DataFrame Operations
The DataFrame Operations component provides powerful data manipulation capabilities for structured data, supporting various operations like filtering, aggregation, and transformation.
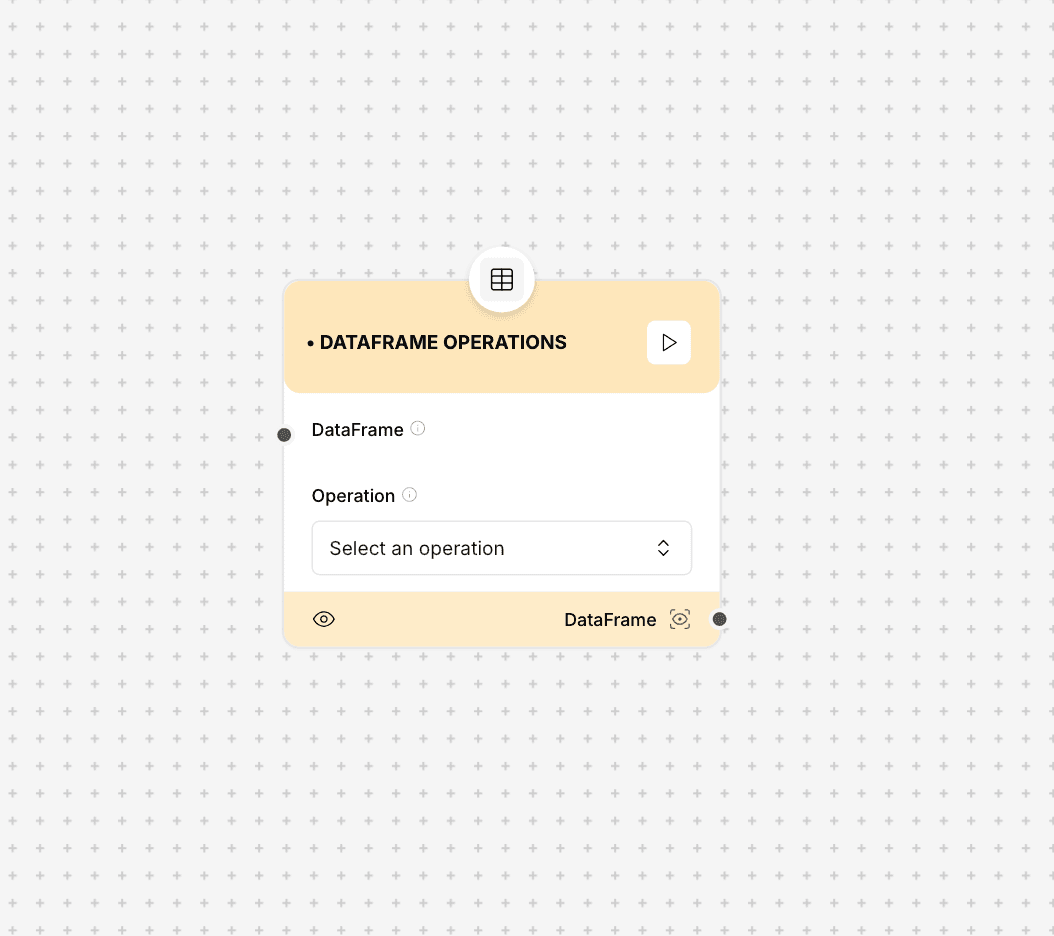
DataFrame Operations interface and configuration
Component Inputs
- DataFrame: Input data structure
The DataFrame to perform operations on
- Operation: Processing operation
The type of operation to perform on the DataFrame
Component Outputs
- DataFrame: Processed result
The resulting DataFrame after applying the operation
Implementation Example
const dataFrameOps = {
dataFrame: {
columns: ["id", "name", "age", "salary"],
data: [
[1, "John", 30, 50000],
[2, "Jane", 25, 60000],
[3, "Bob", 35, 75000]
]
},
operation: {
type: "groupBy",
columns: ["age"],
aggregations: [
{ column: "salary", function: "mean" }
]
}
};
// Output:
// {
// dataFrame: {
// columns: ["age", "mean_salary"],
// data: [
// [25, 60000],
// [30, 50000],
// [35, 75000]
// ]
// }
// }
Additional Resources
Best Practices
- Optimize operations for large datasets
- Use appropriate data types for columns
- Handle missing data appropriately
- Chain operations efficiently