CrewAI Agent
The CrewAI Agent is a specialized component that enables collaborative task execution through multiple AI agents working together as a coordinated team.
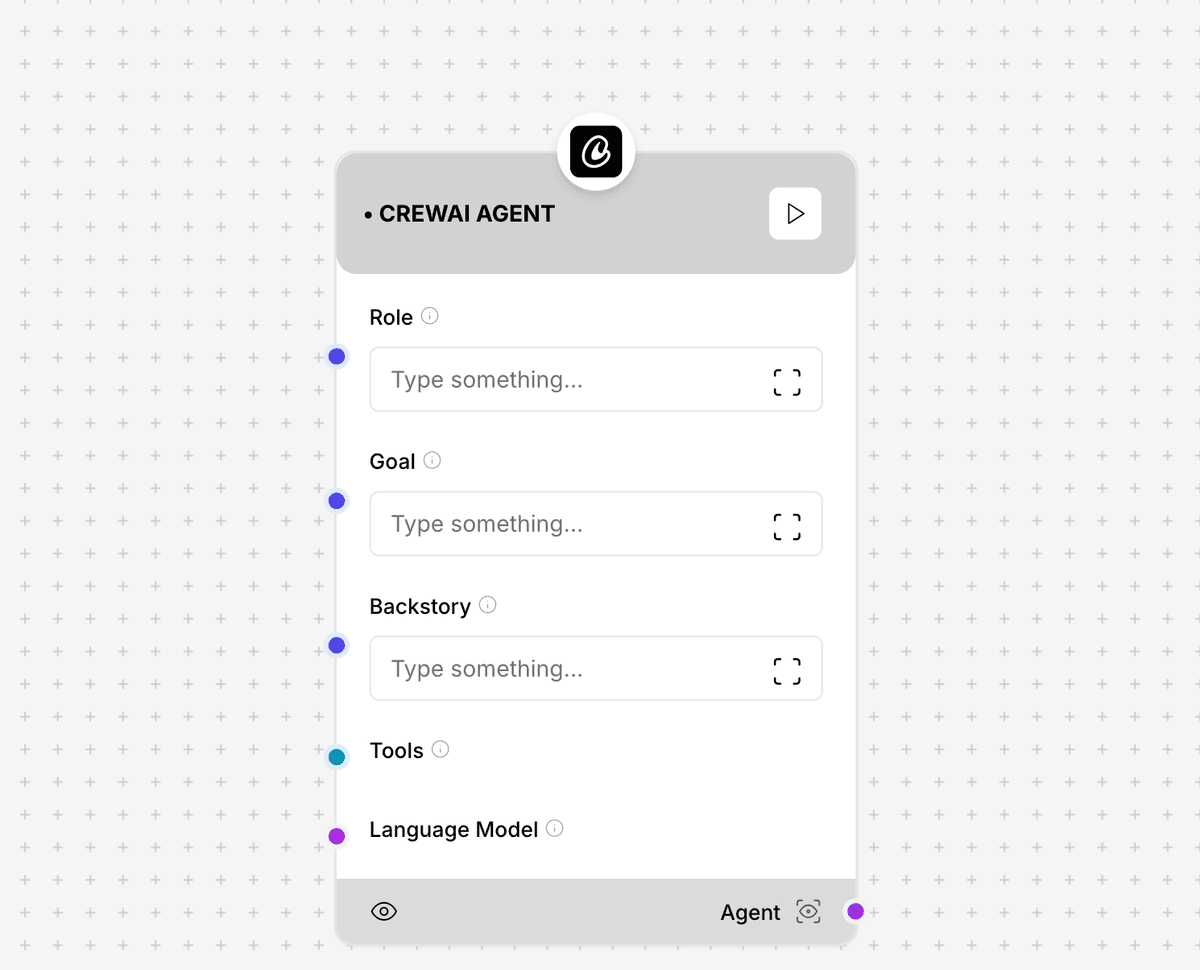
CrewAI Agent interface and configuration options
Configuration Parameters
Required Parameters
- Agents: Team member configurations
- Tasks: Task definitions and assignments
- Language Model: The AI model for processing
- Process Flow: Task execution order
- Memory System: Shared knowledge base
Optional Configuration
- Tools: Available tools and capabilities
- name: Tool identifier
- description: Tool purpose
- parameters: Required inputs
- Delegation Rules: Task delegation settings
- allowDelegation: Enable delegation
- maxDelegationDepth: Maximum chain length
- delegationStrategy: Assignment strategy
- Monitoring: Performance tracking options
Output Format
{ "result": { "taskResults": [ { "taskId": string, "agentId": string, "output": any, "status": string } ], "metadata": { "completionTime": string, "agentsInvolved": array, "taskSequence": array } } }
Example Usage
const crewAIAgent = new CrewAIAgent({ agents: [ { id: "researcher", role: "Research information", expertise: ["web-search", "data-analysis"] }, { id: "writer", role: "Content creation", expertise: ["writing", "editing"] }, { id: "reviewer", role: "Quality assurance", expertise: ["fact-checking", "proofreading"] } ], tasks: [ { id: "research", assignedTo: "researcher", description: "Gather information" }, { id: "write", assignedTo: "writer", description: "Create content" }, { id: "review", assignedTo: "reviewer", description: "Review and approve" } ], languageModel: "gpt-4", processFlow: "sequential", memorySystem: "shared" }); const result = await crewAIAgent.process({ input: "Create a research article about AI trends" });
Additional Resources
Best Practices
- Define clear agent roles
- Design efficient task workflows
- Implement proper error handling
- Monitor agent interactions
- Maintain shared knowledge base