DeepSeek Models
A drag-and-drop component for integrating DeepSeek's language models. Configure model parameters and connect inputs/outputs to leverage DeepSeek's advanced AI capabilities.
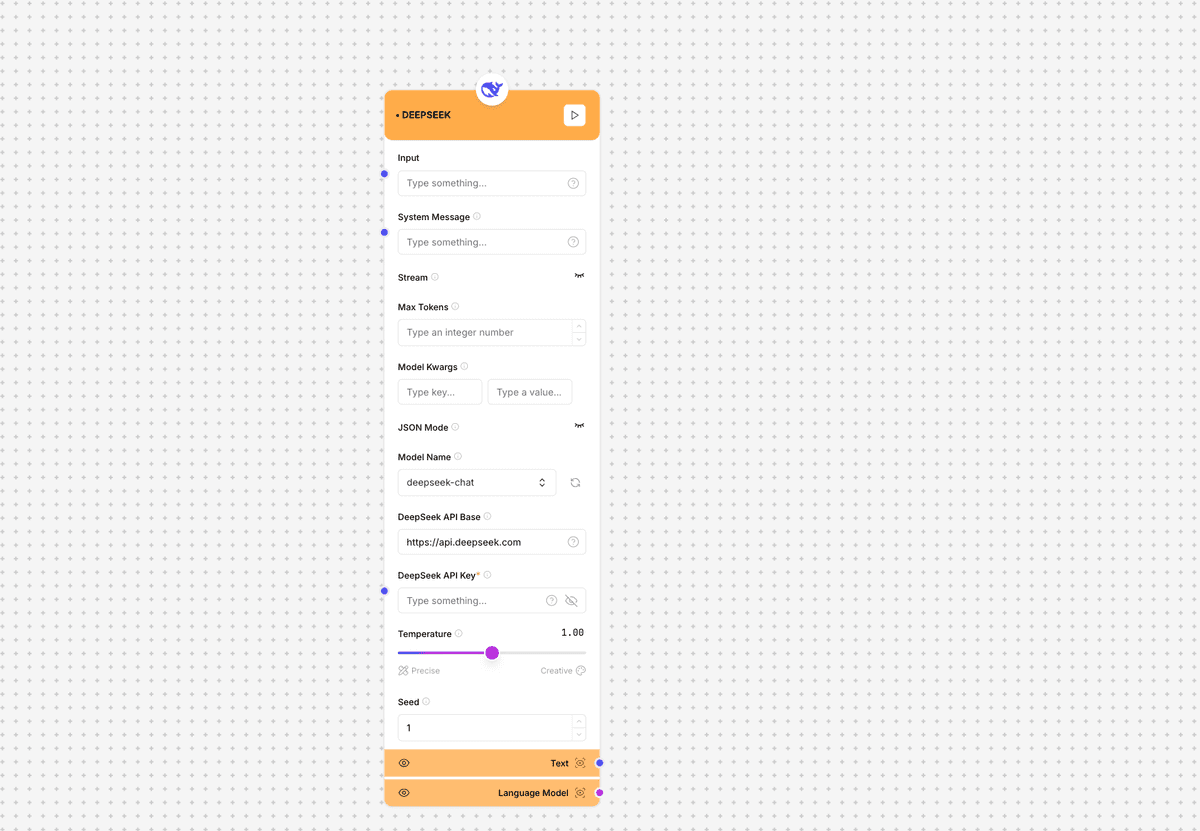
DeepSeek component interface and configuration
API Key Required: A valid DeepSeek API key is required to use this component. You'll need to register for access to DeepSeek's API platform and generate an API key before using this component.
Component Inputs
- Input: Text input for the model
Example: "Write a function in Python to implement a binary search algorithm."
- System Message: System prompt to guide model behavior
Example: "You are an AI assistant specialized in writing efficient and well-documented code."
- Stream: Toggle for streaming responses
Example: true (for real-time token streaming) or false (for complete response)
- Model Name: The DeepSeek model to use
Example: "deepseek-chat", "deepseek-coder"
- DeepSeek API Key: Your API authentication key
Example: "sk-deepseek-xxxx..."
- DeepSeek API Base: API endpoint URL
Example: "https://api.deepseek.com" (Default)
- JSON Mode: Toggle for JSON output format
Example: true (for structured JSON responses) or false (for free-form text)
Component Outputs
- Text: Generated text output
Example: "```python\ndef binary_search(arr, target):\n left, right = 0, len(arr) - 1\n \n while left = right:\n mid = (left + right) // 2\n \n if arr[mid] == target:\n return mid\n elif arr[mid] target:\n left = mid + 1\n else:\n right = mid - 1\n \n return -1\n```"
- Language Model: Model information and metadata
Example: model: deepseek-coder, usage: {prompt_tokens: 45, completion_tokens: 120, total_tokens: 165}
Generation Parameters
Max Tokens
Maximum number of tokens to generate in the response
Default: 4096
Range: 1 to model maximum
Recommendation: Set based on expected response length
Temperature
Controls randomness in the output - higher values increase creativity
Default: 1.0
Range: 0.0 to 2.0
Recommendation: Lower (0.1-0.3) for factual/code responses, Higher (0.7-1.0) for creative tasks
Seed
Random seed for reproducible outputs
Default: 1
Range: Any integer
Recommendation: Set specific values for reproducible results
Model Kwargs
Additional model parameters passed as key-value pairs
Examples:
- top_p: 0.9 (nucleus sampling parameter)
- frequency_penalty: 0.5 (reduces repetition)
- presence_penalty: 0.5 (encourages diversity)
- stop: ["\n\n"] (tokens at which to stop generation)
Available Models
DeepSeek Chat
General-purpose language models for conversational tasks
Models:
- deepseek-chat (7B parameters)
- deepseek-chat-v2 (latest version)
DeepSeek Coder
Specialized language models for programming tasks
Models:
- deepseek-coder (6.7B parameters)
- deepseek-coder-instruct (instruction-tuned)
Implementation Example
// Basic configuration
const deepSeekConfig = {
modelName: "deepseek-chat",
deepSeekApiKey: process.env.DEEPSEEK_API_KEY,
systemMessage: "You are a helpful assistant."
};
// Advanced configuration
const advancedDeepSeekConfig = {
modelName: "deepseek-coder",
deepSeekApiKey: process.env.DEEPSEEK_API_KEY,
deepSeekApiBase: "https://api.deepseek.com",
maxTokens: 2000,
temperature: 0.3,
seed: 42,
jsonMode: true,
stream: true,
modelKwargs: {
top_p: 0.95,
frequency_penalty: 0.2,
presence_penalty: 0.2,
stop: ["\n\n"]
}
};
// Usage example
async function generateCode(input) {
const response = await deepSeekComponent.generate({
input: input,
systemMessage: "You are an expert programmer. Write clean, well-documented code.",
modelName: "deepseek-coder",
temperature: 0.2
});
return response.text;
}
Use Cases
- Code Generation: Create code snippets, functions, and algorithms across multiple programming languages
- Technical Documentation: Generate clear explanations and documentation for technical concepts
- Conversational AI: Build chat interfaces and virtual assistants
- Data Transformation: Leverage JSON mode for structured data formatting
- Content Creation: Generate articles, blogs, and other written content
Best Practices
- Use JSON mode when you need structured, parseable outputs
- Enable streaming for better user experience with real-time responses
- Set a specific seed when you need reproducible results
- Adjust temperature based on task type (lower for precision, higher for creativity)
- Store API keys securely using environment variables
- Monitor your token usage for cost management
- Implement proper error handling for API failures
- Choose DeepSeek Coder specifically for programming tasks