict validation mode
- formats: Custom formats
- schemas: Custom schemas
- Documentation: Documentation settings
- format: Output format
- template: Custom template
- examples: Include examples
- Security: Security options
- scopes: Security scopes
- tokens: API tokens
Output Format
{ "result": { "specification": { "parsed": object, "validated": boolean, "errors": array }, "documentation": { "content": string, "format": string, "metadata": object }, "validation": { "status": string, "issues": array, "suggestions": array } } }
Example Usage
const openAPIAgent = new OpenAPIAgent({ languageModel: "gpt-4", specification: { source: "https://api.example.com/openapi.json", type: "url" }, version: "3.0.0", baseUrl: "https://api.example.com", authentication: { type: "oauth2", credentials: { clientId: process.env.CLIENT_ID, clientSecret: process.env.CLIENT_SECRET } }, validation: { strict: true, formats: { custom: /^custom-format$/ }, schemas: { CustomType: { type: "object", properties: {} } } }, documentation: { format: "markdown", template: "custom-template.md", examples: true }, security: { scopes: ["read", "write"], tokens: { access: process.env.ACCESS_TOKEN, refresh: process.env.REFRESH_TOKEN } } }); const result = await openAPIAgent.process({ input: "Generate API documentation and validate endpoints" });
Additional Resources
Best Practices
- Keep specifications up-to-date
- Use strict validation mode
- Include comprehensive examples
- Implement proper security
- Monitor API changes