Machine Learning Agent
The Machine Learning Agent automates ML workflows, from data preprocessing to model deployment. It handles model selection, hyperparameter tuning, training monitoring, and provides intelligent feedback while maintaining conversation context and external memory.
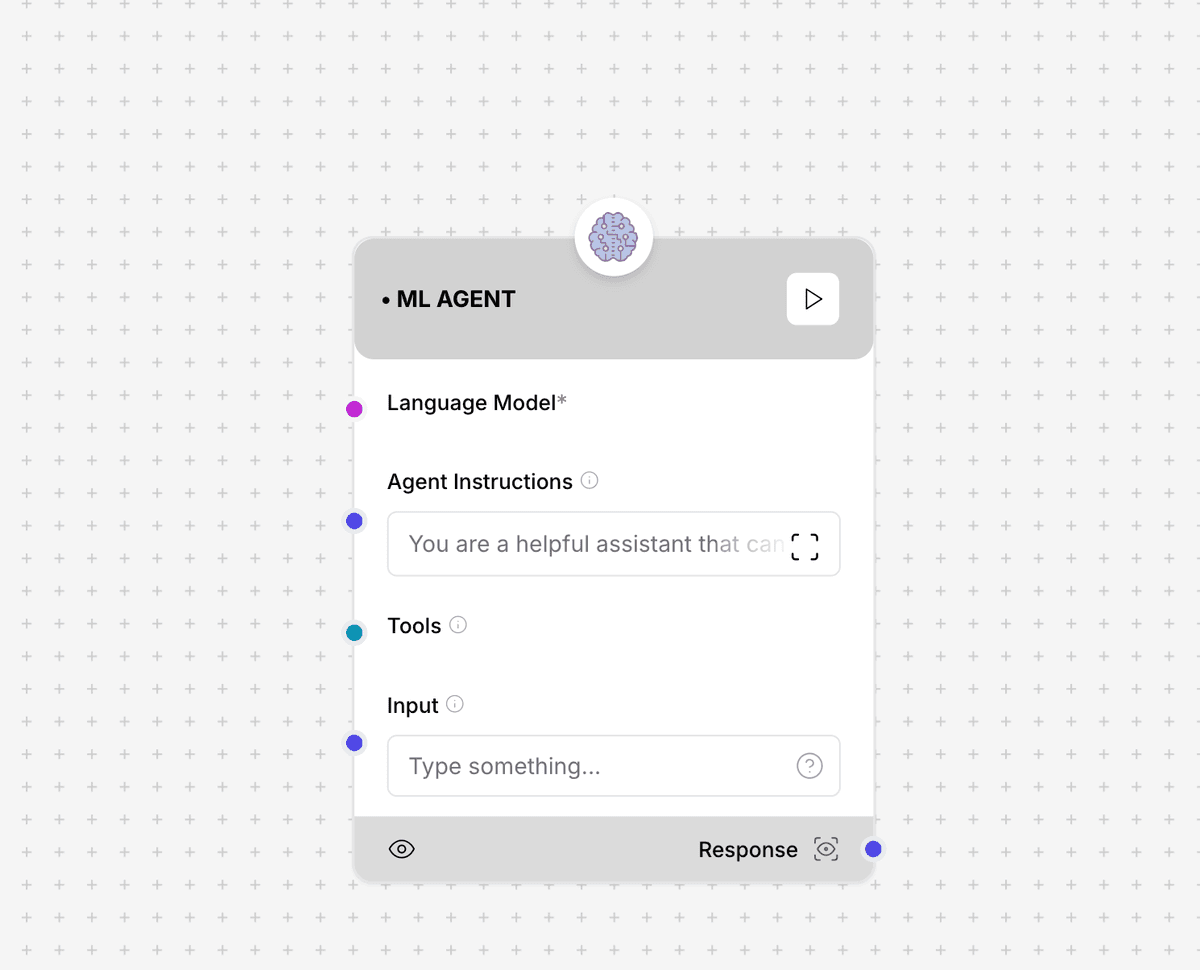
Machine Learning Agent workflow and architecture
Configuration Parameters
Required Input Parameters
- template: ML workflow template
- input: User query or task description
- session_id: Unique session identifier
Optional Configuration
- llm: Language model configuration
- model_name: Name of the language model
- temperature: Response creativity (0-1)
- max_tokens: Maximum response length
- tools: Available ML tools and libraries
- sklearn: Scikit-learn tools
- tensorflow: TensorFlow operations
- pytorch: PyTorch functions
- custom_tools: User-defined tools
- external_memory: Memory configuration
- type: Memory storage type
- capacity: Maximum memory size
- persistence: Storage duration
- sender_config: Message handling
- sender_type: Type of sender
- sender_name: Name identifier
- number_of_messages: Message limit
- order: Message processing order
- agent_config: Agent behavior
- agent_description: Role description
- agent_instructions: Behavior guidelines
- handle_parse_errors: Error handling strategy
- verbose: Detailed logging
- max_iterations: Maximum processing iterations
Output Format
{ "response": { "result": { "model_output": any, "metrics": object, "artifacts": array }, "explanation": { "steps": array, "decisions": array, "visualizations": array }, "recommendations": { "next_steps": array, "improvements": array } }, "metadata": { "session_info": { "session_id": string, "timestamp": string, "duration": number }, "memory_usage": { "current_size": number, "peak_size": number }, "processing_stats": { "iterations": number, "errors_handled": number } } }
Features
- Automated ML workflow execution
- Intelligent model selection
- Hyperparameter optimization
- Training monitoring
- Error handling and recovery
- Performance tracking
- Memory management
- Conversation context preservation
Note: Monitor memory usage when processing large datasets. Consider implementing checkpointing for long-running operations.
Tip: Use templates for common ML workflows and enable verbose mode during development for better debugging capabilities.
Example Usage
const mlAgent = new MLAgent({ template: "classification_workflow", llm: { model_name: "gpt-4", temperature: 0.3 }, tools: ["sklearn", "pytorch"], external_memory: { type: "redis", capacity: "10GB" }, sender_config: { sender_type: "user", sender_name: "data_scientist", number_of_messages: 100, order: "sequential" }, agent_config: { agent_description: "ML workflow automation specialist", handle_parse_errors: true, verbose: true, max_iterations: 1000 } }); const response = await mlAgent.execute({ input: "Train a classification model on customer churn data", session_id: "session_123" });