Combine Text
The Combine Text component merges multiple text inputs into a single output, with support for custom delimiters and text formatting.
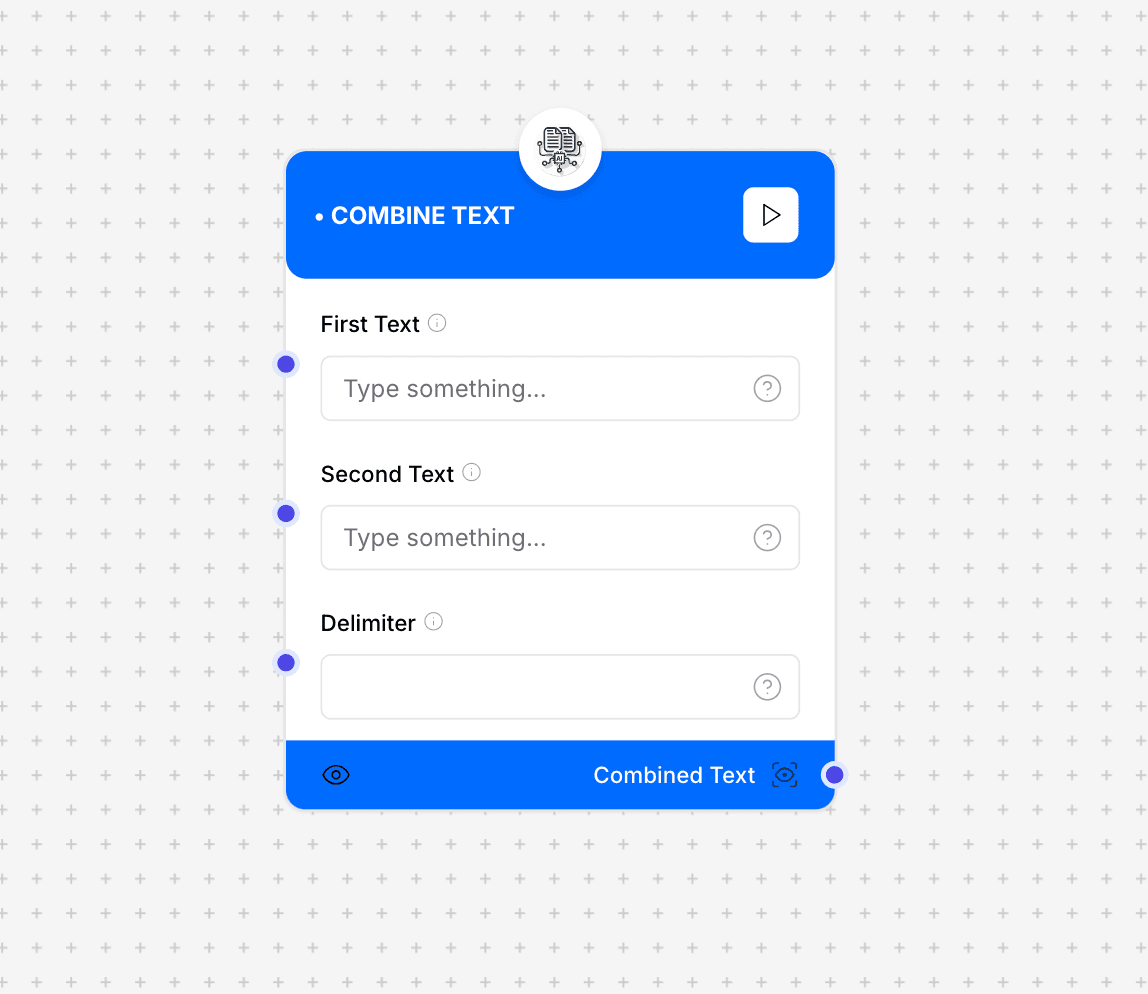
Combine Text interface and configuration
Component Inputs
- First Text: Initial text input
The first text to combine
- Second Text: Additional text input
The second text to combine
- Delimiter: Text separator
The character(s) to use between texts
Component Output
- Combined Text: Merged output
The resulting combined text
Implementation Example
const combineText = {
firstText: "Hello",
secondText: "World",
delimiter: " "
};
// Output:
// {
// combinedText: "Hello World"
// }
Additional Resources
Best Practices
- Choose appropriate delimiters for your content
- Handle empty or null inputs gracefully
- Consider text encoding compatibility
- Validate combined output length