Parse DataFrame
The Parse DataFrame component converts text data into a structured DataFrame format, with support for custom templates and separators.
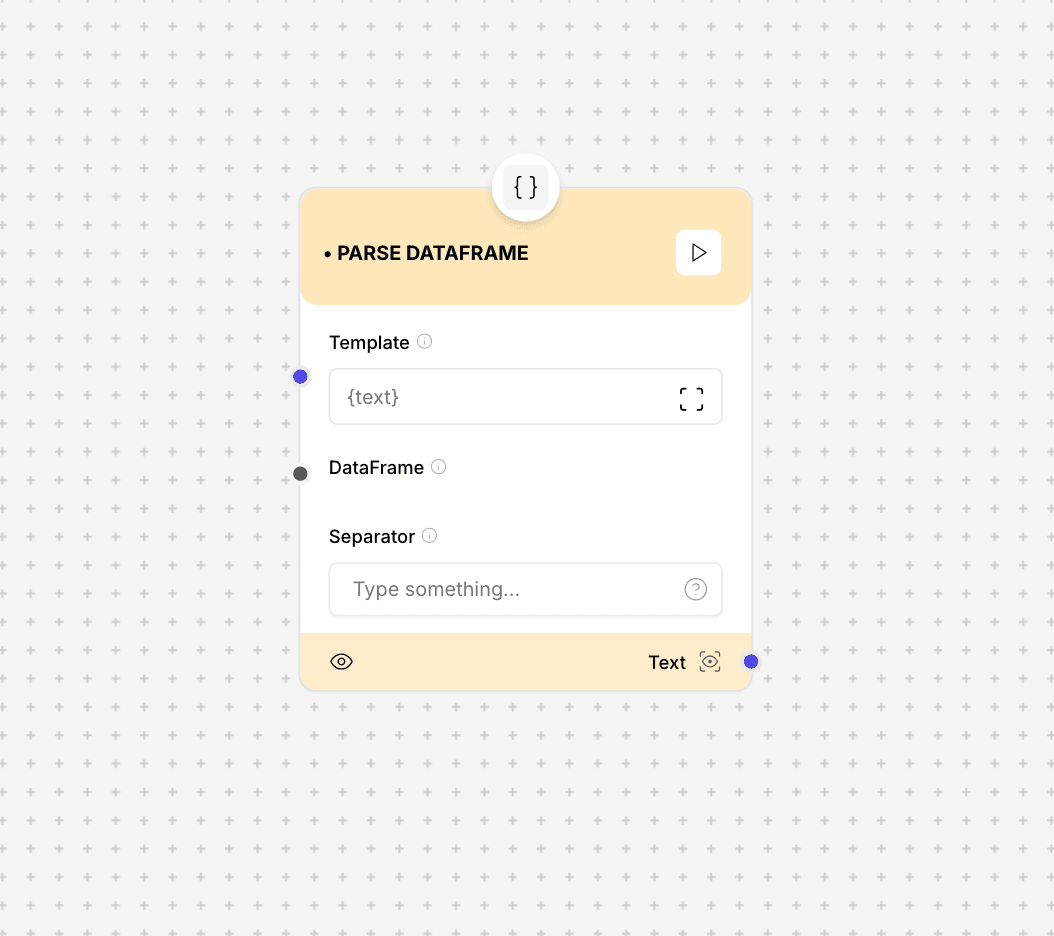
Parse DataFrame interface and configuration
Component Inputs
- Template: {text}
The template format for parsing
- DataFrame: Input data to parse
The data to be converted to DataFrame
- Separator: Custom separator
Character(s) used to separate fields
Component Outputs
- Text: Parsed text output
The resulting text after parsing
Implementation Example
const parseDataFrame = {
template: "{text}",
dataFrame: [
{ text: "Sample text 1" },
{ text: "Sample text 2" }
],
separator: ","
};
// Output:
// {
// text: "Sample text 1, Sample text 2"
// }
Additional Resources
Best Practices
- Use clear and consistent template formats
- Choose appropriate separators for your data
- Validate input data before parsing
- Handle missing or malformed data gracefully