ArXiv
A component that searches and retrieves academic papers from arXiv, the open-access repository of scientific research. Search by topic, author, category, or keywords to integrate scholarly content into your workflows.
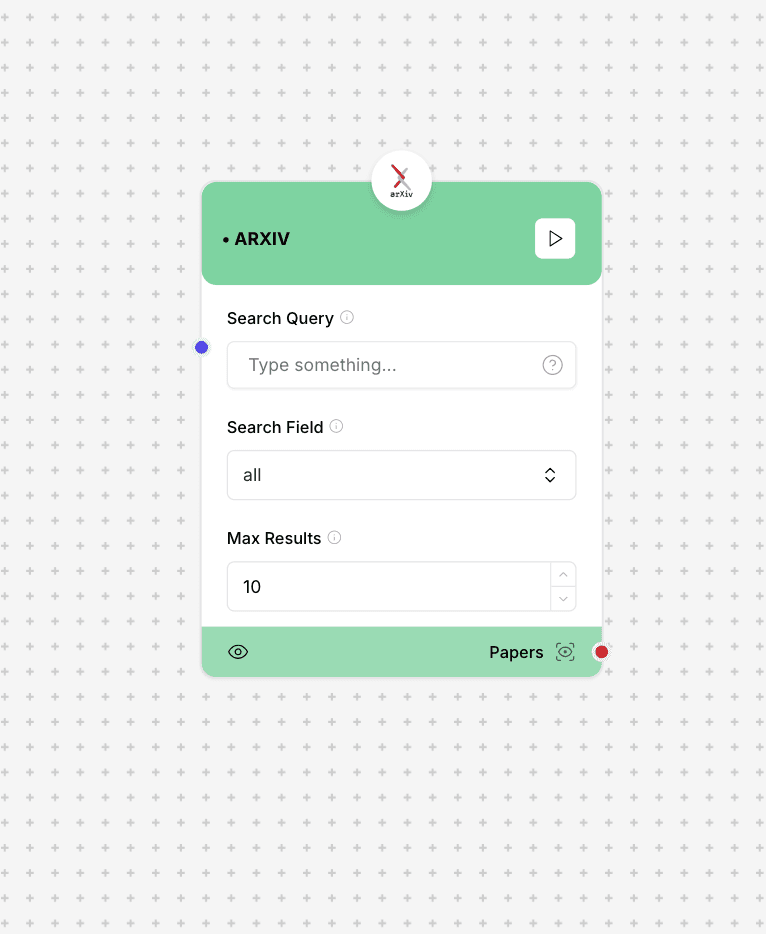
ArXiv component interface and configuration
API Rate Limits: The arXiv API has rate limitations. Make sure to space out your requests and avoid excessive querying. Use specific search terms to minimize unnecessary API calls.
Component Inputs
- Search Field: Which field to search in
Example: "all" (all fields), "title", "author", "abstract", "category"
- Max Results: Maximum number of papers to retrieve
Example: 10 (returns up to 10 research papers)
- Toolset Configuration: Additional search parameters
Example: sortBy: 'relevance', sortOrder: 'descending', includeAbstracts: true
- Search Query: The search terms to look for
Example: "transformers natural language processing", "quantum computing algorithms"
Component Outputs
- Toolset: List of research papers matching the search criteria
Example: Array of paper objects containing title, authors, abstract, publication date, and link to the full paper
Search Parameters
Search Syntax
Special operators for refined searches
Boolean operators: AND, OR, NOT (all caps)
Phrase search: Use quotes "quantum computing"
Field-specific search: prefix:term (e.g., author:"John Smith")
Sort Options
Ways to order search results
Relevance: Orders by match to search terms
SubmittedDate: Orders by submission date
LastUpdatedDate: Orders by last update date
Category Filtering
Limit search to specific disciplines
Examples:
cs: Computer Science
math: Mathematics
physics: Physics
q-bio: Quantitative Biology
q-fin: Quantitative Finance
stat: Statistics
Implementation Example
// Example: Retrieving recent papers on a specific topic
async function getRecentResearch(topic, category = null, maxResults = 10) {
// Build search query with optional category filter
let searchQuery = topic;
if (category) {
searchQuery = `cat:${category} AND ${topic}`;
}
// Execute the ArXiv search
const searchResults = await arxivComponent.execute({
searchField: "all",
maxResults: maxResults,
searchQuery: searchQuery,
toolsetConfiguration: {
sortBy: "submittedDate",
sortOrder: "descending",
includeAbstracts: true
}
});
// Process the results
const papers = searchResults.toolset.map(paper => ({
title: paper.title,
authors: paper.authors,
abstract: paper.abstract,
url: paper.pdf_url,
published: paper.published,
categories: paper.categories
}));
// Return formatted research summary
return {
topic: topic,
category: category || "all",
paperCount: papers.length,
papers: papers,
queryDate: new Date().toISOString()
};
}
// Example: Finding papers by a specific author
async function getAuthorPublications(authorName, maxResults = 25) {
const authorResults = await arxivComponent.execute({
searchField: "author",
maxResults: maxResults,
searchQuery: authorName,
toolsetConfiguration: {
sortBy: "submittedDate",
sortOrder: "descending"
}
});
return {
author: authorName,
publicationCount: authorResults.toolset.length,
publications: authorResults.toolset
};
}
Use Cases
- Literature Reviews: Gather papers on specific topics for systematic reviews
- Research Assistants: Create AI assistants that can reference academic literature
- Academic Writing: Find relevant citations for scholarly work
- Trend Monitoring: Track emerging research in specific fields
- Educational Content: Create course materials with up-to-date research
- Research Summaries: Generate summaries of recent developments in scientific fields
Useful Resources
Best Practices
- Use specific search terms to get more relevant results
- Include categories when you know the field you're interested in
- Limit the number of results when you only need recent or most relevant papers
- Implement caching to avoid repeated API calls for the same query
- Sort by relevance for targeted searches, or by date for recent developments
- Use boolean operators (AND, OR, NOT) to refine complex searches
- Consider combining the ArXiv component with text analysis tools to process paper abstracts
- Respect intellectual property by properly attributing all sources