Tool Calling Agent
The Tool Calling Agent provides a flexible framework for executing multiple tools in sequence or parallel. It manages tool orchestration, memory management, error handling, and maintains conversation context while providing detailed execution tracking.
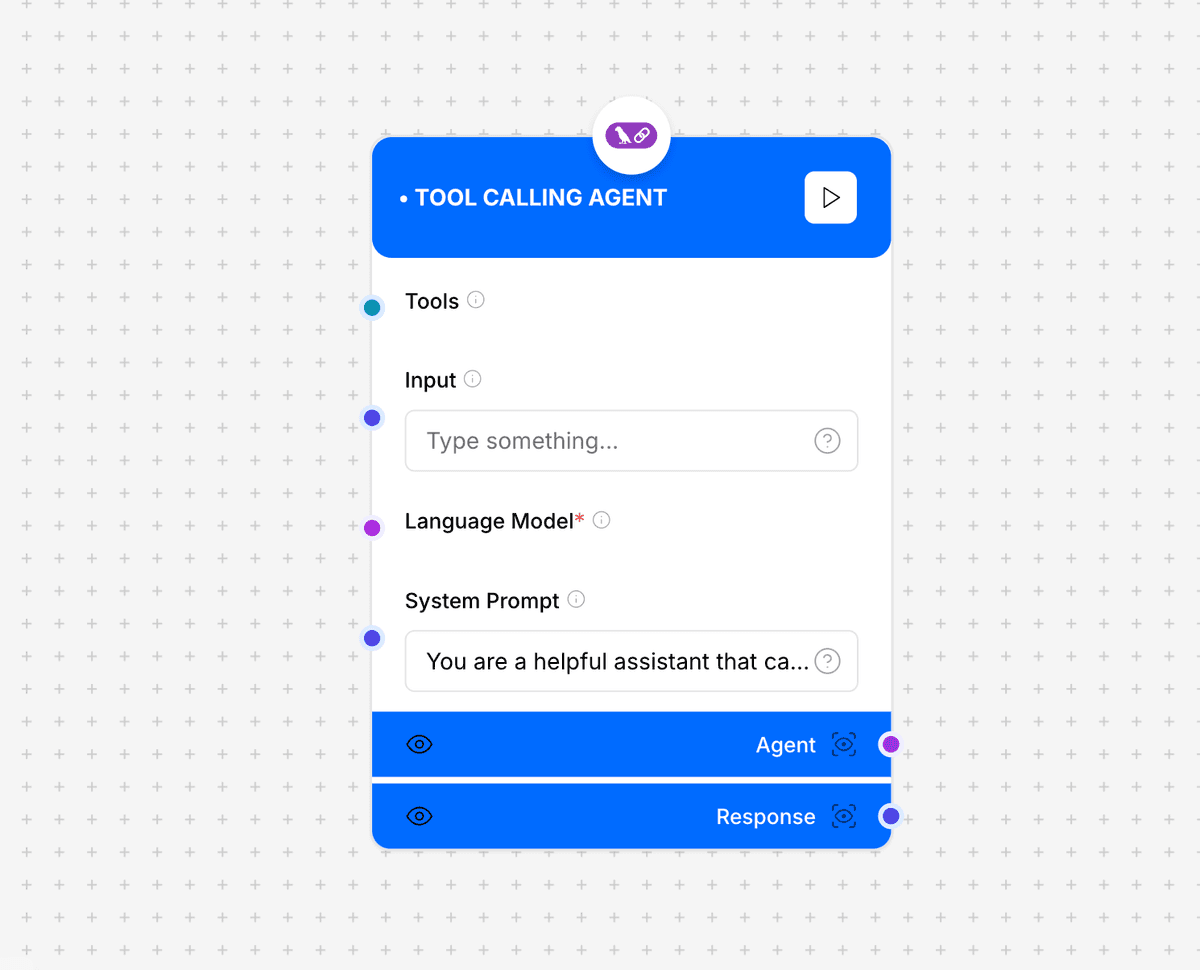
Tool Calling Agent workflow and architecture
Configuration Parameters
Required Input Parameters
- tools: Array of available tools
- input: User query or instruction
- system_prompt: Base system instructions
Optional Configuration
- llm: Language model configuration
- model_name: Model identifier
- temperature: Response creativity (0-1)
- max_tokens: Maximum response length
- agent_config: Agent behavior settings
- agent_description: Role and capabilities
- handle_parse_errors: Error handling strategy
- verbose: Detailed logging
- max_iterations: Maximum tool call iterations
- chat_memory: Memory configuration
- type: Memory storage type
- max_tokens: Token limit for history
- persistence: Storage duration
Output Format
{ "agent": { "status": "success" | "error", "execution_path": [ { "tool": string, "timestamp": string, "success": boolean, "iteration": number } ], "memory_usage": { "current_tokens": number, "max_tokens": number }, "performance_metrics": { "total_time": number, "tool_execution_time": number, "thinking_time": number } }, "response": { "message": string, "tool_results": [ { "tool_name": string, "input": object, "output": any, "error": string | null } ], "reasoning_steps": [ { "thought": string, "action": string, "result": string } ], "confidence": number } }
Features
- Multi-tool orchestration
- Sequential and parallel execution
- Memory management
- Error handling and recovery
- Execution tracking
- Performance monitoring
- Detailed logging
- Context preservation
Note: Monitor memory usage when handling long conversations. Implement appropriate cleanup strategies for completed tool executions.
Tip: Use descriptive tool names and clear parameter definitions. Enable verbose mode during development for better debugging.
Example Usage
const toolAgent = new ToolCallingAgent({ tools: [ { name: "search", description: "Search for information", parameters: { query: "string", max_results: "number" } }, { name: "summarize", description: "Summarize text content", parameters: { text: "string", max_length: "number" } } ], llm: { model_name: "gpt-4", temperature: 0.3 }, system_prompt: "You are a helpful assistant that can use various tools...", agent_config: { agent_description: "Tool orchestration specialist", handle_parse_errors: true, verbose: true, max_iterations: 5 }, chat_memory: { type: "buffer", max_tokens: 2000, persistence: "session" } }); const response = await toolAgent.execute({ input: "Search for recent AI developments and provide a summary" });