Gibberish Detection Agent
The Gibberish Detection Agent identifies and filters nonsensical, randomly generated, or intentionally obfuscated text. It helps maintain content quality, prevent spam, and block adversarial attacks that use meaningless text to confuse or overwhelm AI systems.
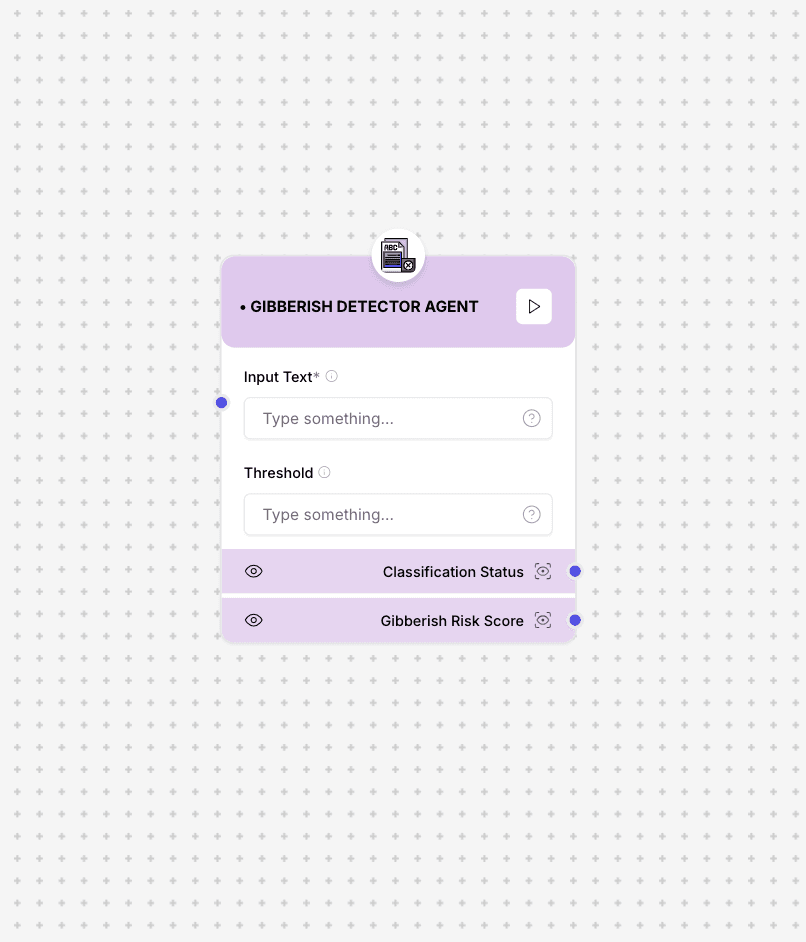
Gibberish Detection Agent interface and configuration
Implementation Notice: Gibberish detection may occasionally flag specialized technical language, code snippets, or uncommon but valid terms as potential gibberish. Consider your content domain when configuring sensitivity thresholds.
Component Inputs
- Input Text: The text content to be analyzed for gibberish or nonsensical content
Example: "Hfueid jdueid kfueis dkeis doeis fjdisf"
- Language: Primary language for gibberish evaluation
Example: "en" (English), "es" (Spanish), "auto" (Automatic detection)
- Threshold: Sensitivity level for gibberish detection
Range: 0.0 to 1.0 (default: 0.7)
Lower values increase detection sensitivity but may generate more false positives
- Is Blocked: Whether content detected as gibberish should be blocked
Options: true (block content) or false (allow but flag content)
Component Outputs
- Safety Status: Overall assessment of gibberish detection results
Values: Safe (meaningful content), Unsafe (gibberish detected)
- Risk Score: Numerical evaluation of gibberish likelihood
Scale: 0.0 (meaningful content) to 1.0 (complete gibberish)
- Coherence Score: Measure of text coherence and meaningfulness
Scale: 0.0 (incoherent) to 1.0 (highly coherent)
- Gibberish Segments: Specific portions of text identified as gibberish
Includes position information and confidence levels
Detection Categories
Types of Gibberish
- Random Character Strings: Completely random sequences of characters
Example: "asdfjkl qwerty zxcvbn"
- Keyboard Mashing: Text generated by random keyboard input
Example: "asdf jkl; qwer tyui"
- Word Salad: Real words arranged in meaningless combinations
Example: "Green ideas sleep furiously colorless"
- Character Repetition: Excessive repetition of characters or patterns
Example: "aaaaaaaa bbbbbbbb cccccccc"
- Obfuscated Text: Intentionally scrambled or encoded text
Example: "Th1s 1s d3l1b3r4t3ly 0bfu5c4t3d"
How It Works
The Gibberish Detection Agent employs multiple linguistic analysis techniques to identify text that lacks meaningful semantic structure. It evaluates character patterns, transition probabilities, lexical validity, and overall coherence to distinguish between legitimate content and nonsensical text.
Detection Techniques
- Markov chain analysis of character transition probabilities
- Dictionary-based validation of word legitimacy
- Statistical analysis of character frequency distributions
- Entropy measurement to detect randomness
- N-gram analysis for sequence probability
- Semantic coherence evaluation using language models
Use Cases
- Spam Prevention: Block nonsensical content in comments, forums, and user-generated content
- AI Protection: Prevent jailbreaking attempts using gibberish to confuse AI systems
- Quality Assurance: Ensure meaningful content in automated content generation
- User Experience: Filter out accidental or intentional keyboard mashing
- Content Moderation: Identify content that attempts to bypass filters through obfuscation
Implementation Example
const gibberishDetector = new GibberishDetectionAgent({
language: "en",
threshold: 0.7,
isBlocked: true,
minTextLength: 5
});
// Example 1: Meaningful text
const validText = "This is a completely normal and coherent English sentence.";
const validResult = gibberishDetector.analyze(validText);
// Output:
// {
// safetyStatus: "Safe",
// riskScore: 0.05,
// coherenceScore: 0.95,
// gibberishSegments: []
// }
// Example 2: Gibberish text
const gibberishText = "Hduei fkeis lwoek djsie kfue lskeuf jdieuf";
const gibberishResult = gibberishDetector.analyze(gibberishText);
// Output:
// {
// safetyStatus: "Unsafe",
// riskScore: 0.92,
// coherenceScore: 0.08,
// gibberishSegments: [
// {
// segment: "Hduei fkeis lwoek djsie kfue lskeuf jdieuf",
// position: 0,
// confidence: 0.92
// }
// ]
// }
Best Practices
- Set appropriate thresholds based on your application's specific needs
- Consider language-specific configurations for multilingual applications
- Combine with other content filters for comprehensive protection
- Implement feedback loops to improve detection accuracy over time
- Consider context-specific exceptions for domains with specialized terminology