Thought Remover Component
The Thought Remover component cleanses LLM responses by removing internal thought processes, reasoning steps, and metadata while preserving the essential response content. It ensures clean, user-friendly output from AI interactions.
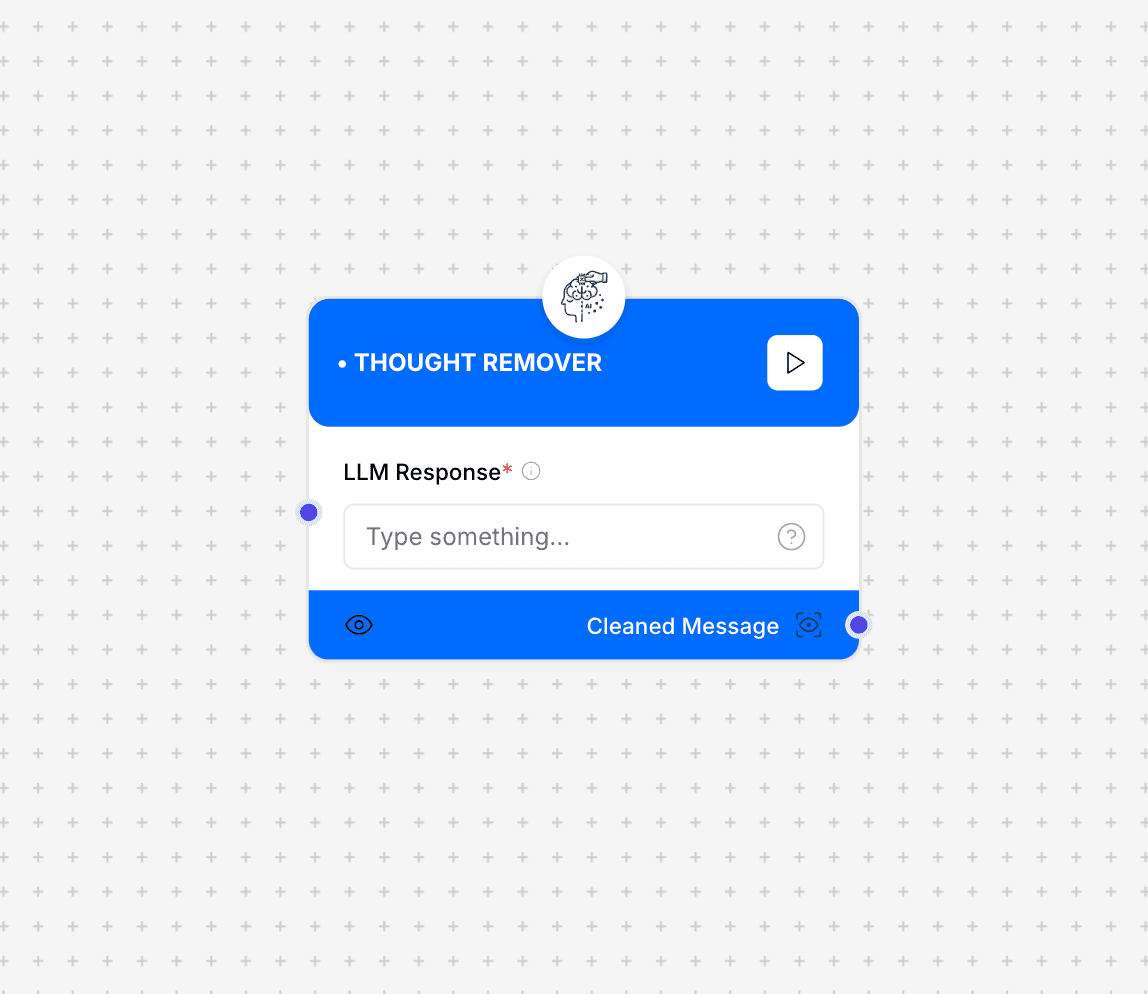
Thought Remover workflow and architecture
Configuration Parameters
Required Parameters
- llmResponse: Raw LLM response text
Output Format
{ "cleaned_message": string, "metadata": { "original_length": number, "cleaned_length": number, "removal_count": { "thought_patterns": number, "reasoning_blocks": number, "metadata_sections": number }, "processing_time": number }, "removed_content": { "thoughts": string[], "reasoning": string[], "metadata": string[] }, "statistics": { "reduction_percentage": number, "pattern_matches": number, "content_preserved": number } }
Features
- Pattern-based removal
- Content preservation
- Metadata extraction
- Performance metrics
- Format validation
- Error handling
- Content statistics
- Removal tracking
Note: The component identifies and removes common thought patterns like "Let me think...", "Analyzing...", and step-by-step reasoning markers.
Tip: Monitor the reduction percentage to ensure important content is not being removed. Adjust patterns if needed.
Example Usage
const thoughtRemover = new ThoughtRemover(); // Example with thought process const result1 = await thoughtRemover.clean({ llmResponse: ` Let me think about this... First, I'll analyze the requirements. 1. User needs a summary 2. Content should be concise 3. Format must be clear Based on this analysis, here's the summary: The project deadline is next week and requires three main deliverables. ` }); // Example with metadata const result2 = await thoughtRemover.clean({ llmResponse: ` [PROCESSING] Analyzing input... [CONFIDENCE: 0.95] The weather forecast shows sunny conditions with a high of 75°F tomorrow. [SOURCES: weather-api, local-data] ` }); // Example with reasoning steps const result3 = await thoughtRemover.clean({ llmResponse: ` Step 1: Examine the code Found potential memory leak Step 2: Analyze impact Critical for long-running processes Step 3: Propose solution Implement proper cleanup in destructor Recommendation: Add destructor method to handle resource cleanup properly. ` });
Common Patterns Removed:
// Thought indicators "Let me think..." "Analyzing..." "Processing..." // Step markers "Step 1:", "First:", etc. "Next, I'll..." "Finally..." // Metadata blocks "[CONFIDENCE: 0.XX]" "[PROCESSING]" "[SOURCES: ...]" // Reasoning patterns "Based on this..." "This suggests..." "Therefore..."