Sequential Crew
The Sequential Crew orchestrates a series of specialized agents working in sequence to accomplish complex tasks. It manages task delegation, memory sharing, and coordination while maintaining efficient workflow execution.
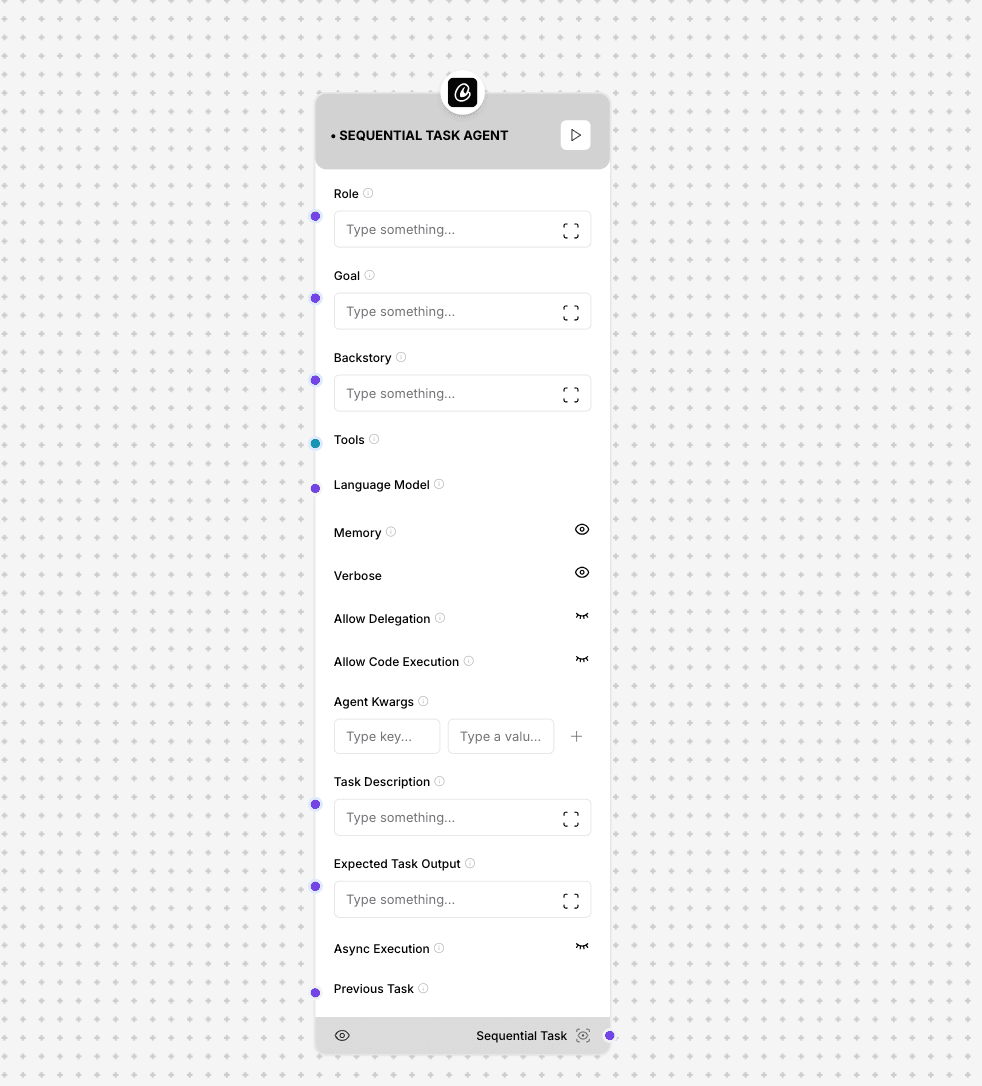
Sequential Crew workflow and architecture
Configuration Parameters
Required Input Parameters
- tasks: Array of sequential tasks
- function_calling_llm: LLM configuration
Optional Configuration
- verbose: Enable detailed logging
- memory: Memory configuration
- type: Memory storage type
- capacity: Maximum memory size
- persistence: Storage duration
- cache: Cache settings
- enabled: Enable caching
- ttl: Cache duration
- max_size: Maximum cache size
- max_rpm: Rate limiting
- share_crew: Enable crew sharing
Output Format
{ "output": { "status": "success" | "error" | "partial", "execution_summary": { "total_tasks": number, "completed_tasks": number, "failed_tasks": number, "total_time": number }, "task_results": [ { "task_id": string, "agent": string, "status": string, "result": any, "execution_time": number, "memory_usage": { "before": number, "after": number, "delta": number } } ], "crew_metrics": { "efficiency": number, "coordination_score": number, "memory_utilization": number, "cache_hits": number }, "shared_context": { "variables": object, "artifacts": array, "decisions": array }, "errors": [ { "task_id": string, "error_type": string, "message": string, "recovery_attempts": number } ], "recommendations": { "optimization": array, "scaling": array, "memory_management": array } } }
Features
- Sequential task execution
- Memory management
- Cache optimization
- Rate limiting
- Crew sharing
- Error handling
- Performance monitoring
- Context management
Note: Monitor memory usage when handling complex task sequences. Enable caching for repetitive operations.
Tip: Use verbose mode during development. Configure appropriate rate limits based on resource availability.
Example Usage
const sequentialCrew = new SequentialCrew({ tasks: [ { id: "research", agent: "ResearchAgent", input: "Gather market data" }, { id: "analysis", agent: "AnalysisAgent", input: "Analyze market trends" }, { id: "report", agent: "ReportAgent", input: "Generate summary report" } ], function_calling_llm: { model: "gpt-4", temperature: 0.3 }, verbose: true, memory: { type: "buffer", capacity: "2GB", persistence: "session" }, cache: { enabled: true, ttl: 3600, max_size: "1GB" }, max_rpm: 60, share_crew: true }); const result = await sequentialCrew.execute();