Amazon Bedrock Component
Drag & Drop LLM Component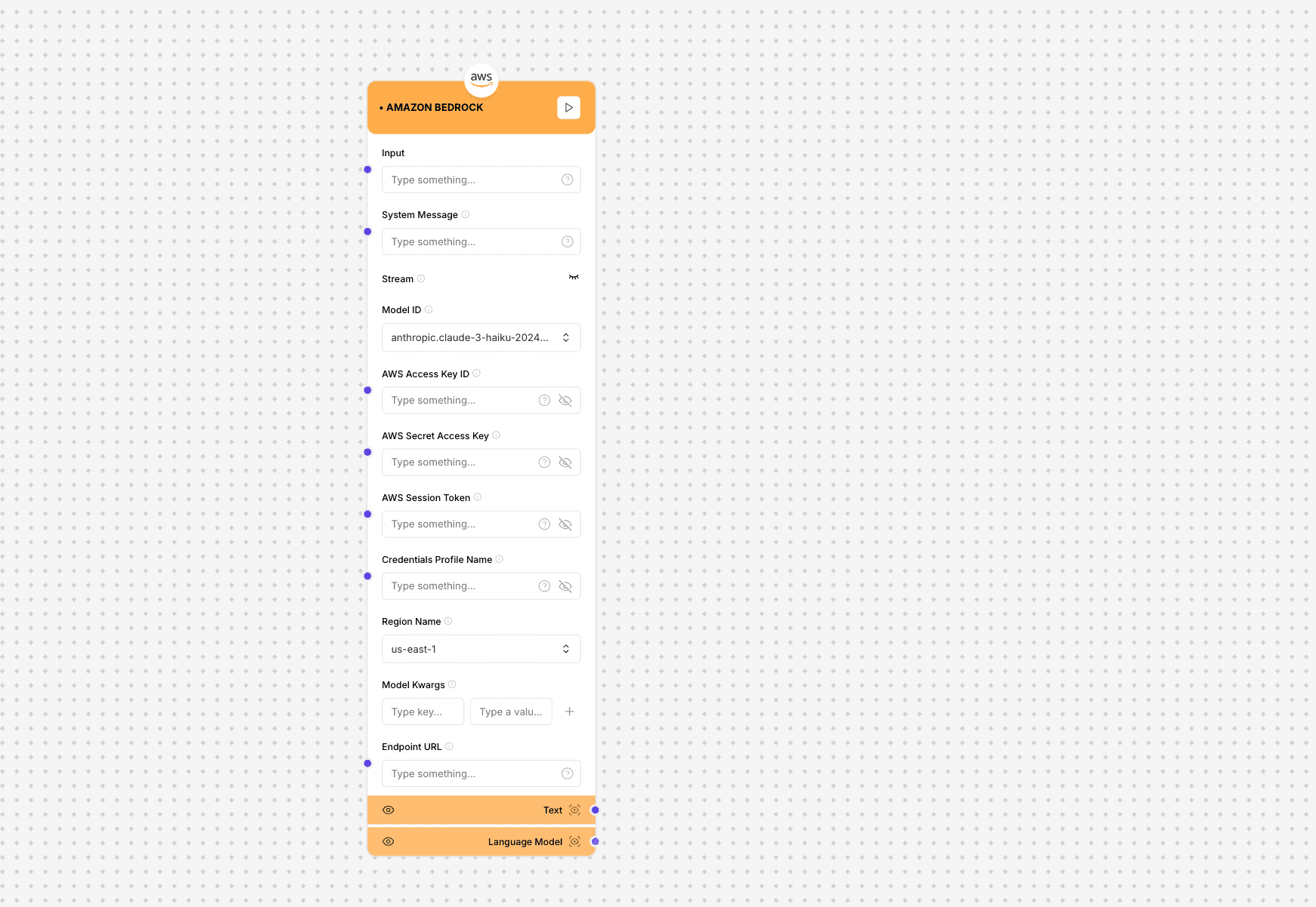
Overview
A drag-and-drop component for integrating Amazon Bedrock LLMs into your workflow. Simply configure the required parameters and connect the inputs/outputs to other components.
Component Configuration
Input Parameters
Input
Text input for the modelSystem Message
System prompt to guide model behaviorStream
Toggle for streaming responses (true/false)Model ID
e.g., anthropic.claude-3-haiku-20240307
AWS Configuration
AWS Access Key ID
Your AWS access keyAWS Secret Access Key
Your AWS secret keyAWS Session Token
Optional temporary session tokenCredentials Profile Name
AWS credentials profile (optional)Region Name
AWS region (e.g., us-east-1)
Additional Settings
Model Kwargs
Key-value pairs for additional model parametersEndpoint URL
Custom endpoint URL (optional)
Output Connections
Text
Generated text outputLanguage Model
Model information and metadata
Usage Tips
- Connect input nodes to data sources
- Use environment variables for AWS credentials
- Enable streaming for real-time responses
- Configure model parameters via kwargs
Best Practices
- Test with small inputs first
- Monitor token usage and costs
- Use appropriate regional endpoints
- Implement error handling in workflows